C 語言中如何檢查檔案是否存在
Satishkumar Bharadwaj
2023年10月12日
C
C File
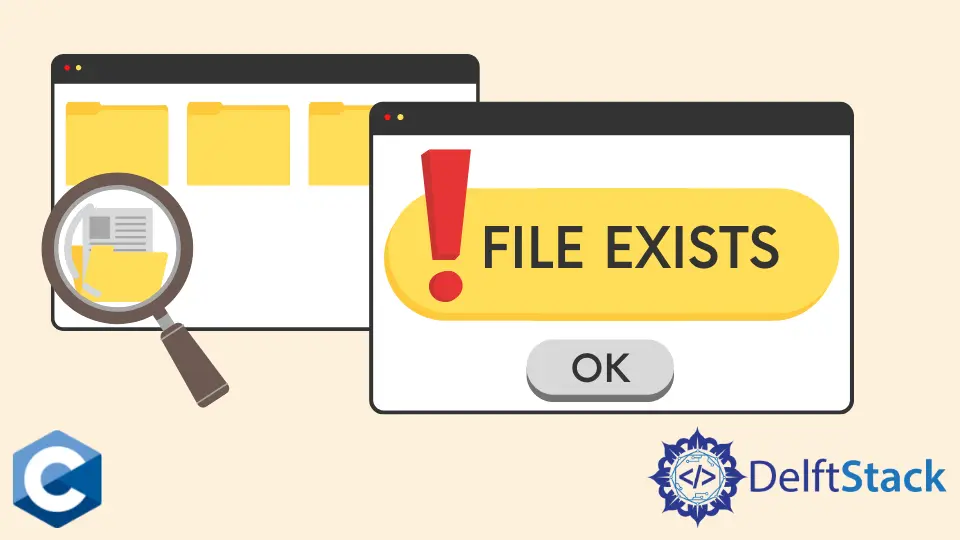
本教程介紹了用 C 語言檢查檔案是否存在的方法,檢查檔案是否存在的方法是嘗試以讀取或寫入模式開啟檔案。本程式是以讀取模式開啟一個檔案的。
fopen()
檢查 C 語言中檔案是否存在的函式
#include <stdio.h>
int main(void) {
FILE *file;
if (file = fopen("demo.txt", "r")) {
fclose(file);
printf("file exists");
} else {
printf("file doesn't exist");
}
return 0;
}
輸出:
file exists
這裡的檔名是 demo.txt
。C 程式和 demo.txt
檔案在同一個目錄下。所以輸出的結果是 file exists
。
如果 C 程式位置和檔案位置不同,我們必須指定檔案的完整路徑。
我們可以建立一個使用者自定義函式,檢查檔案是否存在。下面是帶有使用者自定義函式的程式。
#include <stdio.h>
int checkIfFileExists(const char *filename);
int main(void) {
if (checkIfFileExists("demo.txt")) {
printf("file exists");
} else {
printf("file does not exists");
}
}
int checkIfFileExists(const char *filename) {
FILE *file;
if (file = fopen(filename, "r")) {
fclose(file);
return 1;
}
return 0;
}
如果 demo.txt
檔案和 C 程式的位置相同,程式將列印 file exists
。如果 C 程式和檔名的位置不同,我們必須指定檔案的完整路徑。
stat()
函式檢查 C 程式中的檔案是否存在
我們使用 stat()
函式讀取檔案的屬性,而不是從檔案中讀取資料。如果操作成功,該函式將返回 0
;否則,將返回 -1
,如果檔案不存在。
#include <stdio.h>
#include <sys/stat.h>
int checkIfFileExists(const char* filename);
int main(void) {
if (checkIfFileExists("demo.txt")) {
printf("file exists");
} else {
printf("file does not exists");
}
}
int checkIfFileExists(const char* filename) {
struct stat buffer;
int exist = stat(filename, &buffer);
if (exist == 0)
return 1;
else
return 0;
}
如果 demo.txt
檔案和 C 程式的位置相同,程式將列印 file exists
。如果 C 程式和檔名的位置不同,我們必須指定檔案的完整路徑。
access()
檢查 C 程式中檔案是否存在的函式
檢查檔案是否存在的另一種方法是使用 access
函式。unistd.h
標頭檔案中有一個函式 access
來檢查檔案是否存在。
我們可以用 R_OK
表示讀許可權,W_OK
表示寫許可權,X_OK
表示執行許可權。我們可以將它們組合成 R_OK|W_OK
來讀取和寫入許可權。
#include <stdio.h>
#include <unistd.h>
int main(void) {
if (access("C:\\TEMP\\demo.txt", F_OK) != -1) {
printf("file is found");
} else {
printf("file is not found");
}
return 0;
}
輸出的結果是
file is found
這裡,C:TEMPdemo.txt
是檔案位置。如果檔案存在,則列印 file is found
;否則,列印 file is not found
。程式位置和 demo.txt
檔案的位置相同。否則,我們必須指定檔案的位置。對於 Linux 作業系統來說,stat()
和 access()
函式是一個不錯的選擇。
另一種使用 access 函式的方法如下。
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
void checkIfFileExists(const char *fileName);
int main(void) {
char *fileName = "C:\\TEMP\\demo.txt";
checkIfFileExists(fileName);
return 0;
}
void checkIfFileExists(const char *fileName) {
if (!access(fileName, F_OK)) {
printf("The File %s was Found\n", fileName);
} else {
printf("The File %s not Found\n", fileName);
}
if (!access(fileName, R_OK)) {
printf("The File %s can be read\n", fileName);
} else {
printf("The File %s cannot be read\n", fileName);
}
if (!access(fileName, W_OK)) {
printf("The File %s can be Edited\n", fileName);
} else {
printf("The File %s cannot be Edited\n", fileName);
}
if (!access(fileName, X_OK)) {
printf("The File %s is an Executable\n", fileName);
} else {
printf("The File %s is not an Executable\n", fileName);
}
}
輸出:
The file C:\TEMP\demo.txt was found
The file C:\TEMP\demo.txt can be read
The file C:\TEMP\demo.txt can be Edited
The file C:\TEMP\demo.txt is an executable
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe