What Is the Difference Between List Methods Append and Extend
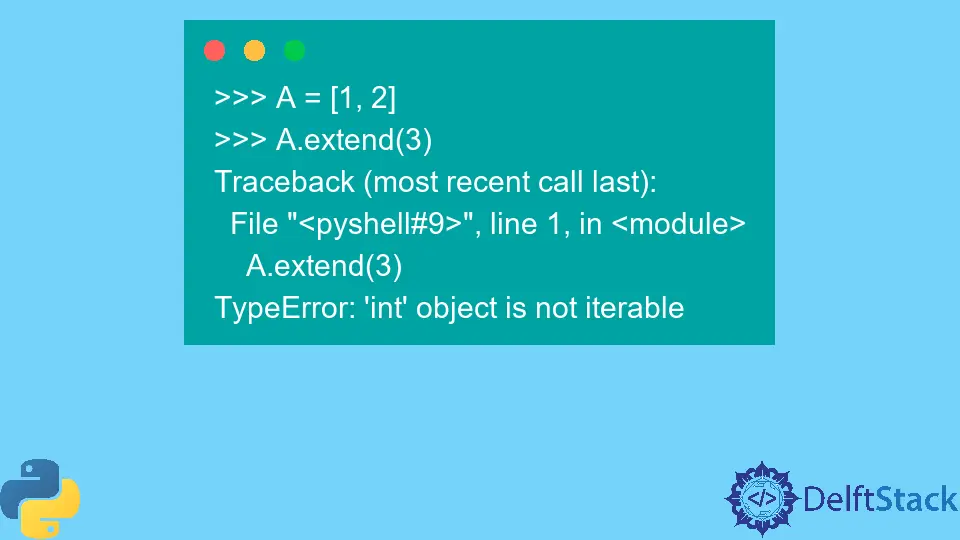
In Python, working with lists is a fundamental aspect of programming. Two commonly used methods for adding elements to a list are append
and extend
. While they may seem similar at first glance, they serve different purposes and can lead to different outcomes when manipulating lists. Understanding these differences is crucial for writing efficient and effective Python code.
In this tutorial, we will explore the nuances between append
and extend
, providing clear examples to illustrate how each method functions. By the end, you’ll have a solid grasp of when to use each method, enhancing your Python programming skills.
Understanding Append
The append
method is used to add a single element to the end of a list. This method modifies the original list in place and does not return a new list. When using append
, you can add any object, such as a number, string, or even another list, but it will always be added as a single element.
Here’s a simple example of how the append
method works:
my_list = [1, 2, 3]
my_list.append(4)
print(my_list)
Output:
[1, 2, 3, 4]
In this example, we started with a list containing the numbers 1, 2, and 3. By calling my_list.append(4)
, we added the number 4 to the end of the list. The output shows that the original list has been modified to include the new element.
Another interesting aspect of append
is its ability to add other data types, including lists. For instance:
my_list.append([5, 6])
print(my_list)
Output:
[1, 2, 3, 4, [5, 6]]
In this case, we appended a list [5, 6]
to my_list
. The result demonstrates that the entire list is treated as a single element, leading to a nested list structure. This behavior is essential to understand when working with lists in Python, as it can affect how you access and manipulate data later.
Understanding Extend
On the other hand, the extend
method is designed to add multiple elements to a list at once. Unlike append
, which adds a single element, extend
takes an iterable (like a list, tuple, or string) and adds each of its elements to the end of the list. This method also modifies the original list in place and does not return a new list.
Let’s look at an example of how extend
works:
my_list = [1, 2, 3]
my_list.extend([4, 5])
print(my_list)
Output:
[1, 2, 3, 4, 5]
In this example, we used extend
to add the elements of the list [4, 5]
to my_list
. The output shows that both 4 and 5 have been added separately, resulting in a flat list. This is a key difference from append
, which would have added the entire list as a single element.
Another example can further illustrate the versatility of extend
:
my_list.extend((6, 7))
print(my_list)
Output:
[1, 2, 3, 4, 5, 6, 7]
Here, we extended my_list
with a tuple (6, 7)
. Similar to the previous example, each element of the tuple is added individually to the list. This method is particularly useful when you want to concatenate lists or combine multiple iterables seamlessly.
Key Differences Between Append and Extend
Now that we have explored both methods, it’s essential to summarize the key differences between append
and extend
:
- Functionality:
append
adds a single element to the end of a list, whileextend
adds multiple elements from an iterable. - Output Structure: When using
append
, if you add a list, it becomes a nested element. Conversely,extend
flattens the iterable and adds its contents directly to the list. - Use Cases: Use
append
when you want to add a single item or a nested structure. Useextend
when you want to merge multiple elements into the list.
Understanding these differences will help you choose the right method based on your specific needs in Python programming.
Conclusion
In summary, the append
and extend
methods in Python serve distinct purposes when working with lists. While append
is ideal for adding single elements, extend
shines when you need to add multiple elements from an iterable. Knowing when to use each method can greatly enhance your coding efficiency and effectiveness. As you continue to explore Python, keep these differences in mind to make the most of your list manipulation skills.
FAQ
-
What does the append method do in Python?
The append method adds a single element to the end of a list. -
Can I use append to add multiple elements at once?
No, append only adds one element at a time. To add multiple elements, use extend. -
What happens if I append a list to another list?
The entire list is added as a single nested element. -
Is extend more efficient than append for adding multiple items?
Yes, extend is designed to add multiple items at once, making it more efficient for that purpose. -
Can I use extend with different data types?
Yes, extend can take any iterable, including lists, tuples, and strings.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook