JavaScript array.pop() Method
-
Syntax of JavaScript
array.pop()
Method -
Example 1: Use
array.pop()
to Remove the Last Element From the Array -
Example 2: Use
array.pop()
to Get the Last Element Removed From the Array -
Example 3: Use the
array.pop()
Method With an Empty Array -
Example 4: Use the
array.pop()
Method to Remove Last Element From Array-Like Objects
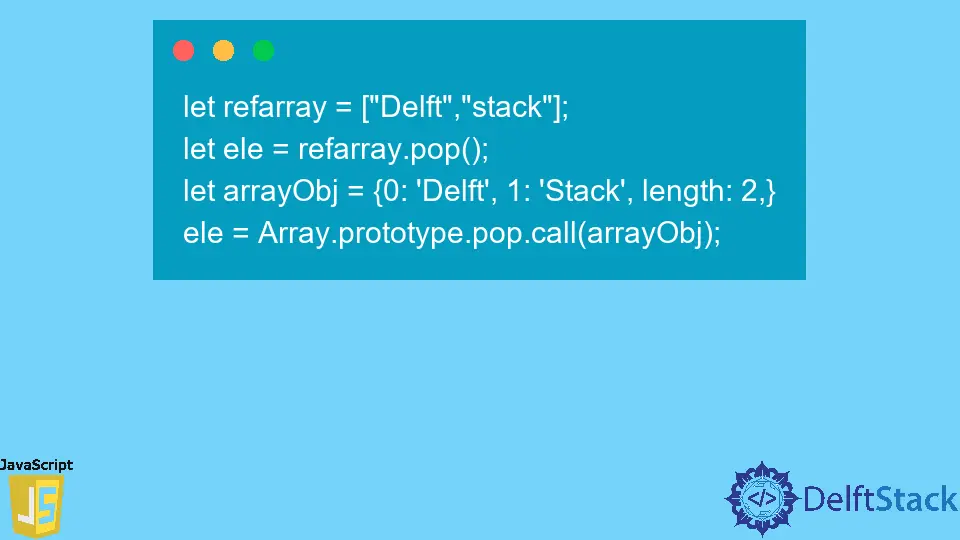
In JavaScript, the array.pop()
method eliminates the last element of an array. This method can also be used to remove elements.
Syntax of JavaScript array.pop()
Method
let refarray = ["Delft","stack"];
let ele = refarray.pop();
let arrayObj = {0: 'Delft', 1: 'Stack', length: 2,}
ele = Array.prototype.pop.call(arrayObj);
Parameters
arrayObj |
an array-like object from which we need to remove the last element. |
Return
This method returns the removed element from the given refarray
.
Example 1: Use array.pop()
to Remove the Last Element From the Array
In JavaScript, the array.pop()
method removes the last element from an array. These elements can be strings, numbers, or any type allowed to create an array in JavaScript.
In this example, an array has been created with three elements. We removed the last element in the output using the array.pop()
method.
const arrtype = ["String","Number","Array"];
arrtype.pop();
console.log(arrtype);
Output:
[ 'String', 'Number' ]
Example 2: Use array.pop()
to Get the Last Element Removed From the Array
When we call out the array.pop()
method, it will remove the last element from the given array and return it the same as the output. In this example, we have created an array using numbers.
Then, we used a variable name for the array.pop()
method. When we called out the variable, the method returned the element that got removed from the array in the output.
const arrnum = [[5,0,][4,25],[20,34]];
let removeEl = arrnum.pop();
console.log(removeEl);
Output:
[ 20, 34 ]
Example 3: Use the array.pop()
Method With an Empty Array
In the example below, we have used the empty array with the array.pop()
method. When an array is empty, the method returns the undefined
value without giving any single error that users can see in the output.
const arr = [];
let removeEl = arr.pop();
console.log(removeEl);
Output:
undefined
Example 4: Use the array.pop()
Method to Remove Last Element From Array-Like Objects
An array-like object means an object which contains the key-value pairs and length of the object also.
In this example, we have defined the array-like object of length 2 and invoked the array.pop()
method. After that, we printed the object and popped the element on the screen.
Users can see that the last element from the object is removed, and the object’s length is also decreased by 1.
let arrayObj = {0: 'Delft', 1: 'Stack', length: 2,}
let ele = Array.prototype.pop.call(arrayObj);
console.log(ele);
console.log(arrayObj);
Output:
Stack
{ '0': 'Delft', length: 1 }
The array.pop()
method removes and returns the last element from the given array.