How to Define Type for Function Callbacks in TypeScript
- What are Callback Functions in TypeScript
- Use of Interface for Callback Type in TypeScript
- Use of Generic for Callback Type in TypeScript
-
Use
Type
Keyword to Declare Callback Type in TypeScript
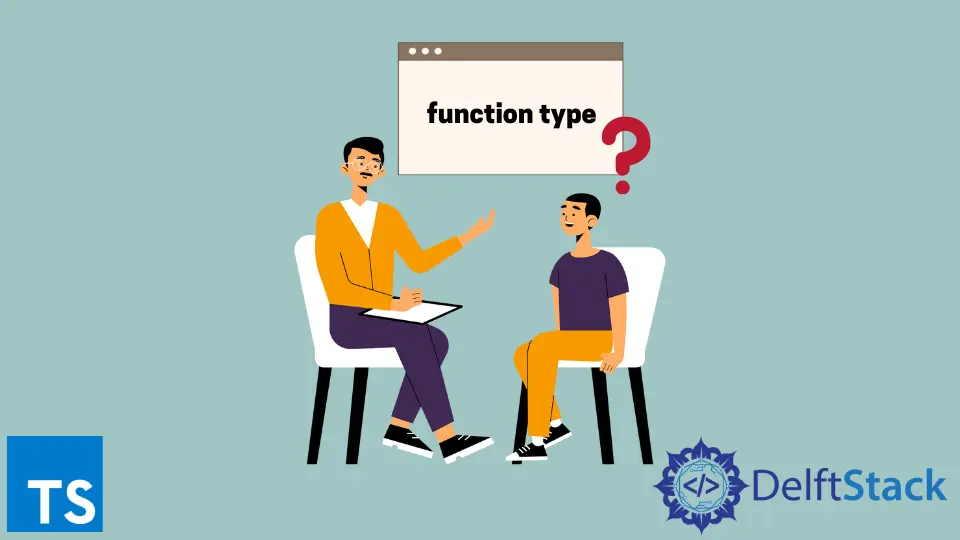
This tutorial demonstrates defining a type for a function callback in a TypeScript. Different solution and coding exercises representing Function Callbacks in TypeScript are illustrated.
Before exploring the solutions and how they can be done, let us look into callback functions.
What are Callback Functions in TypeScript
A callback
function is defined as a function passed into another function as an argument, which is then invoked inside the outer function to complete the desirable routine or action.
function outerFunction(callback: () => void) {
callback();
}
The following are popular approaches to define the type of function callback in TypeScript in the developer community.
Use of Interface for Callback Type in TypeScript
TypeScript allows programmers to define a general definition for a variable or a function by declaring an interface
, which helps define a function’s call signature
type.
Let’s consider the following code.
const greeting = (message:string) => console.log(`Hello ${message}`);
interface definitionInterface{
(message:string):void;
}
function sayHello(callback: definitionInterface) {
callback('World!')
}
sayHello(greeting);
The interface
has a call signature of definitionInterface
sets the callback
parameter type to string and return type of void, which means the value of callback will be ignored.
Let us begin to see callback detail.
Changing the function signature, i.e., greeting to a number instead of a string, will show an error that the type is string is not assignable to the type number.
It creates a strong callback instead of just passing around functions like we normally do in JavaScript.
Use of Generic for Callback Type in TypeScript
Generic
in TypeScript allows us to generalize callback with a parameter as below. It gives you the advantage of not defining, again and again, a new interface with a new name each time you need a callback with a parameter.
The callback will not return any value most of the time, so the typeTwo
type is default set to void. But in case you want to return a value from a callback function, you could specify the typeTwo
type.
interface callackOneParameter<typeTwo, typeTwo = void> {
(param1: typeTwo): typeTwo;
}
Let us see the following two example codes which use the Generic
interface.
Void:
interface callBackOneParameter<typeOne, typeTwo =void> {
(param1: typeOne): typeTwo;
}
function greet(message: string) {
console.log(`${message}, how are you doing?`)
}
function sayHello(callback: callBackOneParameter<string>) {
callback('Hello')
}
sayHello(greet)
This code generates the following outcome.
With Type:
interface callBackOneParameter<typeOne, typeTwo =void> {
(param1: typeOne): typeTwo;
}
function greet(message: string) {
return `${message}, how are you doing?`
}
function sayHello(greet: callBackOneParameter<string, string>) {
let message = greet('Hi Ibrahim!')
console.log(message)
}
sayHello(greet)
The above code generates the following outcome.
Use Type
Keyword to Declare Callback Type in TypeScript
In TypeScript, the type
keyword declares a type
alias. So with the help of the type
keyword, you could declare your callback type as shown below.
type callBackFunction = () => void;
This declares a function that does not take any arguments and returns nothing. Then a function that can take zero to more arguments of type any and returns nothing will be like below.
//Then you can utilize it as
let callback: CallbackFunctionVariadic = function(...args: any[]) {
// do some stuff
};
A function could take an arbitrary number of arguments and return a void value.
type CallbackFunctionVariadicAnyReturn = (...args: any[]) => any;
Ibrahim is a Full Stack developer working as a Software Engineer in a reputable international organization. He has work experience in technologies stack like MERN and Spring Boot. He is an enthusiastic JavaScript lover who loves to provide and share research-based solutions to problems. He loves problem-solving and loves to write solutions of those problems with implemented solutions.
LinkedIn