在 TypeScript 中定义函数回调的类型
- 什么是 TypeScript 中的回调函数
- TypeScript 中回调类型接口的使用
- 在 TypeScript 中使用泛型作为回调类型
-
在 TypeScript 中使用
Type
关键字声明回调类型
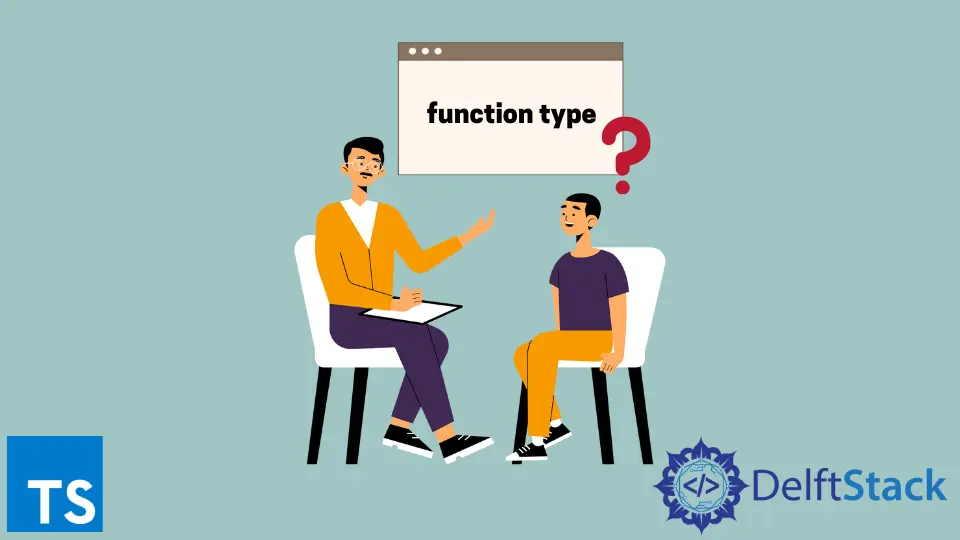
本教程演示了在 TypeScript 中为函数回调定义类型。说明了在 TypeScript 中表示函数回调的不同解决方案和编码练习。
在探索解决方案以及如何完成之前,让我们看看回调函数。
什么是 TypeScript 中的回调函数
callback
函数定义为作为参数传递给另一个函数的函数,然后在外部函数内部调用该函数以完成所需的例程或操作。
function outerFunction(callback: () => void) {
callback();
}
以下是开发者社区中在 TypeScript 中定义函数回调类型的流行方法。
TypeScript 中回调类型接口的使用
TypeScript 允许程序员通过声明接口
来定义变量或函数的一般定义,这有助于定义函数的调用签名
类型。
让我们考虑以下代码。
const greeting = (message:string) => console.log(`Hello ${message}`);
interface definitionInterface{
(message:string):void;
}
function sayHello(callback: definitionInterface) {
callback('World!')
}
sayHello(greeting);
interface
具有 definitionInterface
的调用签名,将 callback
参数类型设置为字符串,返回类型为 void,这意味着回调的值将被忽略。
让我们开始看看回调细节。
更改函数签名,即用数字而不是字符串打招呼,将显示类型为字符串不可分配给类型编号的错误。
它创建了一个强大的回调,而不是像我们通常在 JavaScript 中那样传递函数。
在 TypeScript 中使用泛型作为回调类型
TypeScript 中的 Generic
允许我们使用如下参数泛化回调。它给你的好处是,每次你需要一个带参数的回调时,一次又一次地用新名称定义一个新接口。
回调在大多数情况下不会返回任何值,因此 typeTwo
类型默认设置为 void。但是如果你想从回调函数返回一个值,你可以指定 typeTwo
类型。
interface callackOneParameter<typeTwo, typeTwo = void> {
(param1: typeTwo): typeTwo;
}
让我们看看下面两个使用 Generic
接口的示例代码。
空白:
interface callBackOneParameter<typeOne, typeTwo =void> {
(param1: typeOne): typeTwo;
}
function greet(message: string) {
console.log(`${message}, how are you doing?`)
}
function sayHello(callback: callBackOneParameter<string>) {
callback('Hello')
}
sayHello(greet)
此代码生成以下结果。
带类型:
interface callBackOneParameter<typeOne, typeTwo =void> {
(param1: typeOne): typeTwo;
}
function greet(message: string) {
return `${message}, how are you doing?`
}
function sayHello(greet: callBackOneParameter<string, string>) {
let message = greet('Hi Ibrahim!')
console.log(message)
}
sayHello(greet)
上面的代码生成以下结果。
在 TypeScript 中使用 Type
关键字声明回调类型
在 TypeScript 中,type
关键字声明了一个 type
别名。因此,在 type
关键字的帮助下,你可以声明你的回调类型,如下所示。
type callBackFunction = () => void;
这声明了一个不接受任何参数并且不返回任何内容的函数。然后,一个可以接受零到更多任何类型的参数并且不返回任何内容的函数将如下所示。
//Then you can utilize it as
let callback: CallbackFunctionVariadic = function(...args: any[]) {
// do some stuff
};
一个函数可以接受任意数量的参数并返回一个 void 值。
type CallbackFunctionVariadicAnyReturn = (...args: any[]) => any;
Ibrahim is a Full Stack developer working as a Software Engineer in a reputable international organization. He has work experience in technologies stack like MERN and Spring Boot. He is an enthusiastic JavaScript lover who loves to provide and share research-based solutions to problems. He loves problem-solving and loves to write solutions of those problems with implemented solutions.
LinkedIn