在 TypeScript 中定義函式回撥的型別
- 什麼是 TypeScript 中的回撥函式
- TypeScript 中回撥型別介面的使用
- 在 TypeScript 中使用泛型作為回撥型別
-
在 TypeScript 中使用
Type
關鍵字宣告回撥型別
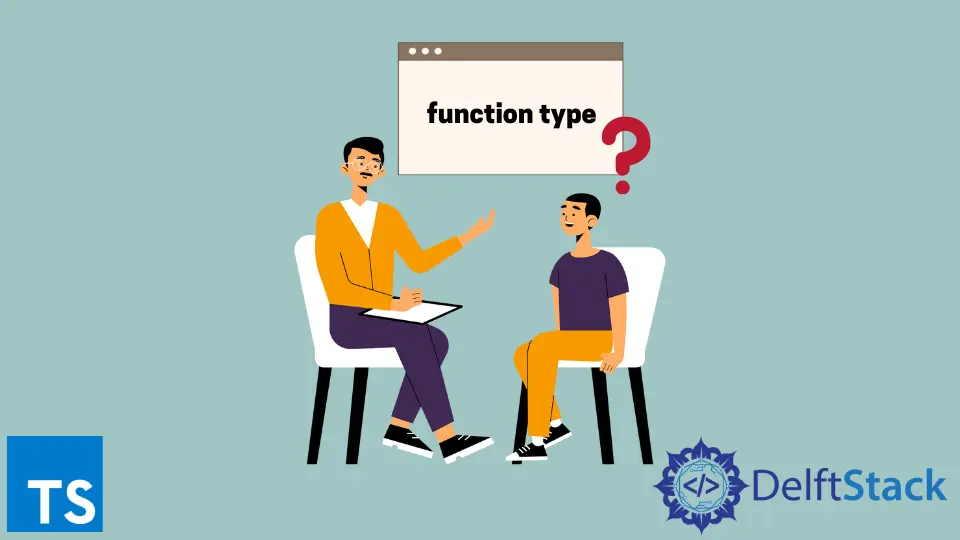
本教程演示了在 TypeScript 中為函式回撥定義型別。說明了在 TypeScript 中表示函式回撥的不同解決方案和編碼練習。
在探索解決方案以及如何完成之前,讓我們看看回撥函式。
什麼是 TypeScript 中的回撥函式
callback
函式定義為作為引數傳遞給另一個函式的函式,然後在外部函式內部呼叫該函式以完成所需的例程或操作。
function outerFunction(callback: () => void) {
callback();
}
以下是開發者社群中在 TypeScript 中定義函式回撥型別的流行方法。
TypeScript 中回撥型別介面的使用
TypeScript 允許程式設計師通過宣告介面
來定義變數或函式的一般定義,這有助於定義函式的呼叫簽名
型別。
讓我們考慮以下程式碼。
const greeting = (message:string) => console.log(`Hello ${message}`);
interface definitionInterface{
(message:string):void;
}
function sayHello(callback: definitionInterface) {
callback('World!')
}
sayHello(greeting);
interface
具有 definitionInterface
的呼叫簽名,將 callback
引數型別設定為字串,返回型別為 void,這意味著回撥的值將被忽略。
讓我們開始看看回撥細節。
更改函式簽名,即用數字而不是字串打招呼,將顯示型別為字串不可分配給型別編號的錯誤。
它建立了一個強大的回撥,而不是像我們通常在 JavaScript 中那樣傳遞函式。
在 TypeScript 中使用泛型作為回撥型別
TypeScript 中的 Generic
允許我們使用如下引數泛化回撥。它給你的好處是,每次你需要一個帶引數的回撥時,一次又一次地用新名稱定義一個新介面。
回撥在大多數情況下不會返回任何值,因此 typeTwo
型別預設設定為 void。但是如果你想從回撥函式返回一個值,你可以指定 typeTwo
型別。
interface callackOneParameter<typeTwo, typeTwo = void> {
(param1: typeTwo): typeTwo;
}
讓我們看看下面兩個使用 Generic
介面的示例程式碼。
空白:
interface callBackOneParameter<typeOne, typeTwo =void> {
(param1: typeOne): typeTwo;
}
function greet(message: string) {
console.log(`${message}, how are you doing?`)
}
function sayHello(callback: callBackOneParameter<string>) {
callback('Hello')
}
sayHello(greet)
此程式碼生成以下結果。
帶型別:
interface callBackOneParameter<typeOne, typeTwo =void> {
(param1: typeOne): typeTwo;
}
function greet(message: string) {
return `${message}, how are you doing?`
}
function sayHello(greet: callBackOneParameter<string, string>) {
let message = greet('Hi Ibrahim!')
console.log(message)
}
sayHello(greet)
上面的程式碼生成以下結果。
在 TypeScript 中使用 Type
關鍵字宣告回撥型別
在 TypeScript 中,type
關鍵字宣告瞭一個 type
別名。因此,在 type
關鍵字的幫助下,你可以宣告你的回撥型別,如下所示。
type callBackFunction = () => void;
這宣告瞭一個不接受任何引數並且不返回任何內容的函式。然後,一個可以接受零到更多任何型別的引數並且不返回任何內容的函式將如下所示。
//Then you can utilize it as
let callback: CallbackFunctionVariadic = function(...args: any[]) {
// do some stuff
};
一個函式可以接受任意數量的引數並返回一個 void 值。
type CallbackFunctionVariadicAnyReturn = (...args: any[]) => any;
Ibrahim is a Full Stack developer working as a Software Engineer in a reputable international organization. He has work experience in technologies stack like MERN and Spring Boot. He is an enthusiastic JavaScript lover who loves to provide and share research-based solutions to problems. He loves problem-solving and loves to write solutions of those problems with implemented solutions.
LinkedIn