How to Create Table Using JavaScript
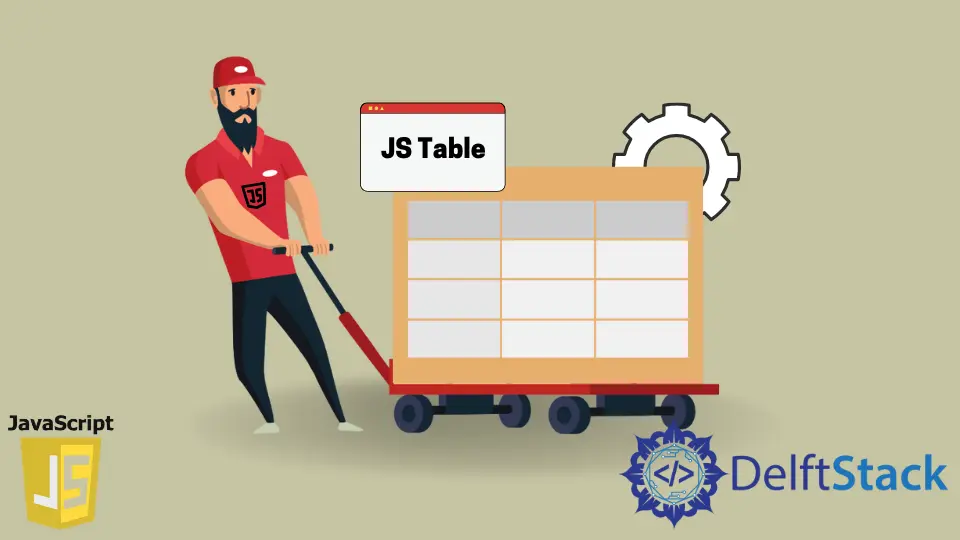
The JavaScript programming language allows us to create and manipulate DOM (Document Object Model) elements. It gives us much more flexibility and makes it a lot easier for everyone to work with DOM, especially if you are a backend developer.
There are two ways of adding HTML elements like tables to an HTML document, the first is by adding the HTML table tag directly into our HTML webpage, and the second way is by creating the entire table inside our JavaScript code. The second option is the most popular way of creating and adding the table to the DOM.
But before diving deep into how the table is created with JavaScript, let’s first visually understand the various table tags used to create a table and what each of those tags exactly means. This will give you a visual representation of the table, making it much easier for you to understand the code part later in this article.
Various Tags Used to Create a Table in HTML
Below is the list of tags that are used to create a table in HTML.
table
: Thetable
HTML tag represents the entire table in HTML. This is the starting tag of the table inside which all the other tags related to the table (likethead
,tr
,td
, etc) will be used depending upon how you want to structure the table.thead
: Thethead
stands for table heading. This tag is used to add heading to the tables. It usually represents the first row of the table. To add data inside thethead
tag, we use theth
tag. If you don’t want to add a heading to your tables, you can skip thethead
tag.tbody
: Thetbody
stands for table body. This tag represents the entire body or contents of the table.tfoot
: Thetfoot
stands for table footer. It usually represents the last row of the table. This tag is optional. We don’t use this tag that often.tr
: Thetr
stands for table row. This represents the entire row of the table. To insert data into the table either inside the heading, body, or footer of the table, we first have to create a row, and then inside that row, we can insert the data with the help of thetd
tag.th
: Theth
is only used inside thethead
tag. It represents the single cell of the heading row. It makes the headings of the table in bold format.td
: Thetd
stands for table data. It represents the single cell of the table. It is similar toth
, but the only difference is thatth
is used only inside thethead
tag, andtd
is used elsewhere. Thetd
tag can be used with thetbody
andtfoot
tags.
If you combine all of these tags in nested form i.e, one inside another, then this is how the table will look like after it is created.
The red border represents the entire table. Inside this, we have 3 tags, table header (thead
), table body (tbody
), and table footer (tfoot
), which are represented with the green border. To insert data inside these three tags we first have to create a row with the help of the tr
tag. This row is represented with the yellow border. And then, to insert data into the table header, we use the th
tag, and to insert data within the table body or table footer, we use the td
tags. It is shown with the help of grey color.
Create a Table Using JavaScript
To create an HTML element using JavaScript we have to use a method called document.createElement()
that takes tag name which is a string as a parameter. For instance, we want to create a table, so we will pass the string table
as an input to the createElement()
method document.createElement('table')
.
Let’s now create the below table entirely using JavaScript.
Sr. No. | Name | Company |
---|---|---|
1. | James Clerk | Netflix |
2. | Adam White | Microsoft |
The above table has 4 rows. The first row contains all the heading, and the next three rows contain the data.
Below we have the HTML, CSS, and JS code. Inside our body
tag, we initially don’t have anything. We will create our table inside the JavaScript, and at the end, we will append the entire table to the body
tag so that it will be visible on the webpage. Inside the style
tag, we have given some basic styling to our table.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
table{
border-collapse: collapse;
border-spacing: 0;
}
th, td{
padding: 10px 20px;
border: 1px solid #000;
}
</style>
</head>
<body id="body">
<script src="script.js"></script>
</body>
</html>
To create a table, we will first create the table
tag. Then we will store this tag into a variable table
so that later we can use it. In the same way, we will also create thead
and tbody
tags and store them inside the variables. Then we append thead
and tbody
to the table
tag.
let table = document.createElement('table');
let thead = document.createElement('thead');
let tbody = document.createElement('tbody');
table.appendChild(thead);
table.appendChild(tbody);
// Adding the entire table to the body tag
document.getElementById('body').appendChild(table);
You have to append or add that table that you have created in JavaScript to the body
tag. We first have to get the HTML body tag using its id
, and then we will append the table using something called the appendChild()
method. Since we already have stored our table inside the table
variable, we will just have to append the table we created as a child to this body element.
At this point, this is how the structure of our table looks like.
To add rows and data to our table, we will create table rows (tr
), table heading (th
), and table data (td
) tags using the createElement()
method, and then we will add the data inside those elements with the help of the innerHTML
property.
// Creating and adding data to first row of the table
let row_1 = document.createElement('tr');
let heading_1 = document.createElement('th');
heading_1.innerHTML = 'Sr. No.';
let heading_2 = document.createElement('th');
heading_2.innerHTML = 'Name';
let heading_3 = document.createElement('th');
heading_3.innerHTML = 'Company';
row_1.appendChild(heading_1);
row_1.appendChild(heading_2);
row_1.appendChild(heading_3);
thead.appendChild(row_1);
// Creating and adding data to second row of the table
let row_2 = document.createElement('tr');
let row_2_data_1 = document.createElement('td');
row_2_data_1.innerHTML = '1.';
let row_2_data_2 = document.createElement('td');
row_2_data_2.innerHTML = 'James Clerk';
let row_2_data_3 = document.createElement('td');
row_2_data_3.innerHTML = 'Netflix';
row_2.appendChild(row_2_data_1);
row_2.appendChild(row_2_data_2);
row_2.appendChild(row_2_data_3);
tbody.appendChild(row_2);
// Creating and adding data to third row of the table
let row_3 = document.createElement('tr');
let row_3_data_1 = document.createElement('td');
row_3_data_1.innerHTML = '2.';
let row_3_data_2 = document.createElement('td');
row_3_data_2.innerHTML = 'Adam White';
let row_3_data_3 = document.createElement('td');
row_3_data_3.innerHTML = 'Microsoft';
row_3.appendChild(row_3_data_1);
row_3.appendChild(row_3_data_2);
row_3.appendChild(row_3_data_3);
tbody.appendChild(row_3);
After inserting the data using the innerHTML
property, we will append that data to their respective rows. For example, first, we are adding the headings (i.e heading_1
, heading_2
, heading_3
) to row_1
. And then we are appending our first row, i.e., row_1
, to the thead
tag as a child.
Similarly, we are creating another 2 rows i.e row_2
and row_3
and then appending (row_2_data_1
, row_2_data_2
, row_2_data_3
) and (row_3_data_1
, row_3_data_2
, row_3_data_3
) variables respectively to these 2 rows. And at the end, we are appending these 2 rows to the tbody
tag as it’s children’s.
Now, this is how the table and its HTML structure look like in the developer’s console.
At the left, we have the table, and at the right, we have the browser-generated code. You can customize the js code based on how you want the table to look. We have only used 3 JavaScript methods (createElement()
, innerHTML
, appendChild()
) in order to achieve this.
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
LinkedIn