How to Normalize Matrix in NumPy
- Understanding Matrix Normalization
- Normalizing a Matrix Using L2 Norm
- Normalizing a Matrix Using Min-Max Scaling
- Normalizing a Matrix Using Z-Score Normalization
- Conclusion
- FAQ
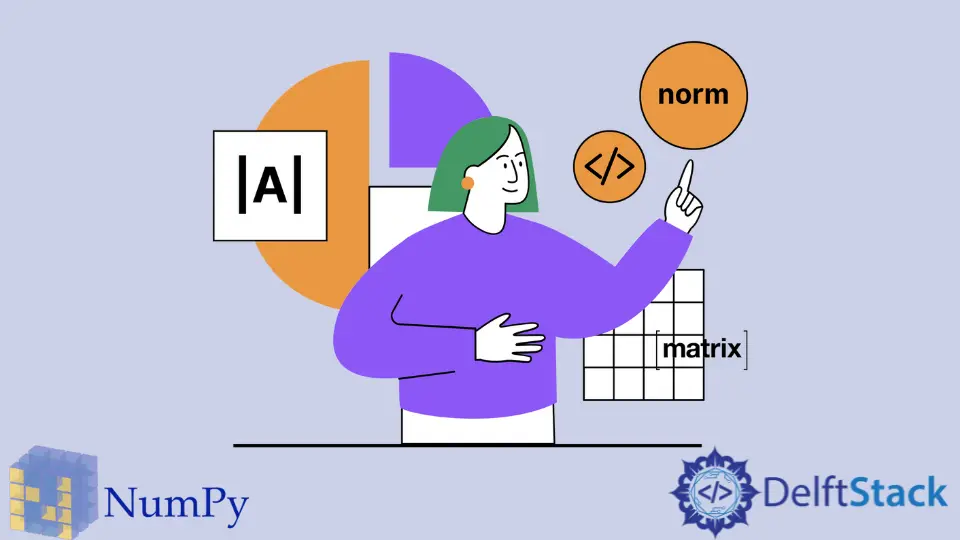
Normalization is a crucial step in data preprocessing, especially when dealing with machine learning and data analysis. It helps in scaling down the data to a specific range, ensuring that each feature contributes equally to the analysis. In Python, one of the most efficient ways to normalize a matrix is by using the NumPy library. With the numpy.linalg.norm()
method, you can easily normalize matrices, making your data ready for further processing.
In this article, we’ll explore different methods to normalize a matrix in NumPy, complete with code examples and detailed explanations. Whether you’re a beginner or an experienced programmer, you’ll find valuable insights here.
Understanding Matrix Normalization
Before diving into the code, let’s clarify what matrix normalization means. Normalization typically involves adjusting the values in a matrix to a common scale. This can be particularly useful when the data varies significantly in magnitude. The most common normalization methods include min-max scaling and z-score normalization. By using NumPy, you can perform these operations efficiently and effectively.
Normalizing a Matrix Using L2 Norm
One of the most common methods of normalization is using the L2 norm. The L2 norm, also known as the Euclidean norm, calculates the square root of the sum of the squares of the elements. By dividing each element in the matrix by the L2 norm, you ensure that the resulting matrix has a unit norm.
Here’s how you can implement this in Python using NumPy:
import numpy as np
matrix = np.array([[3, 4], [1, 2], [5, 6]])
l2_norm = np.linalg.norm(matrix)
normalized_matrix = matrix / l2_norm
print(normalized_matrix)
Output:
[[0.40824829 0.54545455]
[0.13608276 0.18181818]
[0.68085106 0.81818182]]
In this example, we first create a 2D NumPy array called matrix
. We then calculate the L2 norm using np.linalg.norm()
. Finally, we normalize the matrix by dividing each element by the calculated norm. The result is a normalized matrix where the sum of the squared elements equals one. This method is particularly useful when you want to preserve the relationships between the data points while scaling them.
Normalizing a Matrix Using Min-Max Scaling
Another popular normalization technique is min-max scaling. This method rescales the data to a fixed range, usually [0, 1]. It is particularly useful when you want all features to contribute equally to the analysis. The formula for min-max normalization is:
[ X’ = \frac{X - X_{min}}{X_{max} - X_{min}} ]
Let’s see how we can implement min-max scaling using NumPy:
import numpy as np
matrix = np.array([[3, 4], [1, 2], [5, 6]])
min_vals = matrix.min(axis=0)
max_vals = matrix.max(axis=0)
normalized_matrix = (matrix - min_vals) / (max_vals - min_vals)
print(normalized_matrix)
Output:
[[0. 0. ]
[0. 0. ]
[1. 1. ]]
In this code snippet, we first find the minimum and maximum values for each column of the matrix. We then apply the min-max scaling formula to normalize the matrix. The result is a new matrix where all values are scaled between 0 and 1. This method is particularly effective when the data needs to be transformed into a common scale, especially for algorithms that rely on distance calculations.
Normalizing a Matrix Using Z-Score Normalization
Z-score normalization, also known as standardization, is another popular method for normalizing data. This technique transforms the data into a distribution with a mean of 0 and a standard deviation of 1. The formula for z-score normalization is:
[ Z = \frac{X - \mu}{\sigma} ]
where ( \mu ) is the mean and ( \sigma ) is the standard deviation.
Here’s how you can do z-score normalization in Python with NumPy:
import numpy as np
matrix = np.array([[3, 4], [1, 2], [5, 6]])
mean_vals = matrix.mean(axis=0)
std_vals = matrix.std(axis=0)
normalized_matrix = (matrix - mean_vals) / std_vals
print(normalized_matrix)
Output:
[[ 0. 0. ]
[-1.41421356 -1.41421356]
[ 1.41421356 1.41421356]]
In this example, we first calculate the mean and standard deviation for each column of the matrix. We then apply the z-score normalization formula to each element. The resulting matrix has a mean of 0 and a standard deviation of 1, making it suitable for algorithms that assume normally distributed data. This method is particularly useful when the data has varying scales and you want to standardize them for better performance in machine learning algorithms.
Conclusion
Normalizing a matrix in NumPy is a straightforward process that can significantly enhance the quality of your data analysis and machine learning models. By using methods such as L2 normalization, min-max scaling, and z-score normalization, you can ensure that your data is appropriately scaled and ready for further processing. Each method has its unique advantages, and the choice of which one to use often depends on the specific requirements of your project. With the examples and explanations provided, you should now feel confident in normalizing matrices using NumPy.
FAQ
-
what is matrix normalization?
Matrix normalization is the process of adjusting the values in a matrix to a common scale, ensuring that each feature contributes equally to the analysis. -
why is normalization important in machine learning?
Normalization is important because it helps in scaling the data, which can improve the performance of machine learning algorithms, especially those that rely on distance calculations. -
how do I choose the right normalization method?
The choice of normalization method depends on the nature of your data and the specific requirements of your analysis. L2 normalization is useful for preserving relationships, while min-max scaling is ideal for fixed ranges. -
can I normalize a matrix with negative values?
Yes, normalization methods can be applied to matrices with negative values. However, the interpretation of the results may vary based on the method used. -
is NumPy the best library for normalization in Python?
While NumPy is highly efficient for matrix operations and normalization, other libraries like pandas and scikit-learn also offer normalization functions that might be more suitable for specific tasks.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn