How to Draw a Line in Java
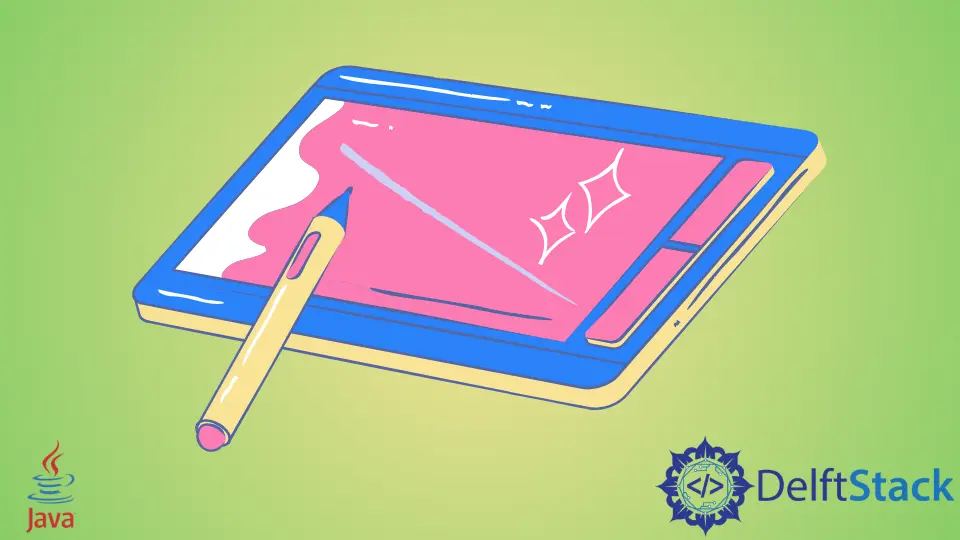
The Java.awt.Graphics
class in Java forms the base for many such drawing and graphics functions. It is an incomprehensible class, as the actual drawing action depends on the system and depends on the device. We will draw a line in Java in this tutorial.
We will start the program by importing the necessary packages. We will import the java.applet.Applet
, java.awt
and java.awt.event
package from the library.
The drawLine()
method of the Graphics class is used to draw a line with the given color between two points.
See the code below.
import java.applet.Applet;
import java.awt.*;
import java.awt.event.*;
public class DrawLine extends Applet {
public static void main(String[] args) {
Frame drawLineApplet = new Frame("Draw Line in Applet Window");
drawLineApplet.setSize(500, 450);
Applet DrawLine = new DrawLine();
drawLineApplet.add(DrawLine);
drawLineApplet.setVisible(true);
drawLineApplet.addWindowListener(new WindowAdapter() {
public void windowClosing(WindowEvent e) {
System.exit(0);
}
});
}
public void paint(Graphics g) {
g.setFont(new Font("Arial", Font.BOLD, 12));
g.drawString("This is Draw Line Example", 100, 70);
g.setColor(Color.blue);
g.drawLine(90, 135, 90, 180);
g.setColor(Color.green);
g.drawLine(60, 4, 120, 120);
}
}
In the above example, we created two lines and also displayed some text. We first declared a DrawLine
class, which extends the Applet
class (Parent class). Inside the class, we declared the main method. Here the Frame drawLineApplet = new Frame()
statement creates the applet window for the output.
The drawLineApplet.setSize()
function is used to set the size of the applet window, and the drawLineApplet.setVisible(true)
function is used to make the frame appear on the screen. We use the system.exit(0)
command to exit the applet frame.
The paint
method here is used to set the color, font, and coordinates o the line to be drawn. We change the font using the setFont()
function. The drawString()
function here displays some text on the output frame. We alter the color of the first line using setColor()
and then the x and y coordinates of the line in the drawLine()
function. Similarly, we provide the coordinates and color for the second line.