在 Python 中計算百分位數
-
在 Python 中使用
scipy
包計算百分位數 -
在 Python 中使用
NumPy
包計算百分位數 -
在 Python 中使用
math
包計算百分位數 -
在 Python 中使用
statistics
包計算百分位數 - 在 Python 中使用 NumPy 的線性插值方法計算百分位數
- 在 Python 中使用 NumPy 的下插值方法計算百分位數
- 在 Python 中使用 NumPy 的高階插值方法計算百分位數
- 在 Python 中使用 NumPy 的中點插值方法計算百分位數
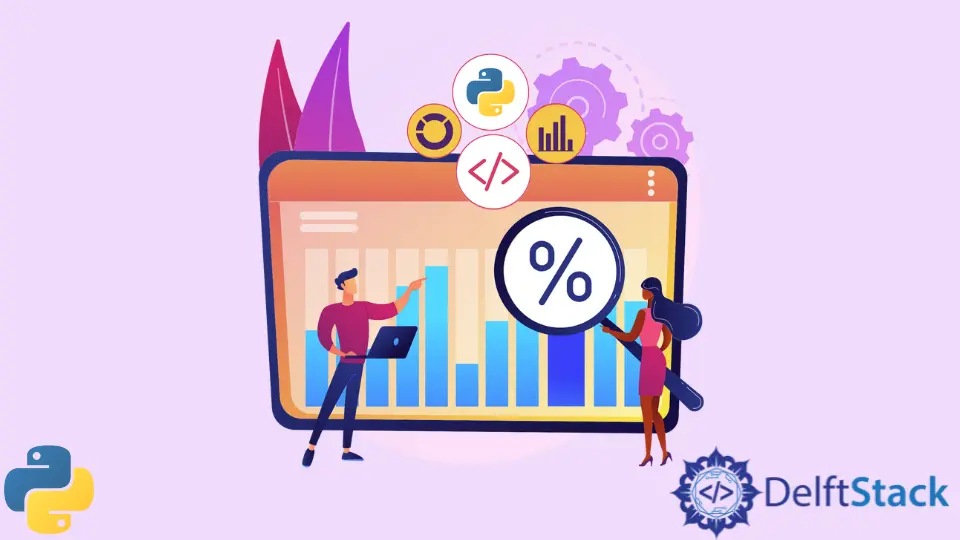
百分位數表示低於某一數值的分數的百分比。例如,一個人的智商為 120,就處於第 91 個百分位數,這意味著他的智商大於 91%的其他人。
本文將討論一些在 Python 中計算百分位數的方法。
在 Python 中使用 scipy
包計算百分位數
這個包將計算輸入序列在給定百分位的得分。scoreatpercentile()
函式的語法如下。
scipy.stats.scoreatpercentile(
a, per, limit=(), interpolation_method="fraction", axis=None
)
在 scoreatpercentile()
函式中,引數 a
代表一維陣列,per
指定 0 到 100 的百分位數。另外兩個引數是可選的。NumPy
庫用來獲取我們計算百分位數的數字。
完整的示例程式碼如下。
from scipy import stats
import numpy as np
array = np.arange(100)
percentile = stats.scoreatpercentile(array, 50)
print("The percentile is:", percentile)
輸出:
The percentile is: 49.5
在 Python 中使用 NumPy
包計算百分位數
這個包有一個 percentile()
函式,可以計算給定陣列的百分位數。percentile()
函式的語法如下。
numpy.percentile(
a,
q,
axis=None,
out=None,
overwrite_input=False,
interpolation="linear",
keepdims=False,
)
引數 q
代表百分位數的計算數。a
代表一個陣列,其他引數是可選的。
完整的示例程式碼如下。
import numpy as np
arry = np.array([4, 6, 8, 10, 12])
percentile = np.percentile(arry, 50)
print("The percentile is:", percentile)
輸出:
The percentile is: 8.0
在 Python 中使用 math
包計算百分位數
math
包及其基本函式-ceil
可用於計算不同的百分比。
完整的示例程式碼如下。
import math
arry = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
def calculate_percentile(arry, percentile):
size = len(arry)
return sorted(arry)[int(math.ceil((size * percentile) / 100)) - 1]
percentile_25 = calculate_percentile(arry, 25)
percentile_50 = calculate_percentile(arry, 50)
percentile_75 = calculate_percentile(arry, 75)
print("The 25th percentile is:", percentile_25)
print("The 50th percentile is:", percentile_50)
print("The 75th percentile is:", percentile_75)
math.ceil(x)
將數值四捨五入並返回大於或等於 x
的最小整數,而 sorted
函式則對陣列進行排序。
輸出:
The 25th percentile is: 3
The 50th percentile is: 5
The 75th percentile is: 8
在 Python 中使用 statistics
包計算百分位數
statistics
包中的 quantiles()
函式用於將資料分解為等概率,並返回 n-1
的分佈列表。該函式的語法如下。
statistics.quantiles(data, *, n=4, method='exclusive')
完整的示例程式碼如下。
from statistics import quantiles
data = [1, 2, 3, 4, 5]
percentle = quantiles(data, n=4)
print("The Percentile is:", percentle)
輸出:
The Percentile is: [1.5, 3.0, 4.5]
在 Python 中使用 NumPy 的線性插值方法計算百分位數
我們可以使用插值模式計算不同的百分比。插值模式有 linear
、lower
、higher
、midpoint
和 nearest
。當百分位數在兩個資料點 i
和 j
之間時,會使用這些插值。當百分位值為 i
時,為低位插值模式,j
代表高位插值模式,i + (j - i) * fraction
代表線性模式,其中 fraction
表示 i
和 j
包圍的索引。
下面給出了線性插值模式的完整示例程式碼。
import numpy as np
arry = np.array([1, 2, 3, 4, 5, 6, 7, 8, 9, 10])
print("percentiles using interpolation = ", "linear")
percentile_10 = np.percentile(arry, 10, interpolation="linear")
percentile_50 = np.percentile(arry, 50, interpolation="linear")
percentile_75 = np.percentile(arry, 75, interpolation="linear")
print(
"percentile_10 = ",
percentile_10,
", median = ",
percentile_50,
" and percentile_75 = ",
percentile_75,
)
我們使用 numpy.percentile()
函式,附加引數 interpolation
。你可以看到,我們得到的是這種插值的浮動值。
輸出:
percentiles using interpolation = linear
percentile_10 = 1.9 , median = 5.5 and percentile_75 = 7.75
在 Python 中使用 NumPy 的下插值方法計算百分位數
下面給出了較低插值模式的完整示例程式碼。
import numpy as np
arry = np.array([1, 2, 3, 4, 5, 6, 7, 8, 9, 10])
print("percentiles using interpolation = ", "lower")
percentile_10 = np.percentile(arry, 10, interpolation="lower")
percentile_50 = np.percentile(arry, 50, interpolation="lower")
percentile_75 = np.percentile(arry, 75, interpolation="lower")
print(
"percentile_10 = ",
percentile_10,
", median = ",
percentile_50,
" and percentile_75 = ",
percentile_75,
)
輸出:
percentiles using interpolation = lower
percentile_10 = 1 , median = 5 and percentile_75 = 7
你可以看到,最終的百分位數被粗略地調整到了最低值。
在 Python 中使用 NumPy 的高階插值方法計算百分位數
此方法將給出給定陣列的百分數,取最高舍入值。
下面給出了高插值模式的完整示例程式碼。
import numpy as np
arry = np.array([1, 2, 3, 4, 5, 6, 7, 8, 9, 10])
print("percentiles using interpolation = ", "higher")
percentile_10 = np.percentile(arry, 10, interpolation="higher")
percentile_50 = np.percentile(arry, 50, interpolation="higher")
percentile_75 = np.percentile(arry, 75, interpolation="higher")
print(
"percentile_10 = ",
percentile_10,
", median = ",
percentile_50,
" and percentile_75 = ",
percentile_75,
)
輸出:
percentiles using interpolation = higher
percentile_10 = 2 , median = 6 and percentile_75 = 8
在 Python 中使用 NumPy 的中點插值方法計算百分位數
此方法將給出百分位數的中點。
下面給出了中點插值模式的完整示例程式碼。
import numpy as np
arry = np.array([1, 2, 3, 4, 5, 6, 7, 8, 9, 10])
print("percentiles using interpolation = ", "midpoint")
percentile_10 = np.percentile(arry, 10, interpolation="midpoint")
percentile_50 = np.percentile(arry, 50, interpolation="midpoint")
percentile_75 = np.percentile(arry, 75, interpolation="midpoint")
print(
"percentile_10 = ",
percentile_10,
", median = ",
percentile_50,
" and percentile_75 = ",
percentile_75,
)
輸出:
percentiles using interpolation = midpoint
percentile_10 = 1.5 , median = 5.5 and percentile_75 = 7.5
相關文章 - Python Math
- 在 Python 中計算階乘
- 在 Python 中計算反餘弦
- 在 Python 中計算模乘逆
- 使用基本程式設計概念在 Python 中列印乘法表
- 用 Python 製作成績轉換器
- Python 中的級數求和