일정한 크기로 Tkinter 창을 설정하는 방법
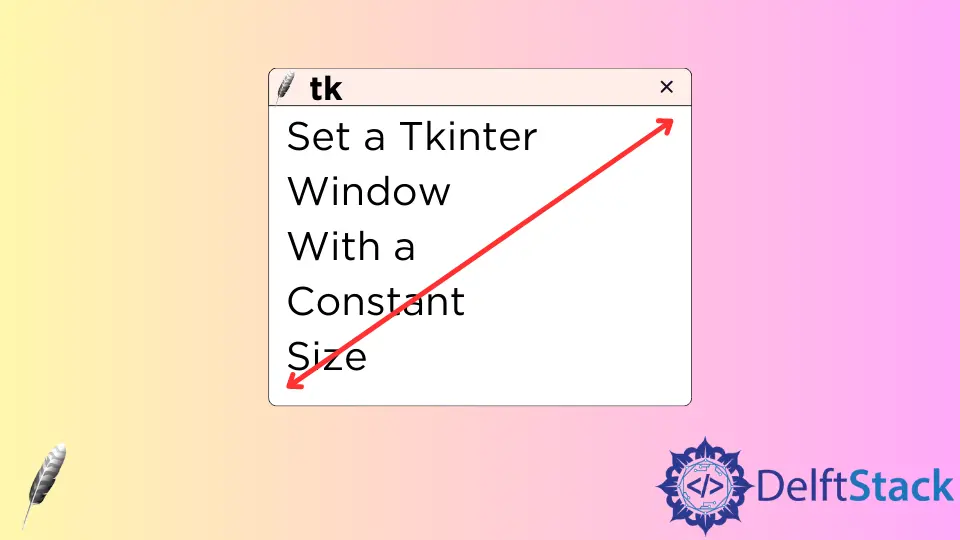
윈도우 인스턴스가 시작될 때 너비와 높이를 할당하더라도 Tkinter 윈도우 크기는 기본적으로 크기를 조정할 수 있습니다.
import tkinter as tk
app = tk.Tk()
app.geometry("200x200")
app.mainloop()
위의 코드로 만든 창을 끌어서 다른 창 크기를 얻을 수 있습니다. ‘크기 조정 가능’기능을 사용하여 너비와 높이 크기를 제한해야합니다.
resizable(self, width=None, height=None)
-이 너비를 WIDTH 또는 HEIGHT 로 조정할 수 있는지 창 관리자에게 지시합니다. 두 값 모두 부울 값입니다.import tkinter as tk
app = tk.Tk()
app.geometry("200x200")
app.resizable(width=0, height=0)
app.mainloop()
app.resizable(width=0, height=0)
에서는 너비와 높이를 모두 조정할 수 없습니다.
일정한 크기로 Tkinter 프레임 설정
창 내부의 프레임은 창과 약간 유사합니다. 즉, 프레임의 너비와 높이를 정의하더라도 자동으로 크기가 조정됩니다.
import tkinter as tk
app = tk.Tk()
app.geometry("200x200")
app.resizable(0, 0)
backFrame = tk.Frame(master=app, width=200, height=200, bg="blue").pack()
button1 = tk.Button(master=backFrame, text="Button 1", bg="blue", fg="red").pack()
button2 = tk.Button(master=backFrame, text="Button 2", bg="blue", fg="green").pack()
button3 = tk.Label(master=backFrame, text="Button 3", bg="red", fg="blue").pack()
app.mainloop()
우리가 예상 한 GUI 는 다음과 같습니다.
그러나 위 코드를 실행 한 후에 얻는 것은
프레임 backFrame
은 연결된 위젯에 맞게 자동으로 크기가 조정됩니다. 즉, backFrame
내부의 위젯은 부모 프레임의 크기를 제어합니다.
pack_propagate(0)
을 설정하여 위젯의 크기를 조정하도록 프레임을 비활성화 할 수 있습니다.
propagate(self, flag=['_noarg_'])
-지오메트리 정보의 전파 상태를 설정하거나 가져옵니다. 부울 인수는 슬레이브의 지오메트리 정보가이 위젯의 크기를 결정할지 여부를 지정합니다. 인수가 없으면 현재 설정이 반환됩니다.프레임 크기를 고정하는 올바른 코드는
import tkinter as tk
app = tk.Tk()
app.geometry("200x200")
app.resizable(0, 0)
backFrame = tk.Frame(master=app, width=200, height=200, bg="blue")
backFrame.pack()
backFrame.propagate(0)
button1 = tk.Button(master=backFrame, text="Button 1", bg="blue", fg="red").pack()
button2 = tk.Button(master=backFrame, text="Button 2", bg="blue", fg="green").pack()
button3 = tk.Label(master=backFrame, text="Button 3", bg="red", fg="blue").pack()
app.mainloop()
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook