How to Read or Parse CSV File in PHP
-
Use
fread()
to Read CSV File in PHP -
Use
readfile()
to Read CSV File in PHP -
Use the
str_getcsv()
Function to Parse CSV in Python -
Use the
fgetcsv()
Function to Parse CSV in Python
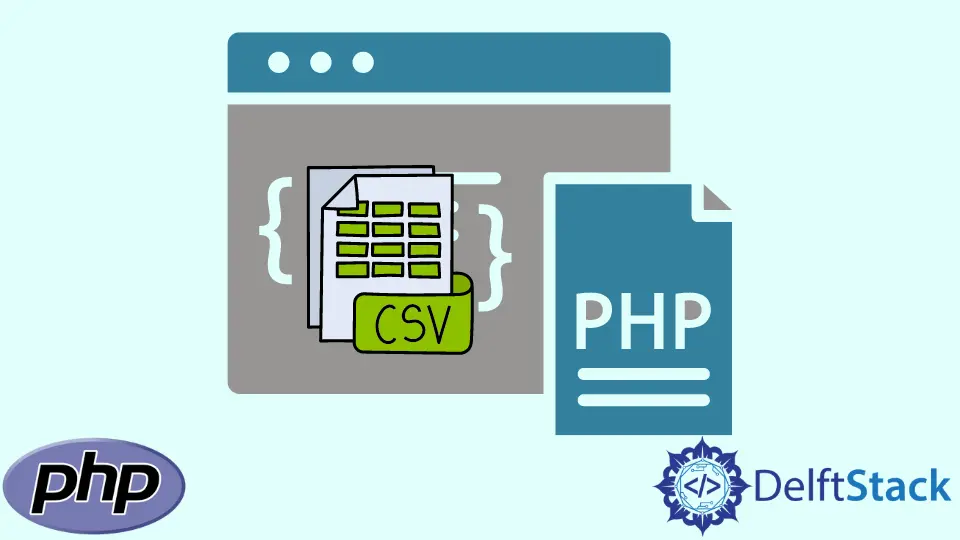
File handling is an essential component of any web application.
This tutorial will introduce how to read, write, and append files using file handling.
Use fread()
to Read CSV File in PHP
It is the basic function of reading the CSV file in PHP. It reads the file and returns all contents present in the file.
See the example code.
<?php
$file = "text1.csv";
$openfile = fopen($file, "r");
$cont = fread($openfile, filesize($file));
echo $cont;
?>
Output:
aaa,bbb,ccc,dddd
123,456,789
"""aaa""","""bbb"""
fread()
requires two parameters: the file from which we want to read data and the size of the file that we can obtain by passing the file as a parameter to the function filesize($file)
.
Use readfile()
to Read CSV File in PHP
This function reads a file and saves the results to memory or cache. It opens the file and reads the file’s content. It only accepts one parameter, which is the file.
<?php
echo readfile("text1.csv");
?>
Ouput:
aaa,bbb,ccc,dddd
123,456,789
"""aaa""","""bbb"""
49
The fread()
fuction only reads the file and returns the data in the editor, but the readfile()
function reads a file but also save its result to memory or cache.
Use the str_getcsv()
Function to Parse CSV in Python
This function parses a string in CSV format and returns an array containing the file’s data. It converts the data from a CSV file into an array, but before you run it, you should open the file using the fopen()
function, which takes file and mode as parameters. See the following example.
<?php
$handle = fopen("text1.csv", "r");
$lineNumber = 1;
while (($raw_string = fgets($handle)) !== false) {
$row = str_getcsv($raw_string);
var_dump($row);
$lineNumber++;
}
fclose($handle);
?>
Output:
array(4) {
[0]=>
string(3) "aaa"
[1]=>
string(3) "bbb"
[2]=>
string(3) "ccc"
[3]=>
string(4) "dddd"
}
array(3) {
[0]=>
string(3) "123"
[1]=>
string(3) "456"
[2]=>
string(3) "789"
}
array(2) {
[0]=>
string(5) ""aaa""
[1]=>
string(5) ""bbb""
}
Use the fgetcsv()
Function to Parse CSV in Python
Now, we’ll use a cool function named fgetcsv()
to parse the data from a CSV file. The following steps are needed to use this function.
- Open the file to access file data from a file
- Use the
fgetcsv()
Function within a loop to parse each row of the file separately. - Close the file
See the example code.
<?php
$file = fopen('text1.csv', 'r');
while (($line = fgetcsv($file)) !== FALSE) {
echo '<pre>';
print_r($line);
echo '</pre>';
}
fclose($file);
?>
Output:
<pre>Array
(
[0] => aaa
[1] => bbb
[2] => ccc
[3] => dddd
)
</pre><pre>Array
(
[0] => 123
[1] => 456
[2] => 789
)
</pre><pre>Array
(
[0] => "aaa"
[1] => "bbb"
)
</pre>