C에 파일이 있는지 확인
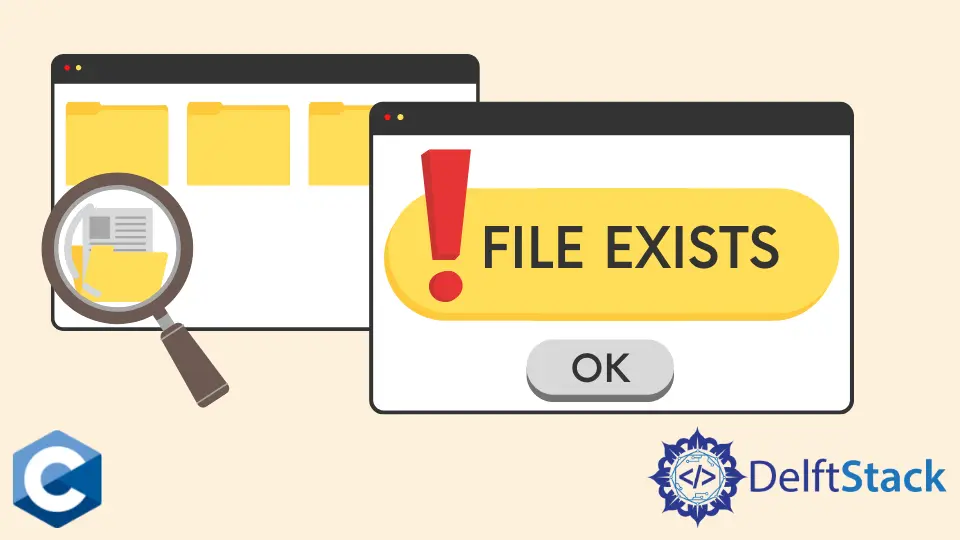
이 튜토리얼에서는 파일이 C에 있는지 확인하는 방법을 소개합니다. 파일이 있는지 확인하는 방법은 파일을 읽기 또는 쓰기 모드로 열어 보는 것입니다. 이 프로그램은 읽기 모드에서 파일을 여는 중입니다.
파일이 C에 있는지 확인하는fopen()
함수
#include <stdio.h>
int main(void) {
FILE *file;
if (file = fopen("demo.txt", "r")) {
fclose(file);
printf("file exists");
} else {
printf("file doesn't exist");
}
return 0;
}
출력은 다음과 같습니다.
file exists
여기서 파일 이름은demo.txt
입니다. C 프로그램과demo.txt
파일은 같은 디렉토리에 있습니다. 따라서 출력은 file exists
입니다.
C 프로그램 위치와 파일 위치가 다른 경우 파일의 전체 경로를 지정해야합니다.
사용자 정의 함수를 생성하고 파일의 존재 여부를 확인할 수 있습니다. 다음은 사용자 정의 기능이있는 프로그램입니다.
#include <stdio.h>
int checkIfFileExists(const char *filename);
int main(void) {
if (checkIfFileExists("demo.txt")) {
printf("file exists");
} else {
printf("file does not exists");
}
}
int checkIfFileExists(const char *filename) {
FILE *file;
if (file = fopen(filename, "r")) {
fclose(file);
return 1;
}
return 0;
}
프로그램은demo.txt
파일과 C 프로그램이 같은 위치에 있으면file exists
를 출력합니다. C 프로그램과 파일 이름이 다른 위치에있는 경우 파일의 전체 경로를 지정해야합니다.
파일이 C에 있는지 확인하는stat()
함수
파일에서 데이터를 읽는 대신stat()
함수를 사용하여 파일의 속성을 읽습니다. 이 함수는 작업이 성공하면 0
을 반환합니다. 그렇지 않으면 파일이 존재하지 않으면-1
을 반환합니다.
#include <stdio.h>
#include <sys/stat.h>
int checkIfFileExists(const char* filename);
int main(void) {
if (checkIfFileExists("demo.txt")) {
printf("file exists");
} else {
printf("file does not exists");
}
}
int checkIfFileExists(const char* filename) {
struct stat buffer;
int exist = stat(filename, &buffer);
if (exist == 0)
return 1;
else
return 0;
}
프로그램은demo.txt
파일과 C 프로그램이 같은 위치에 있으면file exists
를 출력합니다. C 프로그램과 파일 이름이 다른 위치에있는 경우 파일의 전체 경로를 지정해야합니다.
C에 파일이 있는지 확인하는access()
함수
파일이 존재하는지 확인하는 또 다른 방법은access()
함수를 사용하는 것입니다. unistd.h
헤더 파일에는 파일의 존재 여부를 확인하는 ‘액세스’기능이 있습니다.
읽기 권한에는 R_OK
, 쓰기 권한에는 W_OK
, 실행 권한에는 X_OK
를 사용할 수 있습니다. 읽기 및 쓰기 권한을 위해R_OK|W_OK
로 함께 사용할 수 있습니다.
#include <stdio.h>
#include <unistd.h>
int main(void) {
if (access("C:\\TEMP\\demo.txt", F_OK) != -1) {
printf("file is found");
} else {
printf("file is not found");
}
return 0;
}
출력은 다음과 같습니다.
file is found
여기서C:\TEMPdemo.txt
는 파일 위치입니다. 파일이 존재하면file is found
를 출력합니다. 그렇지 않으면파일을 찾을 수 없음
을 출력합니다. 프로그램 위치와demo.txt
파일은 같은 위치에 있습니다. 그렇지 않으면 파일의 위치를 지정해야합니다. stat()
및access()
함수의 조합은 Linux 운영 체제에 적합한 선택입니다.
access()
함수를 사용하는 또 다른 방법은 다음과 같습니다.
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
void checkIfFileExists(const char *fileName);
int main(void) {
char *fileName = "C:\\TEMP\\demo.txt";
checkIfFileExists(fileName);
return 0;
}
void checkIfFileExists(const char *fileName) {
if (!access(fileName, F_OK)) {
printf("The File %s was Found\n", fileName);
} else {
printf("The File %s not Found\n", fileName);
}
if (!access(fileName, R_OK)) {
printf("The File %s can be read\n", fileName);
} else {
printf("The File %s cannot be read\n", fileName);
}
if (!access(fileName, W_OK)) {
printf("The File %s can be Edited\n", fileName);
} else {
printf("The File %s cannot be Edited\n", fileName);
}
if (!access(fileName, X_OK)) {
printf("The File %s is an Executable\n", fileName);
} else {
printf("The File %s is not an Executable\n", fileName);
}
}
출력은 다음과 같습니다.
The file C :\TEMP\demo.txt was found The file C
:\TEMP\demo.txt can be read The file C
:\TEMP\demo.txt can be Edited The file C :\TEMP\demo.txt is an executable