NumPy Matrix Subtraction
- Understanding Matrix Subtraction in NumPy
- Method 1: Using the Infix Operator
- Method 2: Using the NumPy Subtract Function
- Method 3: Subtracting Matrices with Broadcasting
- Conclusion
- FAQ
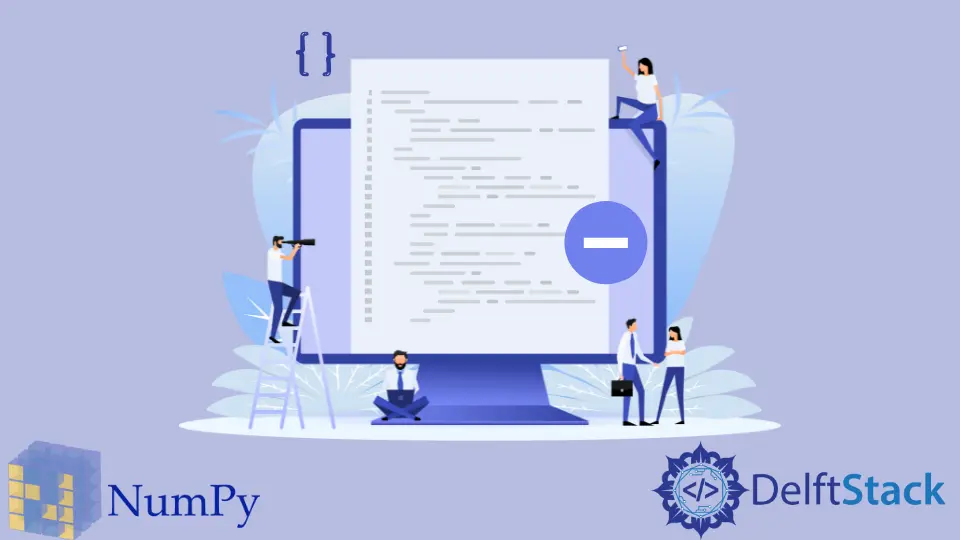
Matrix operations are a fundamental part of data manipulation in Python, especially when using the NumPy library. One of the most common operations you’ll encounter is matrix subtraction. In NumPy, we can easily subtract one matrix from another using the infix subtraction operator (-
). This operation is not only straightforward but also efficient, making it a popular choice for data scientists and engineers alike.
In this article, we will explore various methods to perform matrix subtraction in NumPy, complete with code examples and detailed explanations. Whether you’re a beginner or looking to refine your skills, this guide will help you master matrix subtraction in no time.
Understanding Matrix Subtraction in NumPy
Before diving into the methods of matrix subtraction, it’s essential to understand the basics of matrices in NumPy. A matrix is essentially a two-dimensional array, and NumPy provides a robust framework for handling these structures. The subtraction of two matrices involves subtracting corresponding elements from each matrix. For this operation to be valid, both matrices must have the same dimensions.
Let’s explore the various ways to perform matrix subtraction in NumPy, starting with the most straightforward approach using the infix operator.
Method 1: Using the Infix Operator
The simplest way to subtract two matrices in NumPy is by using the infix subtraction operator (-
). This method is intuitive and allows for clear, readable code. Here’s how you can do it:
import numpy as np
A = np.array([[5, 7, 9], [3, 6, 8]])
B = np.array([[2, 4, 6], [1, 3, 5]])
C = A - B
print(C)
Output:
[[3 3 3]
[2 3 3]]
In this example, we first import the NumPy library and create two matrices, A
and B
. The subtraction operation A - B
is performed element-wise. This means that each element in matrix A
is subtracted from the corresponding element in matrix B
. The result is stored in matrix C
, which is then printed to the console. This method is not only efficient but also very readable, making it a favorite among programmers.
Method 2: Using the NumPy Subtract Function
Another way to perform matrix subtraction in NumPy is by using the np.subtract()
function. This method is particularly useful when you want to specify additional parameters or when you prefer functional programming styles. Here’s how it works:
import numpy as np
A = np.array([[10, 20, 30], [40, 50, 60]])
B = np.array([[1, 2, 3], [4, 5, 6]])
C = np.subtract(A, B)
print(C)
Output:
[[ 9 18 27]
[36 45 54]]
In this example, we again create two matrices, A
and B
. Instead of using the infix operator, we call np.subtract(A, B)
. This function takes two arrays as input and performs the same element-wise subtraction. The result is stored in C
, which we then print. The advantage of using np.subtract()
is that it can be more flexible in certain contexts, such as when you’re working with higher-dimensional arrays or need to specify an output array.
Method 3: Subtracting Matrices with Broadcasting
NumPy also supports a powerful feature called broadcasting, which allows you to perform operations on arrays of different shapes. This can be particularly useful when you want to subtract a vector from a matrix. Here’s an example of how broadcasting works in matrix subtraction:
import numpy as np
A = np.array([[10, 20, 30], [40, 50, 60]])
B = np.array([1, 2, 3])
C = A - B
print(C)
Output:
[[ 9 18 27]
[39 48 57]]
In this case, we have a 2D matrix A
and a 1D array B
. When we perform the subtraction A - B
, NumPy automatically broadcasts the 1D array B
across the rows of matrix A
. This means that each element of B
is subtracted from the corresponding column of A
. The result is a new matrix C
, where the subtraction has been applied across all rows. Broadcasting simplifies code and enhances performance by allowing you to avoid explicit loops.
Conclusion
Matrix subtraction in NumPy is a straightforward yet powerful operation that can significantly enhance your data manipulation capabilities. Whether you prefer using the infix operator, the np.subtract()
function, or leveraging broadcasting, NumPy provides flexible options to suit your coding style. Understanding these methods not only makes your coding more efficient but also helps you write cleaner and more readable code. As you continue to explore NumPy, you’ll find that mastering these basic operations lays the groundwork for tackling more complex data analysis tasks.
FAQ
- how do I ensure matrices have the same shape for subtraction?
You can check the shape of your matrices using the.shape
attribute in NumPy. Ensure both matrices have the same dimensions before performing subtraction.
-
can I subtract matrices of different dimensions?
No, matrix subtraction requires both matrices to have the same dimensions. However, you can use broadcasting to subtract a vector from a matrix. -
what happens if I try to subtract matrices of different shapes?
If you attempt to subtract matrices of different shapes without broadcasting, NumPy will raise a ValueError indicating that the shapes are not aligned. -
is there a performance difference between using the infix operator and np.subtract?
Generally, both methods are efficient, but the infix operator tends to be more readable and is often preferred for simple operations. -
can I subtract more than two matrices at once?
Yes, you can chain subtraction operations together. For example, you can doA - B - C
to subtract multiple matrices in one line.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn