從 JavaFX 中的選擇框中獲取所選專案
Sheeraz Gul
2023年10月12日
Java
Java JavaFX
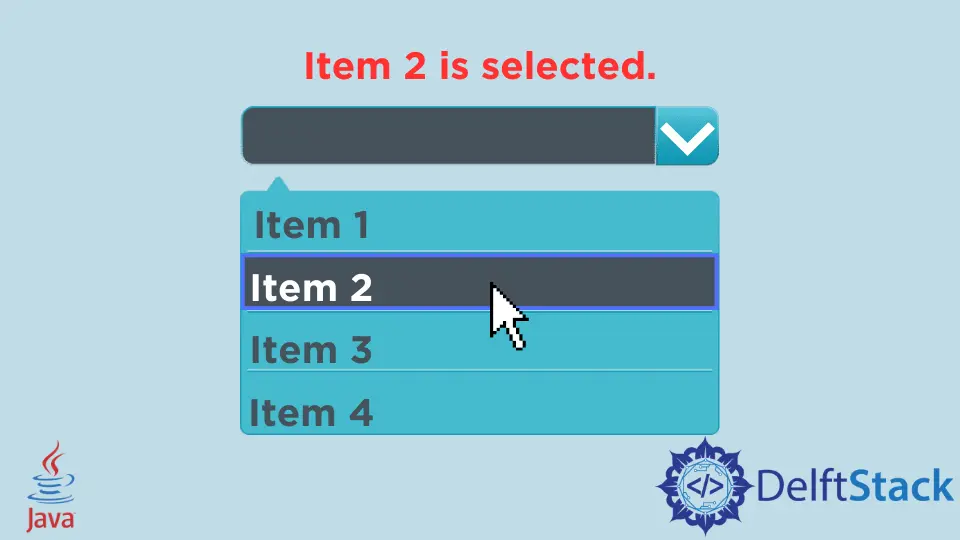
ChoiceBox
是 JavaFX
庫的一部分,我們可以從中獲得選定的選項。本教程演示瞭如何從 JavaFX 中的 ChoiceBox
中獲取選定專案。
從 JavaFX 中的 ChoiceBox
中獲取所選專案
ChoiceBox
包含一組專案,使用者可以從中選擇將成為當前選定專案的選項。選擇框中的預設選擇是空
條目。
以下方法用於使用 ChoiceBox
。
方法 | 說明 |
---|---|
hide() |
此方法將關閉選項列表。 |
setItems(ObservableList value) |
這將設定屬性項的值。 |
setValue(T value) |
這將設定屬性值的值。 |
getItems() |
這將獲得屬性項的值。 |
getValue() |
這將獲取屬性值的值。 |
show() |
這將開啟選項列表。 |
我們使用以下方法從選擇框中獲取選定的專案。
ChoiceBox.getSelectionModel().selectedIndexProperty()
讓我們使用 JavaFX 從 ChoiceBox
中獲取選定專案。
package delftstack;
import javafx.application.Application;
import javafx.beans.value.*;
import javafx.collections.*;
import javafx.event.ActionEvent;
import javafx.event.EventHandler;
import javafx.scene.Scene;
import javafx.scene.control.*;
import javafx.scene.layout.*;
import javafx.stage.Stage;
public class Choice_Box extends Application {
public void start(Stage Choice_Box_Stage) {
// title for the stage
Choice_Box_Stage.setTitle("ChoiceBox");
// button to show
Button Show_Button = new Button("Show Choice");
// tile pane
TilePane Title_Pane = new TilePane();
// labels
Label Label1 = new Label("This is a choice box, Please select your choice");
Label Label2 = new Label("No Choice selected");
// Choices array
String Choice_Array[] = {
"Delftstack 1", "Delftstack 2", "Delftstack 3", "Delftstack 4", "Delftstack 5"};
// choiceBox
ChoiceBox DemoChoiceBox = new ChoiceBox(FXCollections.observableArrayList(Choice_Array));
// adding a listener
DemoChoiceBox.getSelectionModel().selectedIndexProperty().addListener(
new ChangeListener<Number>() {
// if items of the list are changed
public void changed(ObservableValue ov, Number value, Number new_value) {
// text for the label to the selected item
Label2.setText(Choice_Array[new_value.intValue()] + " is Selected");
}
});
// ChoiceBox
Title_Pane.getChildren().add(Label1);
Title_Pane.getChildren().add(DemoChoiceBox);
Title_Pane.getChildren().add(Label2);
Scene sc = new Scene(Title_Pane, 400, 200);
// Setting the scene
Choice_Box_Stage.setScene(sc);
Choice_Box_Stage.show();
}
public static void main(String args[]) {
// launching the application
launch(args);
}
}
上面的程式碼演示了使用 JavaFX 從 ChoiceBox
中獲取選擇項。見輸出:
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Sheeraz Gul
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook