Python でパーセンタイルを計算する
-
Python でパーセンタイルを計算する
scipy
パッケージを使用する -
Python で
NumPy
パッケージを用いてパーセンタイルを計算する -
Python で
math
パッケージを用いてパーセンタイルを計算する -
statistics
パッケージを用いた Python でパーセンタイルを計算する - NumPy の線形補間法を用いて Python でパーセンタイルを計算する
- NumPy の下部補間法を用いた Python でのパーセンタイルの計算
- NumPy の高次補間法を使って Python でパーセンタイルを計算する
- NumPy の中点補間メソッドを使って Python でパーセンタイルを計算する
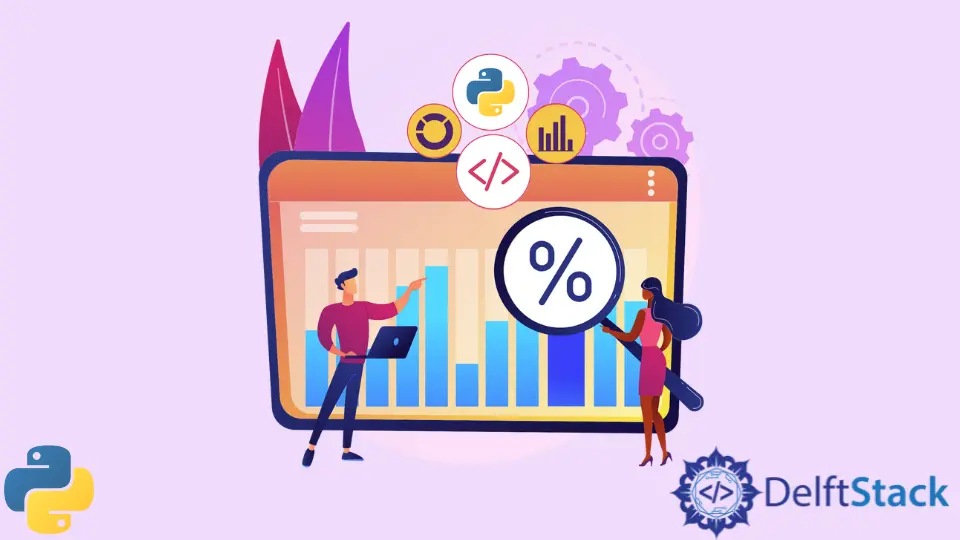
パーセンタイルは、特定の値を下回るスコアの割合を示しています。例えば、IQ が 120 の人はパーセンタイルが 91 で、彼の IQ が他の人の 91%よりも高いことを意味します。
この記事では、Python でパーセンタイルを計算する方法をいくつか紹介します。
Python でパーセンタイルを計算する scipy
パッケージを使用する
本パッケージは、入力系列のスコアを指定されたパーセンタイルで計算します。関数 scoreatpercentile()
の構文は以下の通りです。
scipy.stats.scoreatpercentile(
a, per, limit=(), interpolation_method="fraction", axis=None
)
関数 scoreatpercentile()
のパラメータ a
は 1 次元配列を表し、per
は 0 から 100 までの範囲のパーセンタイルを指定します。他の 2つのパラメータはオプションです。パーセンタイルを計算した数値を取得するには NumPy
ライブラリを使用します。
完全なサンプルコードを以下に示します。
from scipy import stats
import numpy as np
array = np.arange(100)
percentile = stats.scoreatpercentile(array, 50)
print("The percentile is:", percentile)
出力:
The percentile is: 49.5
Python で NumPy
パッケージを用いてパーセンタイルを計算する
本パッケージには、与えられた配列のパーセンタイルを計算する percentile()
関数があります。関数 percentile()
の構文は以下の通りです。
numpy.percentile(
a,
q,
axis=None,
out=None,
overwrite_input=False,
interpolation="linear",
keepdims=False,
)
パラメータ q
はパーセンタイルの計算数を表します。a
は配列を表し、他のパラメータは任意です。
完全なサンプルコードを以下に示します。
import numpy as np
arry = np.array([4, 6, 8, 10, 12])
percentile = np.percentile(arry, 50)
print("The percentile is:", percentile)
出力:
The percentile is: 8.0
Python で math
パッケージを用いてパーセンタイルを計算する
基本的な関数 ceil
を持つ math
パッケージは、異なるパーセンタイルを計算するのに用いることができます。
完全なサンプルコードを以下に示します。
import math
arry = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
def calculate_percentile(arry, percentile):
size = len(arry)
return sorted(arry)[int(math.ceil((size * percentile) / 100)) - 1]
percentile_25 = calculate_percentile(arry, 25)
percentile_50 = calculate_percentile(arry, 50)
percentile_75 = calculate_percentile(arry, 75)
print("The 25th percentile is:", percentile_25)
print("The 50th percentile is:", percentile_50)
print("The 75th percentile is:", percentile_75)
math.ceil(x)
は値を丸めて x
以上の最小の整数を返し、sorted
は配列をソートします。
出力:
The 25th percentile is: 3
The 50th percentile is: 5
The 75th percentile is: 8
statistics
パッケージを用いた Python でパーセンタイルを計算する
データを等確率に分解して n-1
の分布リストを返すには、statistics
パッケージの quantiles()
関数を用います。この関数の構文を以下に示します。
statistics.quantiles(data, *, n=4, method='exclusive')
完全なサンプルコードを以下に示します。
from statistics import quantiles
data = [1, 2, 3, 4, 5]
percentle = quantiles(data, n=4)
print("The Percentile is:", percentle)
出力:
The Percentile is: [1.5, 3.0, 4.5]
NumPy の線形補間法を用いて Python でパーセンタイルを計算する
補間モードを用いて異なるパーセンタイルを計算することができます。補間モードには linear
、lowower
、higher
、midpoint
、nearest
があります。これらの補間モードは、パーセンタイルが 2つのデータ点 i
と j
の間にある場合に使用されます。パーセンタイル値が i
の場合は低い補間モード、j
の場合は高い補間モード、i + (j - i) * fraction
は線形モードを表し、fraction
は i
と j
で囲まれたインデックスを示します。
線形補間モードの完全なサンプルコードを以下に示します。
import numpy as np
arry = np.array([1, 2, 3, 4, 5, 6, 7, 8, 9, 10])
print("percentiles using interpolation = ", "linear")
percentile_10 = np.percentile(arry, 10, interpolation="linear")
percentile_50 = np.percentile(arry, 50, interpolation="linear")
percentile_75 = np.percentile(arry, 75, interpolation="linear")
print(
"percentile_10 = ",
percentile_10,
", median = ",
percentile_50,
" and percentile_75 = ",
percentile_75,
)
関数 numpy.percentile()
を用いて、パラメータ interpolation
を追加しています。この補間では浮動小数点型の値を取得していることがわかります。
出力:
percentiles using interpolation = linear
percentile_10 = 1.9 , median = 5.5 and percentile_75 = 7.75
NumPy の下部補間法を用いた Python でのパーセンタイルの計算
下位補間モードの完全なサンプルコードを以下に示します。
import numpy as np
arry = np.array([1, 2, 3, 4, 5, 6, 7, 8, 9, 10])
print("percentiles using interpolation = ", "lower")
percentile_10 = np.percentile(arry, 10, interpolation="lower")
percentile_50 = np.percentile(arry, 50, interpolation="lower")
percentile_75 = np.percentile(arry, 75, interpolation="lower")
print(
"percentile_10 = ",
percentile_10,
", median = ",
percentile_50,
" and percentile_75 = ",
percentile_75,
)
出力:
percentiles using interpolation = lower
percentile_10 = 1 , median = 5 and percentile_75 = 7
最終的なパーセンタイルが最も低い値にルーデッドオフされていることがわかります。
NumPy の高次補間法を使って Python でパーセンタイルを計算する
このメソッドは、与えられた配列のパーセンタイルを、最も高い丸め込み値に与えます。
より高い補間モードの完全なサンプルコードを以下に示します。
import numpy as np
arry = np.array([1, 2, 3, 4, 5, 6, 7, 8, 9, 10])
print("percentiles using interpolation = ", "higher")
percentile_10 = np.percentile(arry, 10, interpolation="higher")
percentile_50 = np.percentile(arry, 50, interpolation="higher")
percentile_75 = np.percentile(arry, 75, interpolation="higher")
print(
"percentile_10 = ",
percentile_10,
", median = ",
percentile_50,
" and percentile_75 = ",
percentile_75,
)
出力:
percentiles using interpolation = higher
percentile_10 = 2 , median = 6 and percentile_75 = 8
NumPy の中点補間メソッドを使って Python でパーセンタイルを計算する
このメソッドは、パーセンタイル値の中点を与えます。
中点補間モードの完全なサンプルコードを以下に示します。
import numpy as np
arry = np.array([1, 2, 3, 4, 5, 6, 7, 8, 9, 10])
print("percentiles using interpolation = ", "midpoint")
percentile_10 = np.percentile(arry, 10, interpolation="midpoint")
percentile_50 = np.percentile(arry, 50, interpolation="midpoint")
percentile_75 = np.percentile(arry, 75, interpolation="midpoint")
print(
"percentile_10 = ",
percentile_10,
", median = ",
percentile_50,
" and percentile_75 = ",
percentile_75,
)
出力:
percentiles using interpolation = midpoint
percentile_10 = 1.5 , median = 5.5 and percentile_75 = 7.5
関連記事 - Python Math
- Python で階乗を計算する
- Python でモジュラ逆数を計算する
- Python で余弦の逆数を計算する
- Python での異なるデータセットへのポアソン分布のあてはめ
- Python での多項式回帰の実装
- Python で外れ値を検出して削除する