Matplotlib 密度图
Suraj Joshi
2024年2月15日
Matplotlib
Matplotlib Plot
-
使用
scipy.stats
模块中的gaussian_kde()
方法生成密度图 -
使用
seaborn
包中的kdeplot()
方法生成密度图 -
使用
distplot()
方法从seaborn
包中生成密度图 -
在
pandas.DataFrame.plot()
方法中设置kind='density'
来生成密度图
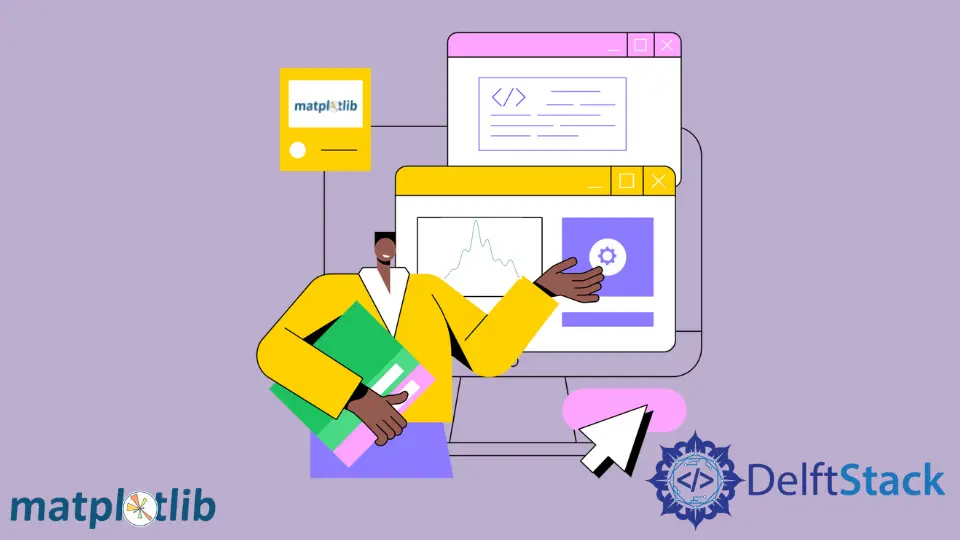
为了使用 Python 生成密度图,我们首先使用 scipy.stats
模块中的 gaussian_kde()
方法从给定数据中估计密度函数。然后我们绘制密度函数,生成密度图。另外,我们也可以使用 seaborn
包中的 kdeplot()
或者在 pandas.DataFrame.plot()
方法中设置 kind='density'
来生成密度图。
使用 scipy.stats
模块中的 gaussian_kde()
方法生成密度图
import numpy as np
import matplotlib.pyplot as plt
from scipy.stats import kde
data = [2, 3, 3, 4, 2, 1, 5, 6, 4, 3, 3, 3, 6, 4, 5, 4, 3, 2]
density = kde.gaussian_kde(data)
x = np.linspace(-2, 10, 300)
y = density(x)
plt.plot(x, y)
plt.title("Density Plot of the data")
plt.show()
输出:
在这里,我们首先使用 gaussian_kde()
方法估计给定数据的密度函数。然后,我们用 plot()
方法绘制出从 -2
到 10
的值的函数。
生成的密度图足够是不精确的,这是由 gaussian_kde
函数自动设置带宽而导致的。为了设置带宽,我们可以使用 gaussian_kde
类的 covariance_factor
函数。然后我们调用 _compute_covariance
方法,使所有的因子都能正确计算,从而生成精确的图。
import numpy as np
import matplotlib.pyplot as plt
from scipy.stats import kde
data = [2, 3, 3, 4, 2, 1, 5, 6, 4, 3, 3, 3, 6, 4, 5, 4, 3, 2]
prob_density = kde.gaussian_kde(data)
prob_density.covariance_factor = lambda: 0.25
prob_density._compute_covariance()
x = np.linspace(-2, 10, 300)
y = prob_density(x)
plt.plot(x, y)
plt.title("Density Plot of the data")
plt.show()
输出:
使用 seaborn
包中的 kdeplot()
方法生成密度图
import matplotlib.pyplot as plt
import seaborn as sns
data = [2, 3, 3, 4, 2, 1, 5, 6, 4, 3, 3, 3, 6, 4, 5, 4, 3, 2]
sns.kdeplot(data, bw=0.25)
plt.show()
输出:
这样,我们只需将数据传入 kdeplot()
方法就可以生成密度图。
使用 distplot()
方法从 seaborn
包中生成密度图
我们也可以使用 seaborn
包中的 distplot()
方法,并设置 hist=False
来生成密度图。
import matplotlib.pyplot as plt
import seaborn as sns
data = [2, 3, 3, 4, 2, 1, 5, 6, 4, 3, 3, 3, 6, 4, 5, 4, 3, 2]
sns.distplot(data, hist=False)
plt.show()
输出:
在 pandas.DataFrame.plot()
方法中设置 kind='density'
来生成密度图
import pandas as pd
import matplotlib.pyplot as plt
data = [2, 3, 3, 4, 2, 1, 5, 6, 4, 3, 3, 3, 6, 4, 5, 4, 3, 2]
df = pd.DataFrame(data)
df.plot(kind="density")
plt.show()
输出:
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Suraj Joshi
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn