Obtenga el elemento seleccionado de un ChoiceBox en JavaFX
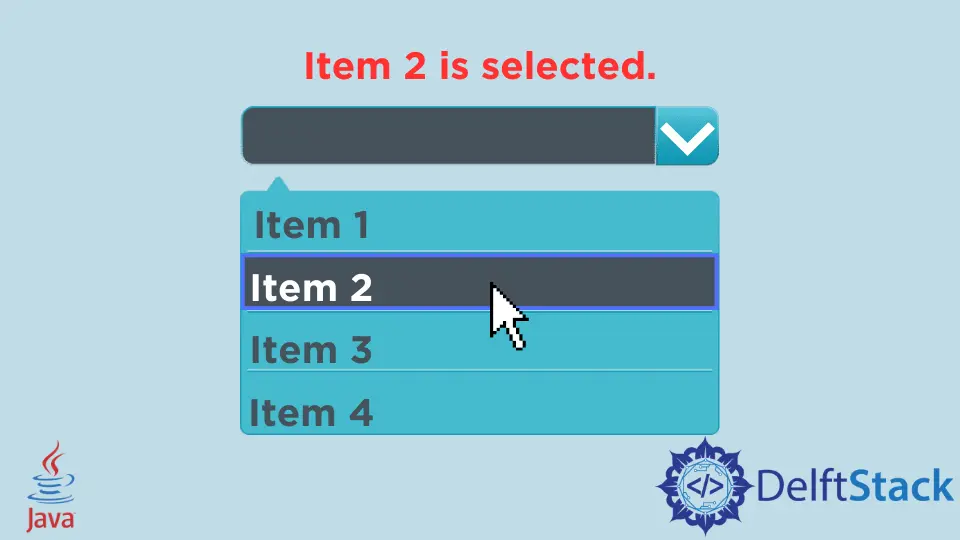
ChoiceBox
es una parte de la biblioteca JavaFX
de la que podemos obtener una opción seleccionada. Este tutorial demuestra cómo obtener un elemento seleccionado de un ChoiceBox
en JavaFX.
Obtenga el elemento seleccionado de un ChoiceBox
en JavaFX
ChoiceBox
contiene un conjunto de elementos de los que un usuario puede seleccionar una opción que será el elemento seleccionado actualmente. La opción predeterminada en un cuadro de opciones es una entrada nula
.
Los siguientes métodos se utilizan para trabajar con el ChoiceBox
.
Método | Descripción |
---|---|
hide() |
Este método cerrará la lista de opciones. |
setItems(ObservableList value) |
Esto establece el valor de los elementos de propiedad. |
setValue(T value) |
Esto establecerá el valor del valor de la propiedad. |
getItems() |
Esto obtendrá el valor de los elementos de propiedad. |
getValue() |
Esto obtiene el valor del valor de la propiedad. |
show() |
Esto abrirá la lista de opciones. |
Usamos el siguiente método para obtener el elemento seleccionado de un cuadro de elección.
ChoiceBox.getSelectionModel().selectedIndexProperty()
Obtengamos un elemento seleccionado de un ChoiceBox
usando JavaFX.
package delftstack;
import javafx.application.Application;
import javafx.beans.value.*;
import javafx.collections.*;
import javafx.event.ActionEvent;
import javafx.event.EventHandler;
import javafx.scene.Scene;
import javafx.scene.control.*;
import javafx.scene.layout.*;
import javafx.stage.Stage;
public class Choice_Box extends Application {
public void start(Stage Choice_Box_Stage) {
// title for the stage
Choice_Box_Stage.setTitle("ChoiceBox");
// button to show
Button Show_Button = new Button("Show Choice");
// tile pane
TilePane Title_Pane = new TilePane();
// labels
Label Label1 = new Label("This is a choice box, Please select your choice");
Label Label2 = new Label("No Choice selected");
// Choices array
String Choice_Array[] = {
"Delftstack 1", "Delftstack 2", "Delftstack 3", "Delftstack 4", "Delftstack 5"};
// choiceBox
ChoiceBox DemoChoiceBox = new ChoiceBox(FXCollections.observableArrayList(Choice_Array));
// adding a listener
DemoChoiceBox.getSelectionModel().selectedIndexProperty().addListener(
new ChangeListener<Number>() {
// if items of the list are changed
public void changed(ObservableValue ov, Number value, Number new_value) {
// text for the label to the selected item
Label2.setText(Choice_Array[new_value.intValue()] + " is Selected");
}
});
// ChoiceBox
Title_Pane.getChildren().add(Label1);
Title_Pane.getChildren().add(DemoChoiceBox);
Title_Pane.getChildren().add(Label2);
Scene sc = new Scene(Title_Pane, 400, 200);
// Setting the scene
Choice_Box_Stage.setScene(sc);
Choice_Box_Stage.show();
}
public static void main(String args[]) {
// launching the application
launch(args);
}
}
El código anterior muestra cómo obtener un elemento seleccionado de un ChoiceBox
con JavaFX. Ver salida:
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook