JavaFX の ChoiceBox から選択したアイテムを取得する
Sheeraz Gul
2023年10月12日
Java
Java JavaFX
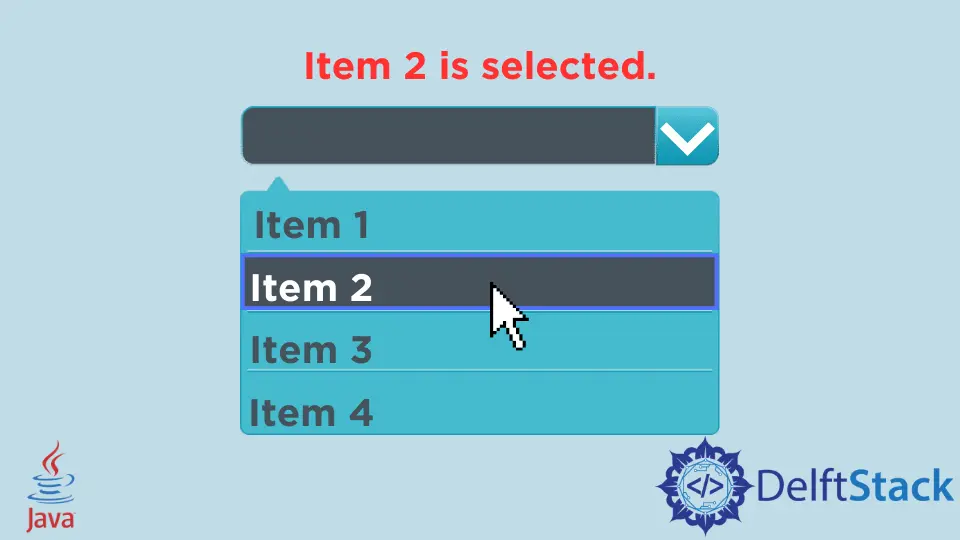
ChoiceBox
は JavaFX
ライブラリの一部であり、そこから選択した選択肢を取得できます。このチュートリアルでは、JavaFX の ChoiceBox
から選択したアイテムを取得する方法を示します。
JavaFX の ChoiceBox
から選択したアイテムを取得する
ChoiceBox
には、ユーザーが現在選択されているアイテムとなる選択肢を選択できるアイテムのセットが含まれています。選択ボックスのデフォルトの選択肢は null
エントリです。
ChoiceBox
を操作するには、次のメソッドを使用します。
方法 | 説明 |
---|---|
hide() |
このメソッドは、選択肢のリストを閉じます。 |
setItems(ObservableList value) |
これにより、プロパティアイテムの値が設定されます。 |
setValue(T value) |
これにより、プロパティ値の値が設定されます。 |
getItems() |
これにより、プロパティアイテムの値が取得されます。 |
getValue() |
これにより、プロパティ値の値が取得されます。 |
show() |
これにより、選択肢のリストが開きます。 |
次の方法を使用して、選択ボックスから選択したアイテムを取得します。
ChoiceBox.getSelectionModel().selectedIndexProperty()
JavaFX を使用して、ChoiceBox
から選択したアイテムを取得しましょう。
package delftstack;
import javafx.application.Application;
import javafx.beans.value.*;
import javafx.collections.*;
import javafx.event.ActionEvent;
import javafx.event.EventHandler;
import javafx.scene.Scene;
import javafx.scene.control.*;
import javafx.scene.layout.*;
import javafx.stage.Stage;
public class Choice_Box extends Application {
public void start(Stage Choice_Box_Stage) {
// title for the stage
Choice_Box_Stage.setTitle("ChoiceBox");
// button to show
Button Show_Button = new Button("Show Choice");
// tile pane
TilePane Title_Pane = new TilePane();
// labels
Label Label1 = new Label("This is a choice box, Please select your choice");
Label Label2 = new Label("No Choice selected");
// Choices array
String Choice_Array[] = {
"Delftstack 1", "Delftstack 2", "Delftstack 3", "Delftstack 4", "Delftstack 5"};
// choiceBox
ChoiceBox DemoChoiceBox = new ChoiceBox(FXCollections.observableArrayList(Choice_Array));
// adding a listener
DemoChoiceBox.getSelectionModel().selectedIndexProperty().addListener(
new ChangeListener<Number>() {
// if items of the list are changed
public void changed(ObservableValue ov, Number value, Number new_value) {
// text for the label to the selected item
Label2.setText(Choice_Array[new_value.intValue()] + " is Selected");
}
});
// ChoiceBox
Title_Pane.getChildren().add(Label1);
Title_Pane.getChildren().add(DemoChoiceBox);
Title_Pane.getChildren().add(Label2);
Scene sc = new Scene(Title_Pane, 400, 200);
// Setting the scene
Choice_Box_Stage.setScene(sc);
Choice_Box_Stage.show();
}
public static void main(String args[]) {
// launching the application
launch(args);
}
}
上記のコードは、JavaFX を使用して ChoiceBox
から選択アイテムを取得する方法を示しています。出力を参照してください:
チュートリアルを楽しんでいますか? <a href="https://www.youtube.com/@delftstack/?sub_confirmation=1" style="color: #a94442; font-weight: bold; text-decoration: underline;">DelftStackをチャンネル登録</a> して、高品質な動画ガイドをさらに制作するためのサポートをお願いします。 Subscribe
著者: Sheeraz Gul
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook