Tkinter Tutorial - Checkbutton
- Tkinter Checkbutton Basic Example
- Tkinter Checkbutton Select/Deselect
- Tkinter Checkbutton State Toggle
- Tkinter Checkbutton Callback Function Binding
- Change Tkinter Checkbutton Default Value
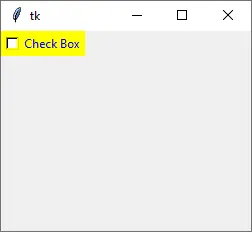
The Checkbutton
widget is a widget holding a value. Checkbuttons could contain either text or images. It could also link the callback function to be called when the checkbutton is clicked.
To create a checkbutton widget in an existing parent window parent, we can use,
tk.Checkbutton(parent, option, ...)
Tkinter Checkbutton Basic Example
import tkinter as tk
app = tk.Tk()
app.geometry("150x100")
chkValue = tk.BooleanVar()
chkValue.set(True)
chkExample = tk.Checkbutton(app, text="Check Box", var=chkValue)
chkExample.grid(column=0, row=0)
app.mainloop()
chkValue = tk.BooleanVar()
Each checkbutton should be associated with a variable. The data type of variable is determined by the checkbutton when selected/deselected.
chkValue.set(True)
It sets the original value of the checkbutton. As it is assigned with the Boolean
data type, you only have two options here, True
or False
.
chkExample = tk.Checkbutton(app, text="Check Box", var=chkValue)
Now the checkbutton instance is eventually created with the variable chkValue
we made above.
chkExample.grid(column=0, row=0)
grid
is another type of Tkinter layout managers besides pack
introduced in the section Tkinter Label.
Tkinter has three layout/geometry managers,
pack
grid
place
We will introduce these layout/geometry managers in one section of this tutorial.
Tkinter Checkbutton Select/Deselect
The user could click the checkbutton in the GUI to select or deselect the button. You could also select or deselect the checkbutton using the method select()
and deselect()
.
chkExample.select()
print("The checkbutton value when selected is {}".format(chkValue.get()))
chkExample.deselect()
print("The checkbutton value when deselected is {}".format(chkValue.get()))
The checkbutton value when selected is True
The checkbutton value when deselected is False
Here, the value of the checkbutton is obtained by the get()
method.
Tkinter Checkbutton State Toggle
The state of the checkbutton could be modified by select()
and deselect()
, and could also be toggled by using the toggle()
method.
import tkinter as tk
app = tk.Tk()
app.geometry("150x100")
chkValue = tk.BooleanVar()
chkValue.set(True)
chkExample = tk.Checkbutton(app, text="Check Box", var=chkValue)
chkExample.grid(column=0, row=0)
print("The checkbutton original value is {}".format(chkValue.get()))
chkExample.toggle()
print("The checkbutton value after toggled is {}".format(chkValue.get()))
chkExample.toggle()
print("The checkbutton value after toggled twice is {}".format(chkValue.get()))
app.mainloop()
The checkbutton original value is True
The checkbutton value after toggled is False
The checkbutton value after toggled twice is True
Tkinter Checkbutton Callback Function Binding
Checkbutton widget is used to select the states, and it could also bind the callback function to the event when it is selected/deselected, or more straightforward, toggled. Whenever the check button state is toggled, the call back function is triggered.
import tkinter as tk
from _cffi_backend import callback
def callBackFunc():
print("Oh. I'm clicked")
app = tk.Tk()
app.geometry("250x200")
chkValue = tk.BooleanVar()
chkValue.set(True)
chkExample = tk.Checkbutton(app, text="Check Box", var=chkValue, command=callBackFunc)
chkExample.grid(column=0, row=0)
app.mainloop()
Whenever you press the checkbutton, you could see it prints Oh. I'm clicked
in the console.
Option command
in Checkbutton class is meant to bind the call back function or method when the button is pressed.
Change Tkinter Checkbutton Default Value
The default value corresponding to the non-selected checkbutton is 0, and the default value of the selected checkbutton is 1. And you could also change checkbutton default values and their associated data type to the other value and/or data type.
import tkinter as tk
app = tk.Tk()
app.geometry("250x200")
chkValue = tk.StringVar()
chkExample = tk.Checkbutton(
app,
text="Check Box",
bg="gray",
fg="blue",
var=chkValue,
onvalue="RGB",
offvalue="YCbCr",
)
chkExample.grid(column=0, row=0)
chkExample.select()
print("The checkbutton value when selected is {}".format(chkValue.get()))
chkExample.deselect()
print("The checkbutton value when deselected is {}".format(chkValue.get()))
app.mainloop()
chkExample = tk.Checkbutton(
app,
text="Check Box",
bg="gray",
fg="blue",
var=chkValue,
onvalue="RGB",
offvalue="YCbCr",
)
The bg
sets the background color of the checkbutton, including the text area.
The fg
sets the foreground color of the checkbutton text.
onvalue
and offvalue
are options to change the selected and non-selected states’ values. They could have data types like Int
, String
, Float
, or others.
onvalue
and offvalue
. Otherwise, it raises a _tkinter.TclError
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook