How to Merge Two Dictionaries in Python 2 and 3
- Merge Dictionaries in Python 2.7
- Python 3.5 (And Above) Dictionary Merge Method
- Merge Methods Conclusion
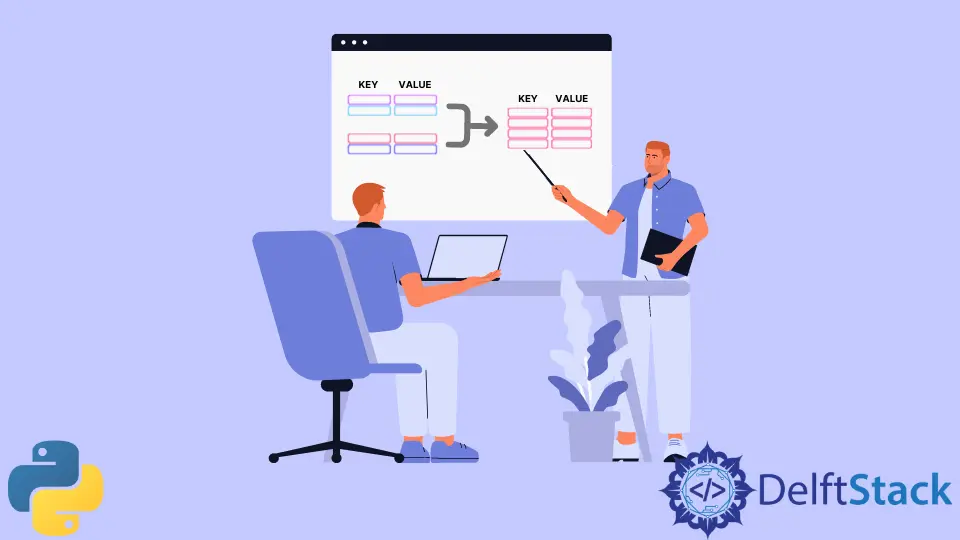
Suppose we have two Python dictionaries A
and B
to be merged, where the values in B
will replace those values in A
if they share the same key
.
A = {"x": 10, "y": 20}
B = {"y": 30, "z": 40}
Python dictionary
object has an intrinsic method update()
method to update dictionary A
with B
,
A.update(B)
But the data of A
will be replaced in-place instead of returning a new dictionary containing merge A
and B
.
We will introduce the methods of how to merge two dictionaries and return a new dictionary.
Merge Dictionaries in Python 2.7
Python Dictionary Comprehension Method - 1
C = {key: value for d in (A, B) for key, value in d.items()}
d.itmes()
returns a list of (key, value)
pairs as 2-tuples of dictionary d
.
This method uses the nested dictionary comprehension to merge two dictionaries. The right order of for
should be paid extra attention. It should be,
flattern_patterns = [planet for sublist in planets for planet in sublist]
Python Dictionary Comprehension Method - 2
We could also use the dict()
method to initialize the new dictionary.
C = dict((key, value) for d in (A, B) for key, value in d.items())
Technically speaking, it is almost the same as the above method but differs in the performance mentioned afterward.
itertools.chain
Method
The itertools
module standardizes a core set of iterator
building blocks. It has features like being fast and memory efficiency.
itertools.chain
returns a chain object whose .next()
method returns elements from the first iterable until it is exhausted, then the next iterable(s), until all are exhausted.
dict(itertools.chain(A.iteritems(), B.iteritems()))
iteritems()
returns an iterator over the (key, value)
items of the dictionary.
Therefore, the scripts above will return a dictionary containing items of A and B.
copy
and update
Method
As mentioned at the beginning, update()
could merge A
and B
, but will replace the dictionary in-place. We could use copy()
method to make a copy of the dictionary A
.
m = A.copy()
C = m.update(B)
Merge Methods Performance Analysis and Comparison
# python 2.x
import timeit
A = {"x": 10, "y": 20}
B = {"y": 30, "z": 40}
SETUP_CODE = """
A = {'x': 10, 'y': 20}
B = {'y': 30, 'z': 40}
"""
TEST_CODE = """
{key: value for d in (A, B) for key, value in d.items()}
"""
print min(timeit.repeat(setup=SETUP_CODE, stmt=TEST_CODE, repeat=3, number=10000))
TEST_CODE = """
dict((key, value) for d in (A, B) for key, value in d.items())
"""
print min(timeit.repeat(setup=SETUP_CODE, stmt=TEST_CODE, repeat=3, number=10000))
TEST_CODE = """
dict(itertools.chain(A.iteritems(), B.iteritems()))
"""
print min(timeit.repeat(setup=SETUP_CODE, stmt=TEST_CODE, repeat=3, number=10000))
SETUP_CODE = """
def merge_dicts(a, b):
m = a.copy()
m.update(b)
return m
A = {'x': 10, 'y': 20}
B = {'y': 30, 'z': 40}
"""
TEST_CODE = """
merge_dicts(A, B)
"""
print min(timeit.repeat(setup=SETUP_CODE, stmt=TEST_CODE, repeat=3, number=10000))
The result is
0.0162378
0.029774
0.019975
0.0110059
Method | Performance | Rank |
---|---|---|
{key: value for d in (A, B) for key, value in d.items()} |
0.0162378 | 2 |
dict((key, value) for d in (A, B) for key, value in d.items()) |
0.029774 | 4 |
dict(itertools.chain(A.iteritems(), B.iteritems())) |
0.019975 | 3 |
merge_dicts(a, b) |
0.0110059 | 1 |
Python 3.5 (And Above) Dictionary Merge Method
From Python 3.5, besides the same methods as in Python 2.7, it has also **
unpacking operator of dictionary
, as introduced in PEP-448. It allows unpacking an arbitrary number of items.
d.iteritems()
becomes deprecated in Python 3. See PEP-469>>> C = {**A, **B}
>>> C
{'x': 10, 'y': 30, 'z': 40}
# python 2.x
import timeit
A = {"x": 10, "y": 20}
B = {"y": 30, "z": 40}
SETUP_CODE = """
A = {'x': 10, 'y': 20}
B = {'y': 30, 'z': 40}
"""
TEST_CODE = """
{**A, **B}
"""
print(min(timeit.repeat(setup=SETUP_CODE, stmt=TEST_CODE, repeat=3, number=10000)))
TEST_CODE = """
{key: value for d in (A, B) for key, value in d.items()}
"""
print(min(timeit.repeat(setup=SETUP_CODE, stmt=TEST_CODE, repeat=3, number=10000)))
TEST_CODE = """
dict((key, value) for d in (A, B) for key, value in d.items())
"""
print(min(timeit.repeat(setup=SETUP_CODE, stmt=TEST_CODE, repeat=3, number=10000)))
TEST_CODE = """
dict(itertools.chain(A.items(), B.items()))
"""
print(min(timeit.repeat(setup=SETUP_CODE, stmt=TEST_CODE, repeat=3, number=10000)))
SETUP_CODE = """
def merge_dicts(a, b):
m = a.copy()
m.update(b)
return m
A = {'x': 10, 'y': 20}
B = {'y': 30, 'z': 40}
"""
TEST_CODE = """
merge_dicts(A, B)
"""
print(min(timeit.repeat(setup=SETUP_CODE, stmt=TEST_CODE, repeat=3, number=10000)))
0.0017047999999999508
0.009127499999999955
0.0168952
0.01078009999999996
0.005767999999999995
Method | Performance | Rank |
---|---|---|
{**A, **B} |
0.0017047999999999508 | 1 |
{key: value for d in (A, B) for key, value in d.items()} |
0.009127499999999955 | 3 |
dict((key, value) for d in (A, B) for key, value in d.items()) |
0.0168952 | 5 |
dict(itertools.chain(A.items(), B.items())) |
0.01078009999999996 | 4 |
merge_dicts(a, b) |
0.005767999999999995 | 2 |
Merge Methods Conclusion
In Python 2.7, copy
and update
is the best method.
m = A.copy()
C = m.update(B)
In Python 3.5+, dictionary unpacking method is the best.
{**A, **B}
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook