How to Return Type of a Function in TypeScript
-
Use Types for
return
Statements in Functions in TypeScript -
Use the
ReturnType
Type to Get the Return Type of a Function in TypeScript
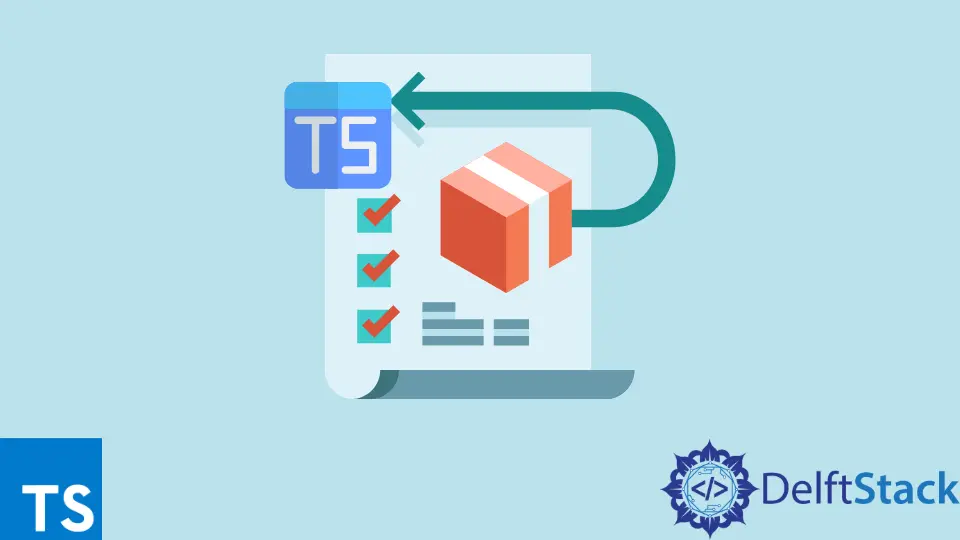
TypeScript is a strongly typed language, and it is always encouraged to have properly defined types for all the variables used in TypeScript. These types can later help in development, particularly during debugging.
Apart from variables, functions can also have types for the return types. Usually, when a type is not defined, the compiler automatically infers the types based on the type of the variables returned.
However, this is inconvenient and can cause less strict types like any
or even undefined
as the return type, so it is always better to return type to TypeScript functions.
Use Types for return
Statements in Functions in TypeScript
The TypeScript compiler automatically infers the return type if any type is not mentioned. It is always better to add types to functions to ensure proper types are passed on through the application code.
The following shows how types can be added to TypeScript functions.
function addTwoNumbers( a : number, b : number) : number {
return a + b;
}
console.log(addTwoNumbers(5,6));
Output:
11
Thus the number
type has been added to the end of the addTwoNumbers
, and thus it denotes that the return type of the function addTwoNumbers
is of type number
. TypeScript supports union and complex types as the return type of a function.
The following example shows an example of the union of types as the return type for a function.
function conditionalAdd(a : number, b : number ) : number | string {
if ( a + b > 20){
return "more than 20!";
} else {
return a + b;
}
}
console.log(conditionalAdd(3,21));
Output:
"more than 20!"
One can even have interfaces as the return type for a function. Suppose there is a function for returning all the config information.
It can be implemented as follows.
interface Config {
logLevel : string,
cache : boolean,
dbUrl : string,
password : string,
production : boolean,
version : number
}
function getConfig() : Config {
return {
logLevel : "debug",
cache : true,
dbUrl : "localhost:5432",
password : "postgres",
production : false,
version : 2
}
}
The benefit of using this approach is that one can directly return an object without declaring a variable and get suggestions during development.
Use the ReturnType
Type to Get the Return Type of a Function in TypeScript
The ReturnType
type is useful to know the type of a function, which may or may not be authored by the user as it may be in a third-party library. The following code segment shows how the ReturnType
type can detect the return type.
function findStudentWithRoll(roll : number){
const students : Student[] = [
{
name : 'Tintin',
roll : 1,
passed : true
},
{
name : 'Haddock',
roll : 2,
passed : false
},
{
name : 'Calculus',
roll : 3,
passed : true
},
];
return students.find( student => student.roll === roll) || null;
}
const studentRoll_1 : ReturnType<typeof findStudentWithRoll> = findStudentWithRoll(1);
if ( studentRoll_1 !== null){
console.log(studentRoll_1);
}
Output:
{
"name": "Tintin",
"roll": 1,
"passed": true
}
Thus the ReturnType<typeof findStudentWithRoll>
conveniently returns Student | null
which is further used for writing application logic.