How to Add Axis Labels to Seaborn Plot
-
Use the
set_xlabel()
andset_ylabel()
Functions to Set the Axis Labels in a Seaborn Plot -
Use the
set()
Function to Set the Axis Labels in a Seaborn Plot -
Use the
matplotlib.pyplot.xlabel()
andmatplotlib.pyplot.ylabel()
Functions to Set the Axis Labels of a Seaborn Plot
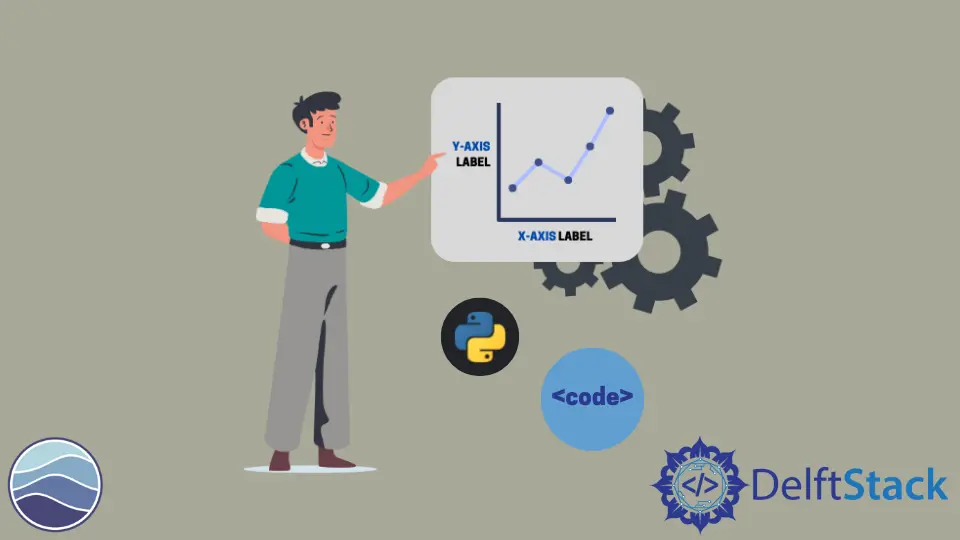
In this tutorial, we will discuss how to add x and y-axis labels to a seaborn plot in Python.
By default, when we specify the values for x and y-axis in the plot function, the graph takes these values as the labels for both the axis. We will be discussing other methods to explicitly add our desired axis labels.
Use the set_xlabel()
and set_ylabel()
Functions to Set the Axis Labels in a Seaborn Plot
A seaborn plot returns a matplotlib axes instance type object. We can use the set_xlabel()
and set_ylabel
to set the x and y-axis label respectively.
For example,
import pandas as pd
import matplotlib.pyplot as plt
import seaborn as sns
df = pd.DataFrame(
{"Day 1": [7, 1, 5, 6, 3, 10, 5, 8], "Day 2": [1, 2, 8, 4, 3, 9, 5, 2]}
)
p = sns.lineplot(data=df)
p.set_xlabel("X-Axis", fontsize=20)
p.set_ylabel("Y-Axis", fontsize=20)
We can use the fontsize
parameter to control the size of the font.
Use the set()
Function to Set the Axis Labels in a Seaborn Plot
The set()
function is used to add different elements to the plot and can be used to add the axis labels. We use the xlabel
and ylabel
parameters to specify the labels.
For example,
import pandas as pd
import matplotlib.pyplot as plt
import seaborn as sns
df = pd.DataFrame(
{"Day 1": [7, 1, 5, 6, 3, 10, 5, 8], "Day 2": [1, 2, 8, 4, 3, 9, 5, 2]}
)
p = sns.lineplot(data=df)
p.set(xlabel="X-Axis", ylabel="Y-Axis")
Use the matplotlib.pyplot.xlabel()
and matplotlib.pyplot.ylabel()
Functions to Set the Axis Labels of a Seaborn Plot
These functions are used to set the labels for both the axis of the current plot. Different arguments like size
, fontweight
, fontsize
can be used to alter the size and shape of the labels.
The following code demonstrates their use.
import pandas as pd
import matplotlib.pyplot as plt
import seaborn as sns
df = pd.DataFrame(
{"Day 1": [7, 1, 5, 6, 3, 10, 5, 8], "Day 2": [1, 2, 8, 4, 3, 9, 5, 2]}
)
p = sns.lineplot(data=df)
plt.xlabel("X-Axis")
plt.ylabel("Y-Axis")
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn