C 言語でファイルが存在するかどうかのチェック
-
C にファイルが存在するかどうかを確認する
fopen()
関数 -
C 言語でファイルが存在するかどうかを確認する
stat()
関数 -
C 言語でファイルが存在するかどうかを調べるための
access()
関数
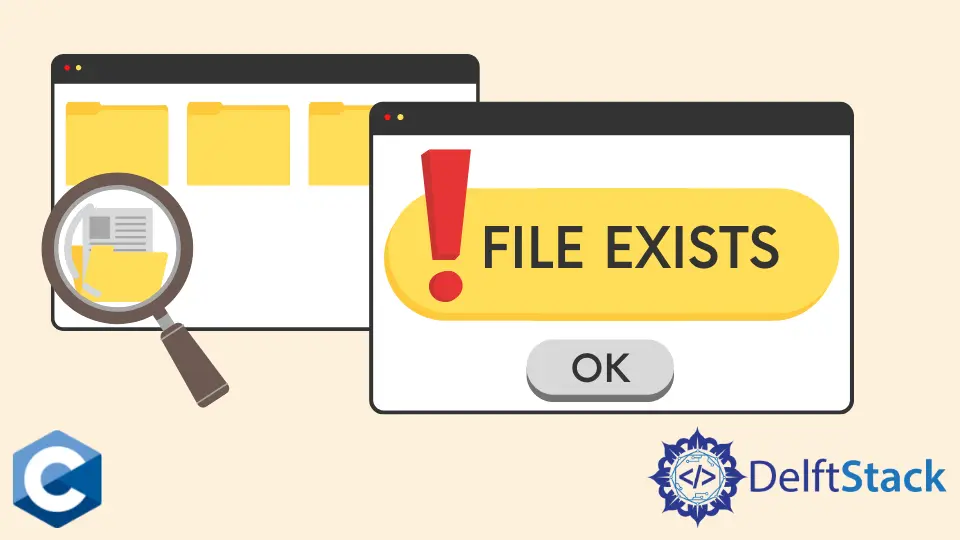
このチュートリアルでは、C 言語でファイルが存在するかどうかを確認する方法を紹介します。ファイルが存在するかどうかを確認する方法は、ファイルを読み書きモードで開いてみることです。このプログラムでは、ファイルを読み込みモードで開いてみます。
C にファイルが存在するかどうかを確認する fopen()
関数
#include <stdio.h>
int main(void) {
FILE *file;
if (file = fopen("demo.txt", "r")) {
fclose(file);
printf("file exists");
} else {
printf("file doesn't exist");
}
return 0;
}
出力は次のとおりです。
file exists
ここでのファイル名は demo.txt
です。C プログラムと demo.txt
ファイルは同じディレクトリにあります。つまり、出力は file exists
です。
C プログラムの場所とファイルの場所が異なる場合は、ファイルのフルパスを指定しなければならません。
ユーザ定義の関数を作成して、ファイルが存在するかどうかを確認できます。以下はユーザ定義関数を用いたプログラムです。
#include <stdio.h>
int checkIfFileExists(const char *filename);
int main(void) {
if (checkIfFileExists("demo.txt")) {
printf("file exists");
} else {
printf("file does not exists");
}
}
int checkIfFileExists(const char *filename) {
FILE *file;
if (file = fopen(filename, "r")) {
fclose(file);
return 1;
}
return 0;
}
このプログラムは、demo.txt
ファイルと C プログラムが同じ場所にあれば file exists
と表示します。C プログラムとファイル名が異なる場所にある場合は、ファイルのフルパスを指定する必要があります。
C 言語でファイルが存在するかどうかを確認する stat()
関数
ファイルからデータを読み込む代わりに stat()
関数を用いてファイルの属性を読み取ります。この関数は操作に成功すれば 0
を返し、そうでなければファイルが存在しなければ -1
を返します。
#include <stdio.h>
#include <sys/stat.h>
int checkIfFileExists(const char* filename);
int main(void) {
if (checkIfFileExists("demo.txt")) {
printf("file exists");
} else {
printf("file does not exists");
}
}
int checkIfFileExists(const char* filename) {
struct stat buffer;
int exist = stat(filename, &buffer);
if (exist == 0)
return 1;
else
return 0;
}
プログラムは、demo.txt
ファイルと C プログラムが同じ場所にあれば file exists
と表示します。C プログラムとファイル名が異なる場所にある場合は、ファイルのフルパスを指定しなければならません。
C 言語でファイルが存在するかどうかを調べるための access()
関数
ファイルが存在するかどうかを調べるもう一つの方法は、access()
関数を利用することです。unistd.h
のヘッダファイルには、ファイルが存在するかどうかを調べるための関数 access
が用意されています。
読み込み権限には R_OK
、書き込み権限には W_OK
、実行権限には X_OK
を用いることができます。R_OK|W_OK
のように併用することで、ファイルの読み込みと書き込みのパーミッションを得ることができます。
#include <stdio.h>
#include <unistd.h>
int main(void) {
if (access("C:\\TEMP\\demo.txt", F_OK) != -1) {
printf("file is found");
} else {
printf("file is not found");
}
return 0;
}
出力:
file is found
ここでは、C:\TEMPdemo.txt
がファイルの場所です。ファイルが存在すれば file is found
と表示され、そうでなければ file is not found
と表示されます。プログラムの場所と demo.txt
ファイルの場所は同じです。それ以外の場合はファイルの位置を指定する必要があります。Linux オペレーティングシステムでは、stat()
と access()
の組み合わせが良い選択です。
また、access()
関数を使用する別の方法は以下の通りです。
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
void checkIfFileExists(const char *fileName);
int main(void) {
char *fileName = "C:\\TEMP\\demo.txt";
checkIfFileExists(fileName);
return 0;
}
void checkIfFileExists(const char *fileName) {
if (!access(fileName, F_OK)) {
printf("The File %s was Found\n", fileName);
} else {
printf("The File %s not Found\n", fileName);
}
if (!access(fileName, R_OK)) {
printf("The File %s can be read\n", fileName);
} else {
printf("The File %s cannot be read\n", fileName);
}
if (!access(fileName, W_OK)) {
printf("The File %s can be Edited\n", fileName);
} else {
printf("The File %s cannot be Edited\n", fileName);
}
if (!access(fileName, X_OK)) {
printf("The File %s is an Executable\n", fileName);
} else {
printf("The File %s is not an Executable\n", fileName);
}
}
出力:
The file C:\TEMP\demo.txt was found
The file C:\TEMP\demo.txt can be read
The file C:\TEMP\demo.txt can be Edited
The file C:\TEMP\demo.txt is an executable