Python에서 백분위 수 계산
-
scipy
패키지를 사용하여 Python에서 백분위 수 계산 -
NumPy
패키지를 사용하여 Python에서 백분위 수 계산 -
math
패키지를 사용하여 Python에서 백분위 수 계산 -
statistics
패키지를 사용하여 Python에서 백분위 수 계산 - NumPy의 선형 보간 방법을 사용하여 Python에서 백분위 수 계산
- NumPy의 하부 보간법을 이용한 Python의 백분위수 계산
- NumPy의 더 높은 보간 방법을 사용하여 Python에서 백분위 수 계산
- NumPy의 중간 점 보간 방법을 사용하여 Python에서 백분위 수 계산
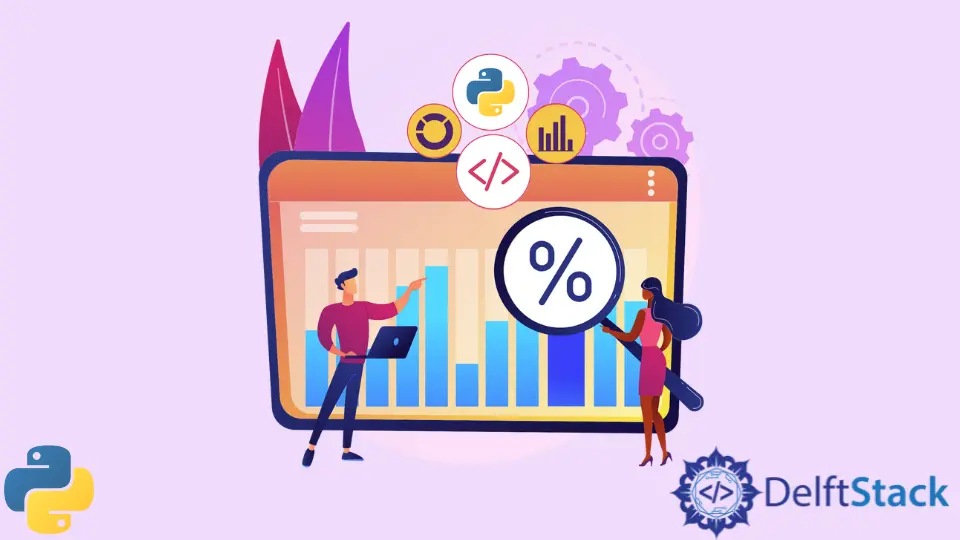
백분위 수는 특정 값 아래로 떨어지는 점수의 백분율을 나타냅니다. 예를 들어, IQ가 120 인 개인은 91 번째 백분위 수에 있으며 이는 그의 IQ가 다른 사람들의 91 %보다 크다는 것을 의미합니다.
이 기사에서는 Python에서 백분위 수를 계산하는 몇 가지 방법에 대해 설명합니다.
scipy
패키지를 사용하여 Python에서 백분위 수 계산
이 패키지는 주어진 백분위 수에서 입력 시리즈의 점수를 계산합니다. scoreatpercentile()
함수의 구문은 다음과 같습니다.
scipy.stats.scoreatpercentile(
a, per, limit=(), interpolation_method="fraction", axis=None
)
scoreatpercentile()
함수에서a
매개 변수는 1D 배열을 나타내고per
는 0에서 100까지의 백분위 수를 지정합니다. 다른 두 매개 변수는 선택 사항입니다. NumPy
라이브러리는 백분위 수를 계산 한 숫자를 가져 오는 데 사용됩니다.
전체 예제 코드는 다음과 같습니다.
from scipy import stats
import numpy as np
array = np.arange(100)
percentile = stats.scoreatpercentile(array, 50)
print("The percentile is:", percentile)
출력:
The percentile is: 49.5
NumPy
패키지를 사용하여 Python에서 백분위 수 계산
이 패키지에는 주어진 배열의 백분위 수를 계산하는percentile()
함수가 있습니다. percentile()
함수의 구문은 다음과 같습니다.
numpy.percentile(
a,
q,
axis=None,
out=None,
overwrite_input=False,
interpolation="linear",
keepdims=False,
)
매개 변수q
는 백분위 수 계산 수를 나타냅니다. a
는 배열을 나타내며 다른 매개 변수는 선택 사항입니다.
전체 예제 코드는 다음과 같습니다.
import numpy as np
arry = np.array([4, 6, 8, 10, 12])
percentile = np.percentile(arry, 50)
print("The percentile is:", percentile)
출력:
The percentile is: 8.0
math
패키지를 사용하여 Python에서 백분위 수 계산
기본 기능인ceil
이있는math
패키지를 사용하여 다른 백분위 수를 계산할 수 있습니다.
전체 예제 코드는 다음과 같습니다.
import math
arry = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
def calculate_percentile(arry, percentile):
size = len(arry)
return sorted(arry)[int(math.ceil((size * percentile) / 100)) - 1]
percentile_25 = calculate_percentile(arry, 25)
percentile_50 = calculate_percentile(arry, 50)
percentile_75 = calculate_percentile(arry, 75)
print("The 25th percentile is:", percentile_25)
print("The 50th percentile is:", percentile_50)
print("The 75th percentile is:", percentile_75)
math.ceil(x)
는 값을 반올림하고x
보다 크거나 같은 가장 작은 정수를 반환하는 반면sorted
함수는 배열을 정렬합니다.
출력:
The 25th percentile is: 3
The 50th percentile is: 5
The 75th percentile is: 8
statistics
패키지를 사용하여 Python에서 백분위 수 계산
statistics
패키지의quantiles()
함수는 데이터를 동일한 확률로 나누고n-1
의 배포 목록을 반환하는 데 사용됩니다. 이 함수의 구문은 다음과 같습니다.
statistics.quantiles(data, *, n=4, method='exclusive')
전체 예제 코드는 다음과 같습니다.
from statistics import quantiles
data = [1, 2, 3, 4, 5]
percentle = quantiles(data, n=4)
print("The Percentile is:", percentle)
출력:
The Percentile is: [1.5, 3.0, 4.5]
NumPy의 선형 보간 방법을 사용하여 Python에서 백분위 수 계산
보간 모드를 사용하여 다른 백분위 수를 계산할 수 있습니다. 보간 모드는linear
, lower
, higher
, midpoint
및nearest
입니다. 이러한 보간은 백분위 수가 두 데이터 포인트i
및j
사이에있을 때 사용됩니다. 백분위 수 값이i
이면 하위 보간 모드,j
는 상위 보간 모드,i + (j-i) * fraction
은fraction
이 i
및j
로 둘러싸인 인덱스를 나타내는 선형 모드를 나타냅니다.
선형 보간 모드에 대한 전체 예제 코드는 다음과 같습니다.
import numpy as np
arry = np.array([1, 2, 3, 4, 5, 6, 7, 8, 9, 10])
print("percentiles using interpolation = ", "linear")
percentile_10 = np.percentile(arry, 10, interpolation="linear")
percentile_50 = np.percentile(arry, 50, interpolation="linear")
percentile_75 = np.percentile(arry, 75, interpolation="linear")
print(
"percentile_10 = ",
percentile_10,
", median = ",
percentile_50,
" and percentile_75 = ",
percentile_75,
)
추가 매개 변수interpolation
과 함께numpy.percentile()
함수를 사용합니다. 이 보간을 위해 float 값을 얻는 것을 볼 수 있습니다.
출력:
percentiles using interpolation = linear
percentile_10 = 1.9 , median = 5.5 and percentile_75 = 7.75
NumPy의 하부 보간법을 이용한 Python의 백분위수 계산
하위 보간 모드에 대한 전체 예제 코드는 다음과 같습니다.
import numpy as np
arry = np.array([1, 2, 3, 4, 5, 6, 7, 8, 9, 10])
print("percentiles using interpolation = ", "lower")
percentile_10 = np.percentile(arry, 10, interpolation="lower")
percentile_50 = np.percentile(arry, 50, interpolation="lower")
percentile_75 = np.percentile(arry, 75, interpolation="lower")
print(
"percentile_10 = ",
percentile_10,
", median = ",
percentile_50,
" and percentile_75 = ",
percentile_75,
)
출력:
percentiles using interpolation = lower
percentile_10 = 1 , median = 5 and percentile_75 = 7
최종 백분위 수가 가장 낮은 값으로 라우팅되는 것을 볼 수 있습니다.
NumPy의 더 높은 보간 방법을 사용하여 Python에서 백분위 수 계산
이 방법은 주어진 배열의 백분위 수를 가장 높은 반올림 값으로 제공합니다.
더 높은 보간 모드에 대한 전체 예제 코드는 다음과 같습니다.
import numpy as np
arry = np.array([1, 2, 3, 4, 5, 6, 7, 8, 9, 10])
print("percentiles using interpolation = ", "higher")
percentile_10 = np.percentile(arry, 10, interpolation="higher")
percentile_50 = np.percentile(arry, 50, interpolation="higher")
percentile_75 = np.percentile(arry, 75, interpolation="higher")
print(
"percentile_10 = ",
percentile_10,
", median = ",
percentile_50,
" and percentile_75 = ",
percentile_75,
)
출력:
percentiles using interpolation = higher
percentile_10 = 2 , median = 6 and percentile_75 = 8
NumPy의 중간 점 보간 방법을 사용하여 Python에서 백분위 수 계산
이 방법은 백분위 수 값의 중간 점을 제공합니다.
중간 점 보간 모드에 대한 전체 예제 코드는 다음과 같습니다.
import numpy as np
arry = np.array([1, 2, 3, 4, 5, 6, 7, 8, 9, 10])
print("percentiles using interpolation = ", "midpoint")
percentile_10 = np.percentile(arry, 10, interpolation="midpoint")
percentile_50 = np.percentile(arry, 50, interpolation="midpoint")
percentile_75 = np.percentile(arry, 75, interpolation="midpoint")
print(
"percentile_10 = ",
percentile_10,
", median = ",
percentile_50,
" and percentile_75 = ",
percentile_75,
)
출력:
percentiles using interpolation = midpoint
percentile_10 = 1.5 , median = 5.5 and percentile_75 = 7.5
관련 문장 - Python Math
- Python에서 팩토리얼 계산
- Python에서 Modular Multiplicative Inverse 계산하기
- Python에서 코사인의 역수 계산
- Python에서 다항식 회귀 구현
- Python에서 무한 값 정의
- Python에서 이상값 감지 및 제거