How to Add Class to Element in JavaScript
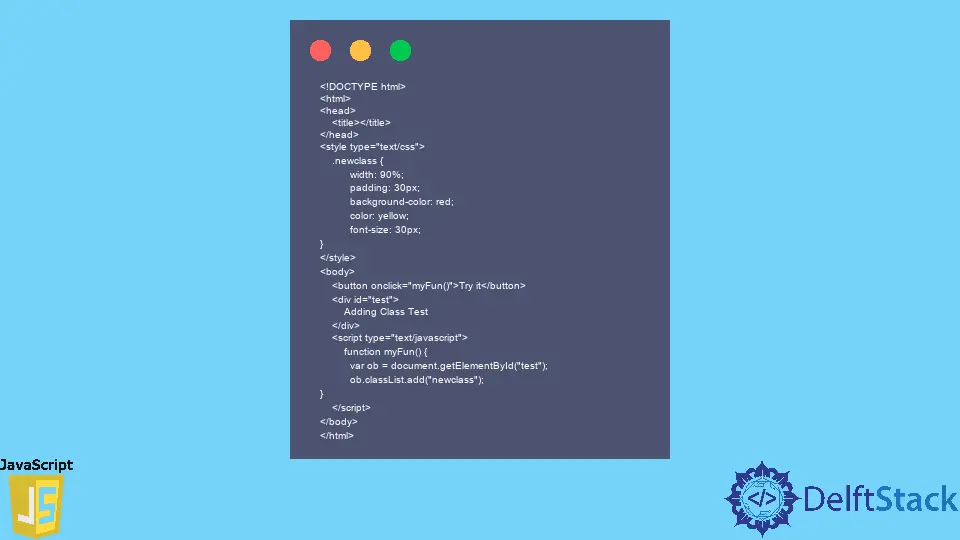
This tutorial will discuss adding a class to a given element using the classList
property in JavaScript.
Add a Class to a Given Element Using the classList
Property in JavaScript
If you want to add a class to a given element in JavaScript, you can use the classList
property. First, you have to get the given element, and the easiest way to do that is to get it by using its id
. Suppose an id
is not given to the element; in that case, you can give it a unique id
and get the element using the getElementById
property in JavaScript and store it in a variable. After that, you can use the variable to change its property using the classList
property. For example, let’s change the class of a text using a button. See the code below.
<!DOCTYPE html>
<html>
<head>
<title></title>
</head>
<style type="text/css">
.newclass {
width: 90%;
padding: 30px;
background-color: red;
color: yellow;
font-size: 30px;
}
</style>
<body>
<button onclick="myFun()">Try it</button>
<div id="test">
Adding Class Test
</div>
<script type="text/javascript">
function myFun() {
var ob = document.getElementById("test");
ob.classList.add("newclass");
}
</script>
</body>
</html>
In the above code, we have a style tag in which we are setting properties of a class object; inside the body tag, we have a button and some text, and inside the script tag, we are changing the class of the text using its id
. In this example, the style sheet and JavaScript code is placed inside the same HTML file, but you can also put them in separate files and link them to the HTML file. To run the above code, you need to copy the code into an HTML file and open it using a browser.
Output:
As you can see in the output, there is a button and some text. When you press this button, the class of the text will be changed.
Output when button is pressed:
As you can see in the output, the text shape is changed because of the change in class. In this example, we are using a button to change the class of an element, but you can change the condition on which you want to change the class of a given element. You cannot use the classList
property in internet explorer 9. Instead, you can use the +=
operator to add the property to a given element. You have to change the last line of the myFun
function inside the script tag with the below line.
ob.className += " newclass";
You have to use a space before the name of the class because maybe there are other classes present.