How to Shift Array in MATLAB
-
Shift an Array Using the
circshift()
Function in MATLAB - Shift an Array Using the Array Indices in MATLAB
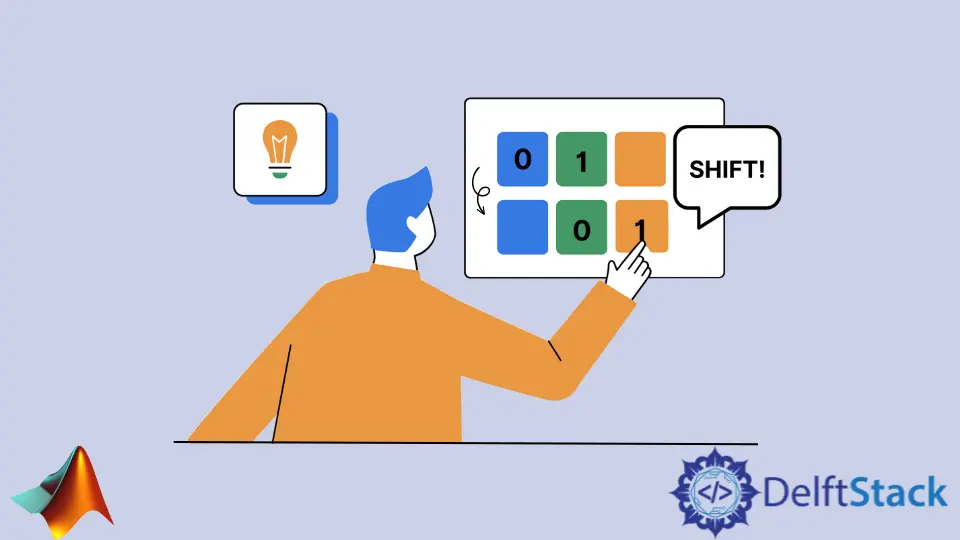
This tutorial will introduce how to shift an array manually and using the circshift()
function in MATLAB.
Shift an Array Using the circshift()
Function in MATLAB
If you want to shift an array to the left or right by a specific number of places, you can use the circshift()
function, which shifts the given array circularly by a specific number of places. The first argument of this function is the array you want to shift, and the second is the number of places you want to shift, which can either be the number of columns or the number of rows. If the second argument is a negative number, then the array will be shifter left otherwise right. For example, let’s define an array of 1 to 10 integers and shift it left using the circshift()
function. See the code below.
myArray = 1:10
shifted_array = circshift(myArray,[1,-3])
Output:
myArray =
1 2 3 4 5 6 7 8 9 10
shifted_array =
4 5 6 7 8 9 10 1 2 3
The second argument specifies that we want to left-shift the first row by three places in the above code. You can also shift columns if you have a matrix.
Shift an Array Using the Array Indices in MATLAB
If you want to shift an array to the left or right by a specific number of places and a new element somewhere in the array, you can use the array indices. For example, let’s define an array of 1 to 10 integers and shift it left using the array indices. See the code below.
myArray = 1:10
shifted_array = [myArray(4:end) myArray(1:3)]
Output:
myArray =
1 2 3 4 5 6 7 8 9 10
shifted_array =
4 5 6 7 8 9 10 1 2 3
In the above code, end
is used to specify the end of the array. You can also shift columns if you have a matrix. Now, let’s shift the array by one place to the left and add a new element at the end of the array and delete the first element. See the code below.
myArray = 1:10
element = 11
shifted_array = [myArray(2:end) element]