Tkinter 튜토리얼-체크 버튼
- Tkinter Checkbutton 기본 예
- Tkinter Checkbutton 선택 / 선택 해제
- Tkinter Checkbutton 상태 토글
- Tkinter Checkbutton 콜백 함수 바인딩
- Tkinter Checkbutton 기본값 변경
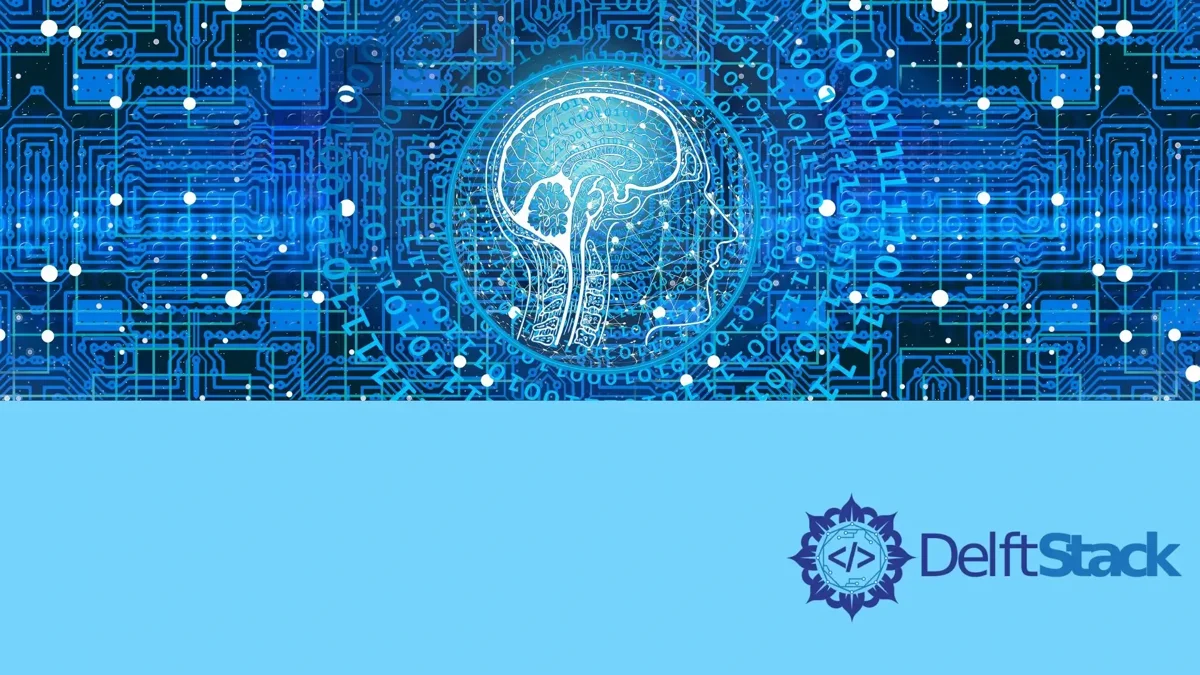
체크 버튼 위젯은 값을 유지하는 위젯이다. 체크 버튼에는 텍스트 또는 이미지가 포함될 수 있습니다. 체크 버튼을 클릭했을 때 호출되는 콜백 함수를 연결할 수도 있습니다.
기존 부모 창 부모에 체크 버튼 위젯을 만들려면
tk.Checkbutton(parent, option, ...)
Tkinter Checkbutton 기본 예
import tkinter as tk
app = tk.Tk()
app.geometry("150x100")
chkValue = tk.BooleanVar()
chkValue.set(True)
chkExample = tk.Checkbutton(app, text="Check Box", var=chkValue)
chkExample.grid(column=0, row=0)
app.mainloop()
chkValue = tk.BooleanVar()
각 체크 버튼은 변수와 연결되어야합니다. 변수의 데이터 유형은 선택 / 선택 해제 될 때 체크 버튼으로 결정됩니다.
chkValue.set(True)
체크 버튼의 원래 값을 설정합니다. 방금 Boolean
데이터 형식이 할당되었으므로 여기에는 True 또는 False 의 두 가지 옵션 만 있습니다.
chkExample = tk.Checkbutton(app, text="Check Box", var=chkValue)
이제 체크 버튼 인스턴스는 위에서 만든 변수 chkValue
를 사용하여 만들어집니다.
chkExample.grid(column=0, row=0)
grid
는 Tkinter Label 섹션에 소개 된 pack
외에 다른 유형의 Tkinter 레이아웃 관리자입니다.
Tkinter 에는 3 개의 레이아웃 / 지오메트리 관리자가 있습니다.
- 팩
- 그리드
- 장소
이 튜토리얼의 한 섹션에서 이러한 레이아웃 / 지오메트리 관리자를 소개합니다.
Tkinter Checkbutton 선택 / 선택 해제
사용자는 GUI 에서 확인 버튼을 클릭하여 버튼을 선택하거나 선택 취소 할 수 있습니다. select()
및 deselect()
메소드를 사용하여 체크 버튼을 선택하거나 선택 해제 할 수도 있습니다.
chkExample.select()
print "The checkbutton value when selected is {}".format(chkValue.get())
chkExample.select()
print "The checkbutton value when deselected is {}".format(chkValue.get())
The checkbutton value when selected is True
The checkbutton value when deselected is False
여기서 체크 버튼의 값은 get() 메소드에 의해 얻어진다.
Tkinter Checkbutton 상태 토글
체크 버튼의 상태는 select()
와 deselect()
에 의해 수정 될 수 있으며 toggle()
메소드를 사용하여 토글 될 수도 있습니다.
import tkinter as tk
app = tk.Tk()
app.geometry("150x100")
chkValue = tk.BooleanVar()
chkValue.set(True)
chkExample = tk.Checkbutton(app, text="Check Box", var=chkValue)
chkExample.grid(column=0, row=0)
print "The checkbutton original value is {}".format(chkValue.get())
chkExample.toggle()
print "The checkbutton value after toggled is {}".format(chkValue.get())
chkExample.toggle()
print "The checkbutton value after toggled twice is {}".format(chkValue.get())
app.mainloop()
The checkbutton original value is True
The checkbutton value after toggled is False
The checkbutton value after toggled twice is True
Tkinter Checkbutton 콜백 함수 바인딩
체크 버튼 위젯은 상태를 선택하는 데 사용되며 콜백 기능을 선택 / 선택 취소하거나 더 간단하게 전환 할 때 이벤트에 바인딩 할 수도 있습니다. 확인 버튼 상태가 전환 될 때마다 콜백 기능이 트리거됩니다.
import tkinter as tk
from _cffi_backend import callback
def callBackFunc():
print "Oh. I'm clicked"
app = tk.Tk()
app.geometry("150x100")
chkValue = tk.BooleanVar()
chkValue.set(True)
chkExample = tk.Checkbutton(app, text="Check Box", var=chkValue, command=callBackFunc)
chkExample.grid(column=0, row=0)
app.mainloop()
체크 버튼을 누를 때마다 Oh. I'm clicked
Checkbutton 클래스의 옵션 command
는 버튼을 눌렀을 때 콜백 함수 또는 메소드를 바인딩하기위한 것입니다.
Tkinter Checkbutton 기본값 변경
선택되지 않은 체크 버튼에 해당하는 기본값은 0이고 선택한 체크 버튼의 기본값은 1입니다. 또한 체크 버튼 기본값 및 관련 데이터 형식을 다른 값 및 / 또는 데이터 형식으로 변경할 수도 있습니다.
import tkinter as tk
app = tk.Tk()
app.geometry("150x100")
chkValue = tk.StringVar()
chkExample = tk.Checkbutton(
app, text="Check Box", var=chkValue, onvalue="RGB", offvalue="YCbCr"
)
chkExample.grid(column=0, row=0)
chkExample.select()
print "The checkbutton value when selected is {}".format(chkValue.get())
chkExample.deselect()
print "The checkbutton value when deselected is {}".format(chkValue.get())
app.mainloop()
The checkbutton value when selected is RGB
The checkbutton value when deselected is YCbCr
chkExample = tk.Checkbutton(
app, text="Check Box", var=chkValue, onvalue="RGB", offvalue="YCbCr"
)
onvalue
및 offvalue
는 선택된 상태와 선택되지 않은 상태의 값을 변경하는 옵션입니다. Int
,String
,Float
등의 데이터 유형을 가질 수 있습니다.
_tkinter.TclError
를보고합니다Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook