How to Set a Tkinter Window With a Constant Size
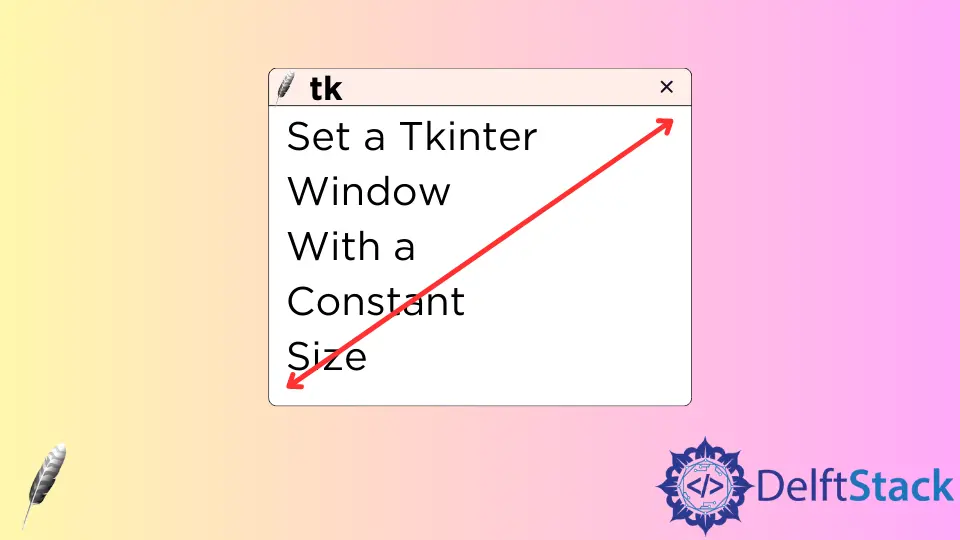
The Tkinter window size is by default resizable even if you assign the width and height when the window instance is initiated.
import tkinter as tk
app = tk.Tk()
app.geometry("200x200")
app.mainloop()
You could drag the window created by the above codes to get different window sizes. resizable
function should be used to restrict the width and height size.
resizable(self, width=None, height=None)
- Instruct the window manager whether this width can be resized in WIDTH or HEIGHT. Both values are boolean values.import tkinter as tk
app = tk.Tk()
app.geometry("200x200")
app.resizable(width=0, height=0)
app.mainloop()
app.resizable(width=0, height=0)
doesn’t allow both width and height to be resized.
Set the Tkinter Frame With a Constant Size
The frame inside the window is a bit similar to the window, in the sense that it automatically resizes itself even if you define the width and height of that frame.
import tkinter as tk
app = tk.Tk()
app.geometry("200x200")
app.resizable(0, 0)
backFrame = tk.Frame(master=app, width=200, height=200, bg="blue").pack()
button1 = tk.Button(master=backFrame, text="Button 1", bg="blue", fg="red").pack()
button2 = tk.Button(master=backFrame, text="Button 2", bg="blue", fg="green").pack()
button3 = tk.Label(master=backFrame, text="Button 3", bg="red", fg="blue").pack()
app.mainloop()
The GUI what we expected is like below,
But what we get after executing the above codes is,
The frame backFrame
resizes automatically to fit the widgets attached to it. Or in other words, the widgets inside backFrame
control their parent frame’s size.
You could disable the frame to resize to its widgets by setting pack_propagate(0)
.
propagate(self, flag=['_noarg_'])
-Set or get the status for propagation of geometry information. A boolean argument specifies whether the geometry information of the slaves will determine the size of this widget. If no argument is given the current setting will be returned.The correct code to freeze the frame size is,
import tkinter as tk
app = tk.Tk()
app.geometry("200x200")
app.resizable(0, 0)
backFrame = tk.Frame(master=app, width=200, height=200, bg="blue")
backFrame.pack()
backFrame.propagate(0)
button1 = tk.Button(master=backFrame, text="Button 1", bg="blue", fg="red").pack()
button2 = tk.Button(master=backFrame, text="Button 2", bg="blue", fg="green").pack()
button3 = tk.Label(master=backFrame, text="Button 3", bg="red", fg="blue").pack()
app.mainloop()
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook