C 语言中如何检查文件是否存在
Satishkumar Bharadwaj
2023年10月12日
C
C File
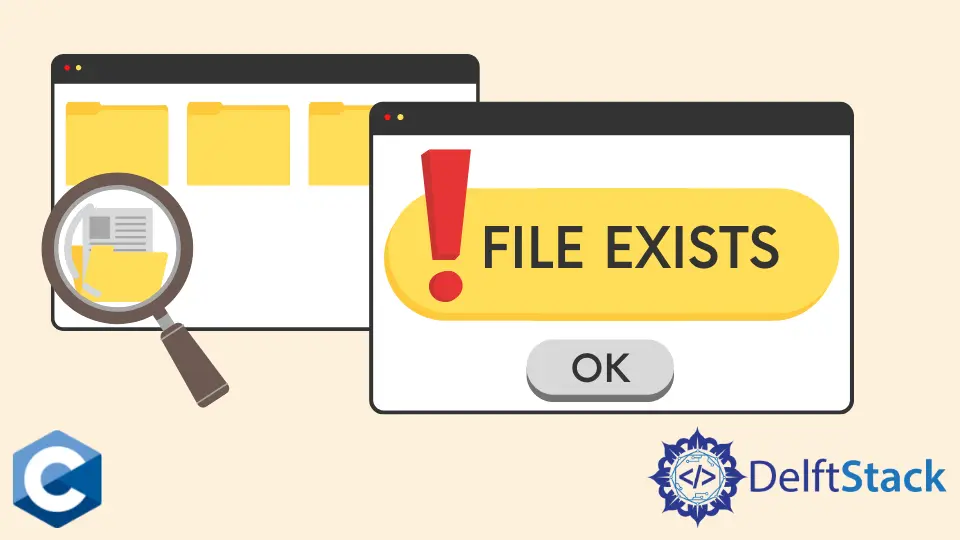
本教程介绍了用 C 语言检查文件是否存在的方法,检查文件是否存在的方法是尝试以读取或写入模式打开文件。本程序是以读取模式打开一个文件的。
fopen()
检查 C 语言中文件是否存在的函数
#include <stdio.h>
int main(void) {
FILE *file;
if (file = fopen("demo.txt", "r")) {
fclose(file);
printf("file exists");
} else {
printf("file doesn't exist");
}
return 0;
}
输出:
file exists
这里的文件名是 demo.txt
。C 程序和 demo.txt
文件在同一个目录下。所以输出的结果是 file exists
。
如果 C 程序位置和文件位置不同,我们必须指定文件的完整路径。
我们可以创建一个用户自定义函数,检查文件是否存在。下面是带有用户自定义函数的程序。
#include <stdio.h>
int checkIfFileExists(const char *filename);
int main(void) {
if (checkIfFileExists("demo.txt")) {
printf("file exists");
} else {
printf("file does not exists");
}
}
int checkIfFileExists(const char *filename) {
FILE *file;
if (file = fopen(filename, "r")) {
fclose(file);
return 1;
}
return 0;
}
如果 demo.txt
文件和 C 程序的位置相同,程序将打印 file exists
。如果 C 程序和文件名的位置不同,我们必须指定文件的完整路径。
stat()
函数检查 C 程序中的文件是否存在
我们使用 stat()
函数读取文件的属性,而不是从文件中读取数据。如果操作成功,该函数将返回 0
;否则,将返回 -1
,如果文件不存在。
#include <stdio.h>
#include <sys/stat.h>
int checkIfFileExists(const char* filename);
int main(void) {
if (checkIfFileExists("demo.txt")) {
printf("file exists");
} else {
printf("file does not exists");
}
}
int checkIfFileExists(const char* filename) {
struct stat buffer;
int exist = stat(filename, &buffer);
if (exist == 0)
return 1;
else
return 0;
}
如果 demo.txt
文件和 C 程序的位置相同,程序将打印 file exists
。如果 C 程序和文件名的位置不同,我们必须指定文件的完整路径。
access()
检查 C 程序中文件是否存在的函数
检查文件是否存在的另一种方法是使用 access
函数。unistd.h
头文件中有一个函数 access
来检查文件是否存在。
我们可以用 R_OK
表示读权限,W_OK
表示写权限,X_OK
表示执行权限。我们可以将它们组合成 R_OK|W_OK
来读取和写入权限。
#include <stdio.h>
#include <unistd.h>
int main(void) {
if (access("C:\\TEMP\\demo.txt", F_OK) != -1) {
printf("file is found");
} else {
printf("file is not found");
}
return 0;
}
输出的结果是
file is found
这里,C:TEMPdemo.txt
是文件位置。如果文件存在,则打印 file is found
;否则,打印 file is not found
。程序位置和 demo.txt
文件的位置相同。否则,我们必须指定文件的位置。对于 Linux 操作系统来说,stat()
和 access()
函数是一个不错的选择。
另一种使用 access 函数的方法如下。
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
void checkIfFileExists(const char *fileName);
int main(void) {
char *fileName = "C:\\TEMP\\demo.txt";
checkIfFileExists(fileName);
return 0;
}
void checkIfFileExists(const char *fileName) {
if (!access(fileName, F_OK)) {
printf("The File %s was Found\n", fileName);
} else {
printf("The File %s not Found\n", fileName);
}
if (!access(fileName, R_OK)) {
printf("The File %s can be read\n", fileName);
} else {
printf("The File %s cannot be read\n", fileName);
}
if (!access(fileName, W_OK)) {
printf("The File %s can be Edited\n", fileName);
} else {
printf("The File %s cannot be Edited\n", fileName);
}
if (!access(fileName, X_OK)) {
printf("The File %s is an Executable\n", fileName);
} else {
printf("The File %s is not an Executable\n", fileName);
}
}
输出:
The file C:\TEMP\demo.txt was found
The file C:\TEMP\demo.txt can be read
The file C:\TEMP\demo.txt can be Edited
The file C:\TEMP\demo.txt is an executable
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe