How to Get the Current Time in Python
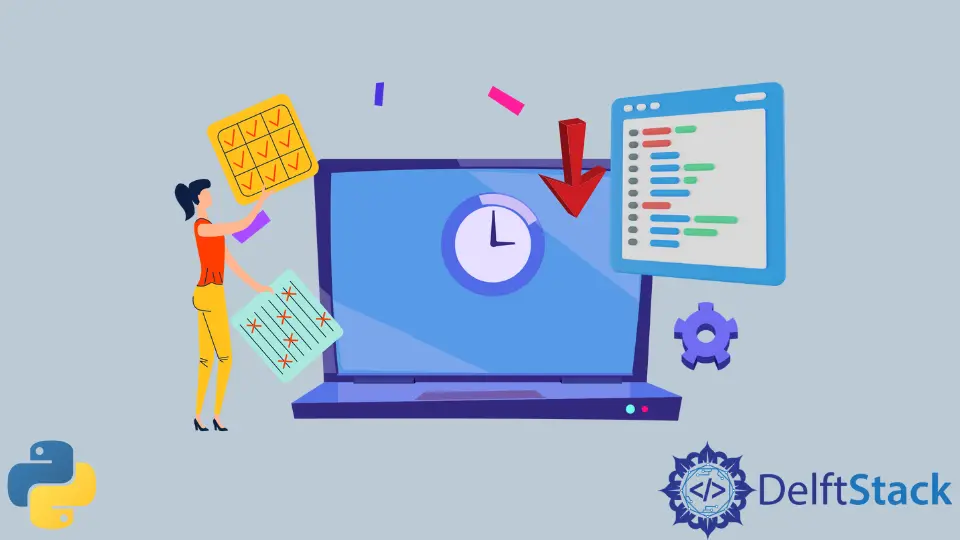
Getting the current time is a common task in programming, especially when you’re working on applications that require time-stamping, logging, or scheduling events. In Python, there are several straightforward methods to retrieve the current time. Whether you’re building a simple script or a complex application, knowing how to get the current time can enhance your project’s functionality.
In this article, we’ll explore various methods to obtain the current time in Python, complete with code examples and detailed explanations. By the end, you’ll have a solid understanding of how to work with time in Python.
Using the datetime Module
One of the most popular ways to get the current time in Python is by using the datetime
module. This built-in module provides classes for manipulating dates and times. To get the current time, you can use the datetime.now()
method. Here’s how it works:
from datetime import datetime
current_time = datetime.now()
print(current_time)
Output:
2023-10-01 14:30:45.123456
The datetime.now()
function returns the current local date and time as a datetime
object. This object contains various attributes, including year, month, day, hour, minute, second, and microsecond. You can easily format this output to display only the time or date as per your requirements. For example, if you want to display just the time, you can use the strftime
method to format it into a more readable string.
formatted_time = current_time.strftime("%H:%M:%S")
print(formatted_time)
Output:
14:30:45
The strftime
method allows you to specify the format you want. In this case, %H:%M:%S
gives you the hour, minute, and second. This flexibility makes the datetime
module a powerful tool for handling time in Python.
Using the time Module
Another way to get the current time is by using the time
module, which is also a built-in module in Python. While the datetime
module is more comprehensive, the time
module provides a simpler interface for dealing with time-related tasks. To get the current time using the time
module, you can use the time()
function, which returns the current time in seconds since the epoch (January 1, 1970). Here’s an example:
import time
current_time = time.time()
print(current_time)
Output:
1696173045.123456
The output is a floating-point number representing the number of seconds since the epoch. If you want to convert this into a more human-readable format, you can use the localtime()
and strftime()
functions from the time
module. Here’s how:
local_time = time.localtime(current_time)
formatted_time = time.strftime("%H:%M:%S", local_time)
print(formatted_time)
Output:
14:30:45
In this example, localtime()
converts the epoch time into a struct_time
object, which contains various time-related attributes. The strftime()
function then formats this into a string that displays only the time. This method is particularly useful if you need a quick way to get the current time without additional complexities.
Using the arrow Library
For those looking for a more intuitive way to work with dates and times, the arrow
library is an excellent third-party option. This library simplifies date and time manipulation and provides a more human-friendly interface. First, you’ll need to install the library if you haven’t done so already:
pip install arrow
Once you have the library installed, you can easily get the current time. Here’s how:
import arrow
current_time = arrow.now()
print(current_time)
Output:
2023-10-01T14:30:45.123456Z
The arrow.now()
function returns the current time as an Arrow
object, which is a more powerful representation of time compared to a standard datetime
object. You can format this output similarly to how you would with the datetime
module. For instance, if you want to display just the time, you can do the following:
formatted_time = current_time.format('HH:mm:ss')
print(formatted_time)
Output:
14:30:45
Using the arrow
library not only makes it easier to get the current time but also provides a range of functionalities for time zone handling, formatting, and arithmetic. This makes it a great choice for projects that require more advanced time manipulation.
Conclusion
In this article, we’ve explored several methods to get the current time in Python, including the built-in datetime
and time
modules, as well as the third-party arrow
library. Each method has its advantages, depending on your specific needs. Whether you prefer the simplicity of the time
module or the advanced features of the arrow
library, Python offers versatile options for working with time. Understanding these methods will not only enhance your programming skills but also empower you to build more robust applications.
FAQ
-
How do I install the arrow library?
You can install the arrow library using pip with the command pip install arrow. -
Can I get the current time in a specific timezone?
Yes, using the arrow library, you can easily get the current time in any timezone by using arrow.now(’timezone’). -
What is the difference between datetime and time modules?
The datetime module provides more comprehensive functionality for date and time manipulation, while the time module is simpler and focuses primarily on time-related tasks. -
How can I format the current time in a specific way?
You can use the strftime method from the datetime or time module, or the format method from the arrow library to customize the output format.
- Is it necessary to install third-party libraries for getting the current time?
No, you can use the built-in datetime and time modules in Python to get the current time without needing any additional libraries.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook