Pandas드롭 행
Suraj Joshi
2023년1월30일
Pandas
Pandas DataFrame Row
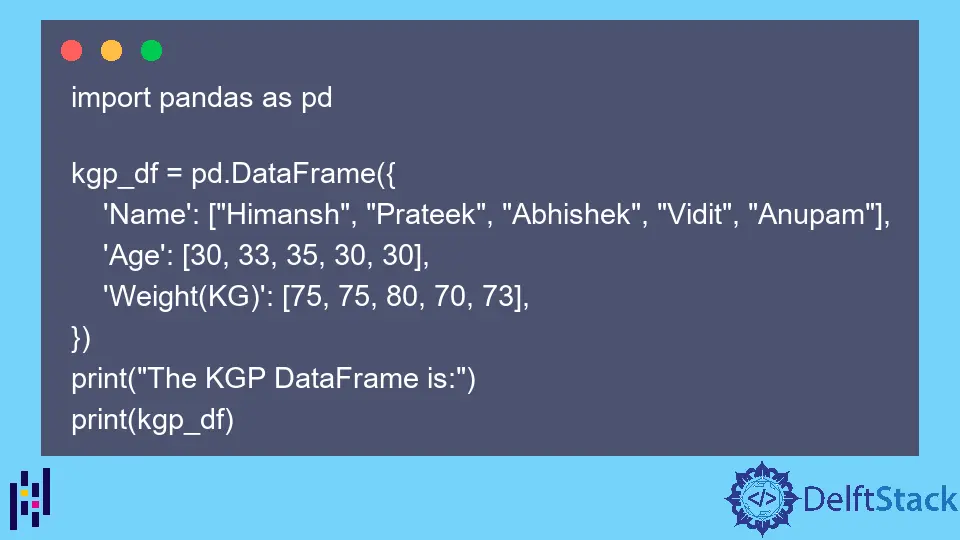
이 자습서에서는pandas.DataFrame.drop()
메서드를 사용하여 Pandas에서 행을 삭제하는 방법을 설명합니다.
import pandas as pd
kgp_df = pd.DataFrame(
{
"Name": ["Himansh", "Prateek", "Abhishek", "Vidit", "Anupam"],
"Age": [30, 33, 35, 30, 30],
"Weight(KG)": [75, 75, 80, 70, 73],
}
)
print("The KGP DataFrame is:")
print(kgp_df)
출력:
The KGP DataFrame is:
Name Age Weight(KG)
0 Himansh 30 75
1 Prateek 33 75
2 Abhishek 35 80
3 Vidit 30 70
4 Anupam 30 73
kgp_df
DataFrame을 사용하여 Pandas DataFrame에서 행을 삭제하는 방법을 설명합니다.
pandas.DataFrame.drop()
메서드에서 색인별로 행 삭제
import pandas as pd
kgp_df = pd.DataFrame(
{
"Name": ["Himansh", "Prateek", "Abhishek", "Vidit", "Anupam"],
"Age": [30, 33, 35, 30, 30],
"Weight(KG)": [75, 75, 80, 70, 73],
}
)
rows_dropped_df = kgp_df.drop(kgp_df.index[[0, 2]])
print("The KGP DataFrame is:")
print(kgp_df, "\n")
print("The KGP DataFrame after dropping 1st and 3rd DataFrame is:")
print(rows_dropped_df)
출력:
The KGP DataFrame is:
Name Age Weight(KG)
0 Himansh 30 75
1 Prateek 33 75
2 Abhishek 35 80
3 Vidit 30 70
4 Anupam 30 73
The KGP DataFrame after dropping 1st and 3rd DataFrame is:
Name Age Weight(KG)
1 Prateek 33 75
3 Vidit 30 70
4 Anupam 30 73
kgp_df
DataFrame에서 인덱스 0과 2가있는 행을 삭제합니다. 인덱스가 0에서 시작하기 때문에 인덱스가 0과 2 인 행은 DataFrame의 첫 번째와 세 번째 행에 해당합니다.
기본 색인을 사용하는 대신 DataFrame의 색인을 사용하여 행을 삭제할 수도 있습니다.
import pandas as pd
kgp_idx = ["A", "B", "C", "D", "E"]
kgp_df = pd.DataFrame(
{
"Name": ["Himansh", "Prateek", "Abhishek", "Vidit", "Anupam"],
"Age": [30, 33, 35, 30, 30],
"Weight(KG)": [75, 75, 80, 70, 73],
},
index=kgp_idx,
)
rows_dropped_df = kgp_df.drop(["A", "C"])
print("The KGP DataFrame is:")
print(kgp_df, "\n")
print("The KGP DataFrame after dropping 1st and 3rd DataFrame is:")
print(rows_dropped_df)
출력:
The KGP DataFrame is:
Name Age Weight(KG)
A Himansh 30 75
B Prateek 33 75
C Abhishek 35 80
D Vidit 30 70
E Anupam 30 73
The KGP DataFrame after dropping 1st and 3rd DataFrame is:
Name Age Weight(KG)
B Prateek 33 75
D Vidit 30 70
E Anupam 30 73
인덱스A
와C
가있는 행 또는 DataFrame에서 첫 번째와 세 번째 행을 삭제합니다.
삭제할 행의 인덱스 목록을drop()
메소드에 전달하여 각 행을 삭제합니다.
Pandas DataFrame의 특정 열 값에 따라 행 삭제
import pandas as pd
kgp_idx = ["A", "B", "C", "D", "E"]
kgp_df = pd.DataFrame(
{
"Name": ["Himansh", "Prateek", "Abhishek", "Vidit", "Anupam"],
"Age": [31, 33, 35, 36, 34],
"Weight(KG)": [75, 75, 80, 70, 73],
},
index=kgp_idx,
)
young_df_idx = kgp_df[kgp_df["Age"] <= 33].index
young_folks = kgp_df.drop(young_df_idx)
print("The KGP DataFrame is:")
print(kgp_df, "\n")
print("The DataFrame of folks with age less than or equal to 33 are:")
print(young_folks)
출력:
The KGP DataFrame is:
Name Age Weight(KG)
A Himansh 31 75
B Prateek 33 75
C Abhishek 35 80
D Vidit 36 70
E Anupam 34 73
The DataFrame of folks with age less than or equal to 33 are:
Name Age Weight(KG)
C Abhishek 35 80
D Vidit 36 70
E Anupam 34 73
나이가 33 이하인 모든 행을 삭제합니다.
먼저 나이가 33 이하인 모든 행의 인덱스를 찾은 다음drop()
메서드를 사용하여 행을 삭제합니다.
튜토리얼이 마음에 드시나요? DelftStack을 구독하세요 YouTube에서 저희가 더 많은 고품질 비디오 가이드를 제작할 수 있도록 지원해주세요. 구독하다
작가: Suraj Joshi
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn