NumPy Matrix Vector Multiplication
- Understanding Matrix Vector Multiplication
- Using numpy.matmul() Method
- Using numpy.dot() Method
- Conclusion
- FAQ
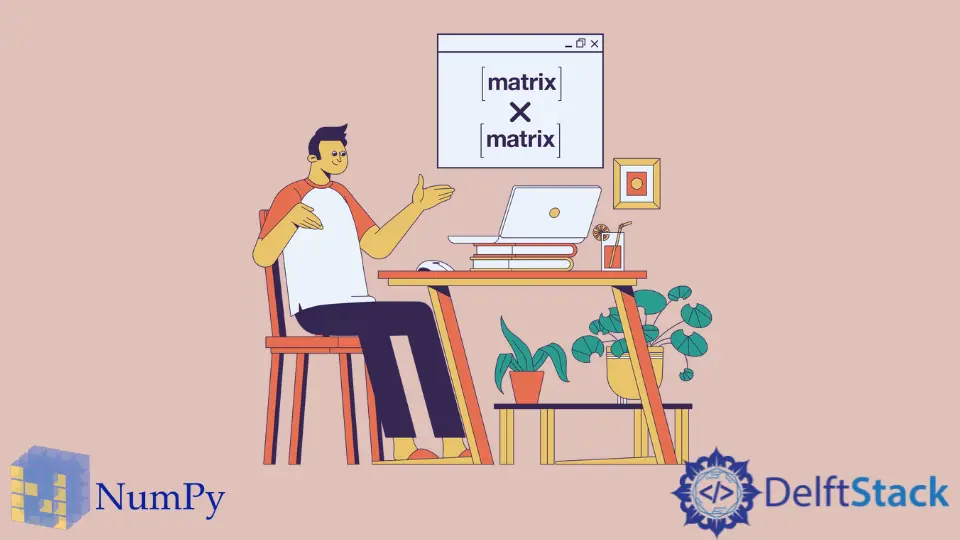
Matrix vector multiplication is a fundamental operation in numerical computing and data analysis. In Python, the NumPy library provides powerful tools for performing these operations efficiently. The two primary methods for multiplying matrices in NumPy are the numpy.matmul()
method and the numpy.dot()
method. Each method has its unique features and use cases, making it essential to understand when to use which one.
In this article, we will explore both methods in detail, including code examples and explanations. By the end, you’ll have a solid grasp of how to perform matrix vector multiplication using NumPy, enhancing your data manipulation skills in Python.
Understanding Matrix Vector Multiplication
Before diving into the methods, let’s briefly discuss what matrix vector multiplication entails. In mathematical terms, multiplying a matrix by a vector involves taking the dot product of each row of the matrix with the vector. The result is a new vector whose length is equal to the number of rows in the matrix. This operation is crucial in various fields, including machine learning, physics, and engineering, where linear transformations are common.
Using numpy.matmul() Method
The numpy.matmul()
method is a straightforward way to perform matrix vector multiplication in Python. This function is designed specifically for matrix operations, making it more intuitive for users familiar with linear algebra.
Here’s how you can use numpy.matmul()
for matrix vector multiplication:
import numpy as np
matrix = np.array([[1, 2, 3],
[4, 5, 6],
[7, 8, 9]])
vector = np.array([1, 2, 3])
result = np.matmul(matrix, vector)
print(result)
Output:
[14 32 50]
In this example, we first import the NumPy library and define a 3x3 matrix and a vector of size 3. The np.matmul()
function takes these two inputs and performs the multiplication. The result is a new vector, where each element is the sum of the products of the corresponding row in the matrix and the vector. Specifically, the first element (14) is calculated as (11 + 22 + 33), the second element (32) as (41 + 52 + 63), and so on. This method is particularly useful when dealing with higher-dimensional arrays and is optimized for performance.
Using numpy.dot() Method
Another commonly used method for matrix vector multiplication in NumPy is the numpy.dot()
function. While it can also perform other operations, its use for matrix multiplication is well-known and widely adopted.
Here’s how to use numpy.dot()
for the same example:
import numpy as np
matrix = np.array([[1, 2, 3],
[4, 5, 6],
[7, 8, 9]])
vector = np.array([1, 2, 3])
result = np.dot(matrix, vector)
print(result)
Output:
[14 32 50]
As you can see, the code structure is almost identical to the previous example. After importing NumPy and defining the matrix and vector, we use the np.dot()
function to perform the multiplication. The output remains the same, yielding a new vector that results from the dot product of the matrix and the vector. While numpy.dot()
is versatile and can handle various types of operations, it is essential to note that it may not be as intuitive as numpy.matmul()
for users focused solely on matrix operations.
Conclusion
In summary, both numpy.matmul()
and numpy.dot()
are effective methods for performing matrix vector multiplication in Python. While numpy.matmul()
is tailored for matrix operations and is often the preferred choice for clarity, numpy.dot()
offers versatility for various mathematical computations. Understanding these methods will enable you to leverage NumPy’s capabilities fully, enhancing your data analysis and numerical computing skills. Whether you’re working on machine learning algorithms or scientific computations, mastering these functions is a valuable asset in your Python toolkit.
FAQ
-
What is the difference between numpy.matmul() and numpy.dot()?
numpy.matmul() is specifically designed for matrix operations, while numpy.dot() can perform various mathematical operations, including inner and outer products. -
Can I multiply a matrix by a scalar using these methods?
No, both numpy.matmul() and numpy.dot() are intended for matrix and vector operations. To multiply a matrix by a scalar, you can simply use the multiplication operator (*). -
What happens if the dimensions of the matrix and vector do not align?
If the dimensions do not align, NumPy will raise a ValueError indicating that the shapes are not aligned for matrix multiplication. -
Are there any performance differences between the two methods?
Generally, numpy.matmul() may be slightly faster for matrix operations since it is optimized for that purpose, but the difference is often negligible for small matrices.
- Can these methods handle multi-dimensional arrays?
Yes, both numpy.matmul() and numpy.dot() can handle multi-dimensional arrays, but the behavior may vary depending on the dimensions of the arrays involved.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn