How to Rotate Axis Tick Labels of Seaborn Plots
-
Use the
set_xticklabels()
Function to Rotate Labels on Seaborn Axes -
Use the
xticks()
Function to Rotate Labels on Seaborn Axes -
Use the
setp()
Function to Rotate Labels on on Seaborn Axes
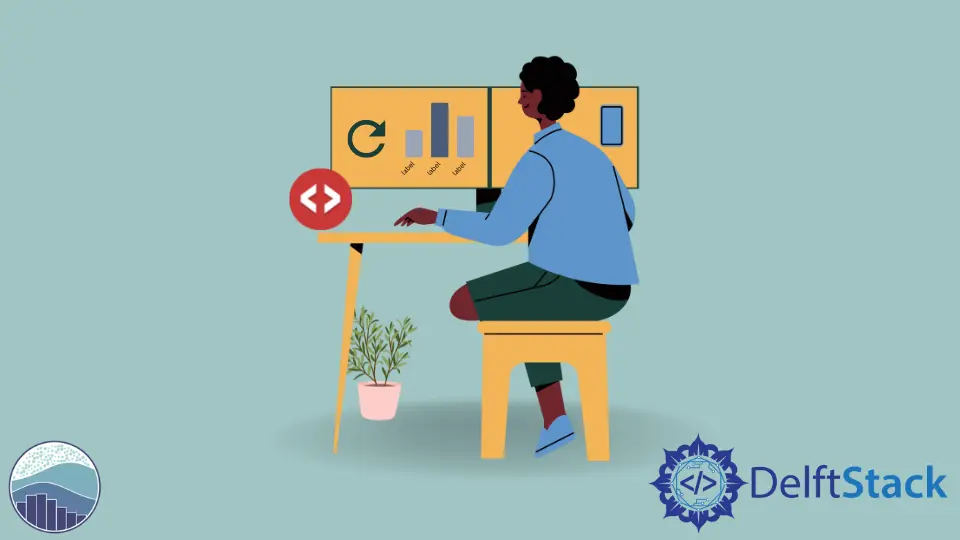
Seaborn offers a lot of customizations for the final figure. One such small but essential customization is that we can control the tick labels on both axes.
For example, pay attention to the problem with the following graph.
import pandas as pd
import matplotlib.pyplot as plt
import seaborn as sns
df = pd.DataFrame(
{
"Date": [
"01012019",
"01022019",
"01032019",
"01042019",
"01052019",
"01062019",
"01072019",
"01082019",
],
"Price": [77, 76, 68, 70, 78, 79, 74, 75],
}
)
df["Date"] = pd.to_datetime(df["Date"], format="%d%m%Y")
plt.figure(figsize=(15, 8))
ax = sns.barplot(x="Date", y="Price", data=df)
In the above code, we plotted a graph for the time series data of the price of a product. As you can see, whole dates are plotted on the x-axis. Due to this, everything is overlapped and is difficult to read.
For such situations, we can rotate the tick labels on the axis.
In this tutorial, we will learn how to rotate such tick labels on seaborn plots.
Use the set_xticklabels()
Function to Rotate Labels on Seaborn Axes
The set_xticklabels()
function sets the values for tick labels on the x-axis. We can use it to rotate the labels. However, this function needs some label values to use the get_xticklabels()
function that returns the default labels and rotates them using the rotation
parameter.
The following code demonstrates its use.
import pandas as pd
import matplotlib.pyplot as plt
import seaborn as sns
df = pd.DataFrame(
{
"Date": [
"01012019",
"01022019",
"01032019",
"01042019",
"01052019",
"01062019",
"01072019",
"01082019",
],
"Price": [77, 76, 68, 70, 78, 79, 74, 75],
}
)
df["Date"] = pd.to_datetime(df["Date"], format="%d%m%Y")
plt.figure(figsize=(15, 8))
ax = sns.barplot(x="Date", y="Price", data=df)
ax.set_xticklabels(ax.get_xticklabels(), rotation=30)
Use the xticks()
Function to Rotate Labels on Seaborn Axes
The rotation
parameter in the matplotlib.pyplot.xticks()
function can also achieve this. We do not need to pass any labels and can directly use the parameter in this function.
For example,
import pandas as pd
import matplotlib.pyplot as plt
import seaborn as sns
df = pd.DataFrame(
{
"Date": [
"01012019",
"01022019",
"01032019",
"01042019",
"01052019",
"01062019",
"01072019",
"01082019",
],
"Price": [77, 76, 68, 70, 78, 79, 74, 75],
}
)
df["Date"] = pd.to_datetime(df["Date"], format="%d%m%Y")
plt.figure(figsize=(15, 8))
ax = sns.barplot(x="Date", y="Price", data=df)
plt.xticks(rotation=45)
Use the setp()
Function to Rotate Labels on on Seaborn Axes
Since most seaborn plots return a matplotlib axes object, we can use the setp()
function from this library. We will take the tick label values using the xtick()
function and rotate them using the rotation
parameter of the setp()
function.
See the following code.
import pandas as pd
import matplotlib.pyplot as plt
import seaborn as sns
df = pd.DataFrame(
{
"Date": [
"01012019",
"01022019",
"01032019",
"01042019",
"01052019",
"01062019",
"01072019",
"01082019",
],
"Price": [77, 76, 68, 70, 78, 79, 74, 75],
}
)
df["Date"] = pd.to_datetime(df["Date"], format="%d%m%Y")
plt.figure(figsize=(15, 8))
ax = sns.barplot(x="Date", y="Price", data=df)
locs, labels = plt.xticks()
plt.setp(labels, rotation=45)
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn