How to Append to Empty Array in NumPy
-
Append New Rows to an Empty NumPy Array in Python Using the
np.append()
-
Append New Rows to an Empty NumPy Array in Python Using the
np.vstack()
-
Append New Rows to an Empty NumPy Array in Python Using List Conversion and
np.array()
- Append New Rows to an Empty NumPy Array in Python Using a Loop to Add Multiple Rows
- Append New Rows to an Empty NumPy Array in Python Using a List to Store Rows and Then Converting to a NumPy Array
- Conclusion
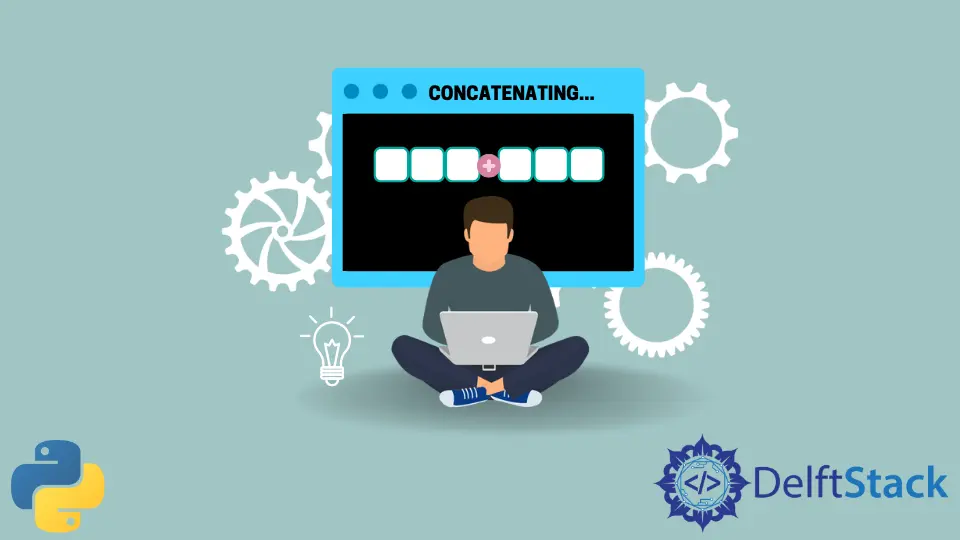
NumPy is a powerful library for numerical computations in Python, and it provides several methods to work with arrays.
In this article, we’ll explore various methods to append new rows to an empty NumPy array. You’ll find detailed explanations and code examples for each method, allowing you to choose the one that suits your specific needs.
Append New Rows to an Empty NumPy Array in Python Using the np.append()
In Python, the NumPy library offers a powerful tool for manipulating arrays, and one essential function is np.append()
. This versatile function allows you to add new rows to an existing NumPy array along a specified axis, making it a valuable tool for a wide range of data manipulation tasks.
The np.append()
function has the following syntax:
numpy.append(arr, values, axis=None)
Here is a breakdown of the parameters:
arr
: This parameter represents the input array to which new rows will be appended. It’s essentially the array to which you want to add more data.values
: This parameter denotes the values or rows that you want to append to the input array. These can be in the form of a single array, a list of arrays, or even scalar values.axis
: Theaxis
parameter specifies along which axis the new rows should be appended. If this parameter is not explicitly specified, NumPy will attempt to infer the axis automatically.
Let’s walk through a simple example to demonstrate how to use np.append()
:
import numpy as np
empty_array = np.array([]) # Create an empty array
new_row = np.array([1, 2, 3]) # Create a new row
updated_array = np.append(empty_array, new_row, axis=0)
print(updated_array)
Running the code example above will produce the following output:
[1. 2. 3.]
Here, we begin by importing the NumPy library and aliasing it as np
to make it more concise and accessible in our code.
We then create an empty NumPy array named empty_array
. This is our starting point and the array to which we want to add new data.
Next, we create a new row using the np.array()
function, defining the row’s content as [1, 2, 3]
. This is the data that we want to append to the empty_array
.
To append the new row to the empty_array
, we use the np.append()
function, passing in the empty_array
as the first argument, the new_row
as the second argument, and axis=0
to indicate that the new row should be added along the first axis. In a 2D array, the first axis is typically the row axis.
The resulting array, which now contains the newly appended row, is stored in the variable updated_array
.
Finally, we print updated_array
, and the output shows that the new_row
has been successfully added to the empty_array
.
Append New Rows to an Empty NumPy Array in Python Using the np.vstack()
When it comes to handling NumPy arrays in Python, the np.vstack()
function is a powerful tool specifically designed for vertically stacking arrays. This makes it an excellent choice for appending new rows to an empty NumPy array.
The np.vstack()
function has a straightforward syntax:
numpy.vstack(tup)
Parameter:
tup
: This parameter expects a tuple of arrays that you want to stack vertically. These arrays can be NumPy arrays, lists of arrays, or a combination of both.
To demonstrate how to use np.vstack()
to append new rows to an empty NumPy array, we’ll walk through a practical code example:
import numpy as np
empty_array = np.empty((0, 3)) # Create an empty array
new_row = np.array([1, 2, 3]) # Create a new row
updated_array = np.vstack((empty_array, new_row))
print(updated_array)
Running the code example will yield the following output:
[[1. 2. 3.]]
We begin by importing the NumPy library and aliasing it as np
, a common convention to make code more concise and readable.
Then, we create an empty NumPy array called empty_array
. This array serves as our starting point, and our objective is to add new data to it.
Next, we create a new row using the np.array()
function. This new row is defined as [1, 2, 3]
, and it’s the data we want to append to the empty_array
.
To accomplish this, we use the np.vstack()
function. We provide the empty_array
as the first element of a tuple and the new_row
as the second element.
The function stacks these arrays vertically, which means it combines them in a way that adds the new row on top of the existing empty array. The result of the vertical stacking operation is stored in the variable updated_array
.
Finally, we print the updated_array
, and the output demonstrates that the new_row
has been successfully appended to the empty_array
.
Append New Rows to an Empty NumPy Array in Python Using List Conversion and np.array()
Appending new rows to an empty NumPy array can also be accomplished using a straightforward approach that involves creating an empty list to temporarily store rows and then converting that list into a NumPy array. While this method doesn’t rely on a specific NumPy function for appending, it’s an effective and easy-to-understand way of achieving the desired result.
Unlike the previous methods that use dedicated NumPy functions like np.append()
and np.vstack()
, this approach doesn’t have a specific syntax tied to a NumPy function. Instead, it relies on Python’s native list operations and NumPy’s np.array()
function for array conversion.
Let’s go through a practical code example to illustrate how to use list conversion and np.array()
to append new rows to an empty NumPy array:
import numpy as np
empty_array = np.array([]) # Create an empty array
new_row = np.array([1, 2, 3]) # Create a new row
updated_array = np.array(list(empty_array) + [new_row])
print(updated_array)
When you run the code example provided above, you’ll see the following output:
[[1 2 3]]
As you can see, we start by importing the NumPy library and aliasing it as np
, a common practice for improved code readability.
An empty NumPy array, named empty_array
, is created. This array serves as our starting point, and we intend to add new data to it.
We create a new row using the np.array()
function, defining its content as [1, 2, 3]
. This new row represents the data we want to append to the empty_array
.
To append the new row to the empty_array
, we take advantage of Python’s list operations.
We temporarily store the rows in a Python list using list(empty_array)
and then add the new row to this list using the +
operator. This operation combines the elements of the list, effectively appending the new row.
Finally, to transform the combined list back into a NumPy array, we use the np.array()
function. The result is stored in the variable updated_array
.
We print the updated_array
, which demonstrates that the new row has been successfully appended to the empty_array
.
Append New Rows to an Empty NumPy Array in Python Using a Loop to Add Multiple Rows
Appending new rows to an empty NumPy array becomes even more practical when you need to add multiple rows. In such cases, a loop can be a powerful tool to iteratively add each row to the empty NumPy array.
This approach allows you to efficiently expand your array with multiple rows while maintaining code flexibility.
Similar to the previous method that utilized list conversion and np.array()
, this approach doesn’t rely on a specific NumPy function for appending rows. Instead, it combines native Python loop constructs and NumPy’s np.append()
function for array manipulation.
Let’s dive into a practical code example to demonstrate how to use a loop to append new rows to an empty NumPy array:
import numpy as np
empty_array = np.array([]) # Create an empty array
new_rows = [np.array([1, 2, 3]), np.array([4, 5, 6])] # Create new rows
for row in new_rows:
empty_array = np.append(empty_array, row, axis=0)
print(empty_array)
When you run the provided code example, you’ll observe the following output:
[1. 2. 3. 4. 5. 6.]
In the code example, we initiate the process by importing the NumPy library and aliasing it as np
, a common practice to enhance code readability.
Then, an empty NumPy array, denoted as empty_array
, is created. This array acts as our starting point for adding new data.
Next, we define a list of new rows named new_rows
. Each row within this list is represented as a NumPy array. In this example, we have two new rows: [1, 2, 3]
and [4, 5, 6]
.
To append these new rows to the empty_array
, we utilize a for
loop. This loop iterates through the list of new rows.
Inside the loop, we employ the np.append()
function. It appends each row to the empty_array
along the first axis (specified as axis=0
). This step effectively adds each row to the existing array in sequence.
Upon completion of the loop, the empty_array
contains all the appended rows, and we print this updated array.
Append New Rows to an Empty NumPy Array in Python Using a List to Store Rows and Then Converting to a NumPy Array
Appending new rows to an empty NumPy array can also be efficiently achieved by utilizing a Python list to temporarily store the rows and subsequently converting this list into a NumPy array once all rows have been collected.
This method provides an organized and effective way to accumulate and append data, which is especially useful when you have a set of rows to add and want to streamline the process.
Unlike the previous methods that rely on specific NumPy functions like np.append()
and np.vstack()
, this approach doesn’t have a specific NumPy function associated with it. Instead, it leverages Python’s list operations and NumPy’s np.array()
function for array conversion.
Let’s have a practical code example to demonstrate how to use a list to store rows and then convert them into a NumPy array:
import numpy as np
rows_list = [] # Create an empty list to store rows
new_rows = [np.array([1, 2, 3]), np.array([4, 5, 6])] # Create new rows
rows_list.extend(new_rows)
updated_array = np.array(rows_list)
print(updated_array)
When you execute the provided code example, you will see the following output:
[[1 2 3]
[4 5 6]]
We initiate the process by importing the NumPy library and aliasing it as np
, a common practice to enhance code readability.
To store the rows temporarily, we create an empty Python list named rows_list
. This list serves as a container to accumulate the rows before converting them into a NumPy array.
We then define a list of new rows named new_rows
. Each row within this list is represented as a NumPy array. In this example, we have two new rows: [1, 2, 3]
and [4, 5, 6]
.
To add all the rows from new_rows
to the rows_list
, we use the extend()
method of the list. This operation efficiently appends all elements from new_rows
to rows_list
.
Finally, to transform the rows_list
into a NumPy array, we employ the np.array()
function. The result is stored in the variable updated_array
.
We print the updated_array
, which displays that the new rows have been successfully appended to the empty_array
.
Conclusion
Appending new rows to an empty NumPy array in Python can be achieved through various methods, each with its advantages. The choice of method depends on the specific requirements of your task.
You can use np.append()
or np.vstack()
for a single row, list conversion for a simple approach, and loops or list storage for scenarios involving multiple rows. These methods provide flexibility and efficiency, allowing you to work with NumPy arrays effectively in your Python projects.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn