Typed Functions as Function Parameters in TypeScript
- Use Typed Callbacks in Functions in TypeScript
- Typed Functions as Parameters Using Interface in TypeScript
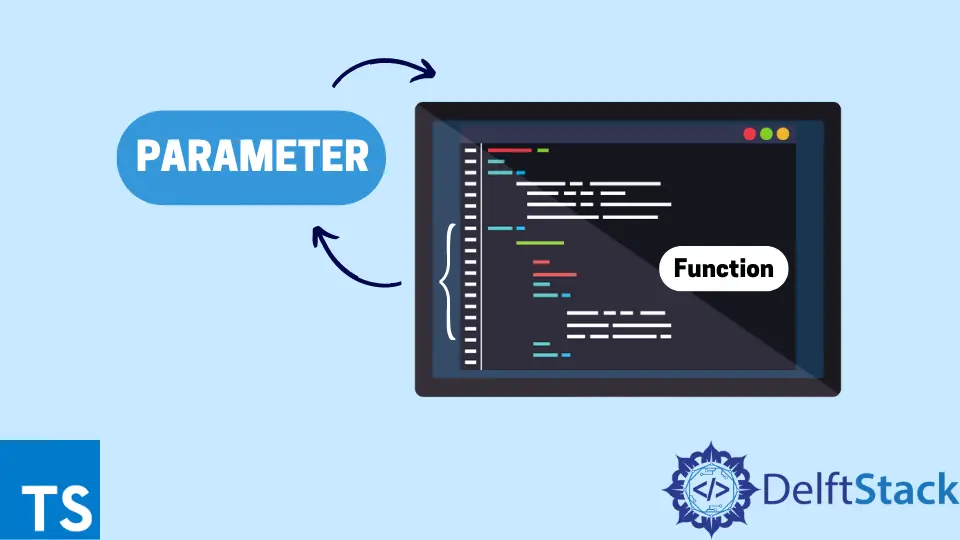
In TypeScript, along with different variables consisting of different types, strongly and weakly typed functions can also be passed along as function parameters in other functions.
This is owing to the functional programming paradigm of JavaScript that different functions can be passed on as parameters of other functions, TypeScript ensuring those functions are being typed. This tutorial will demonstrate how to use typed functions as function parameters.
Use Typed Callbacks in Functions in TypeScript
Different kinds of typed callbacks can be used in function definitions. The callbacks can have different types and act as parameters for different functions.
The following code block shows a demonstration of this.
enum LogLevel {
DEBUG,
INFO,
DANGER
}
interface LogFunction {
(message : string, level? : LogLevel) : void
}
function showLogMessage(message : string, level : LogLevel = 5) {
switch(level){
case LogLevel.INFO:
console.info("Message : " , message);
break;
case LogLevel.DEBUG:
console.debug("Message : " , message);
break;
case LogLevel.DANGER:
console.error("Message : " , message);
break;
default :
console.log("Message : " , message);
}
}
class Compute {
compute(callback :LogFunction) {
// Some Computation
var a = 5 + 6;
callback('Computed value of a : ' + a);
}
}
var computeObj = new Compute();
computeObj.compute(showLogMessage);
Output:
"Message : ", "Computed value of a : 11"
The above shows how typed functions can be used as function parameters of other functions. The Compute
class has the compute
function, which takes in a callback
of the type LogFunction
and calls the callback after some computation.
Any implementation of the callback
can be used while calling the function compute
. For this implementation, showLogMessage
is used to implement the interface LogFunction
.
Typed Functions as Parameters Using Interface in TypeScript
The functions passed in as parameters to different functions can have the types defined in the function definition, or an interface can be used to define the type of the function.
The following code blocks will show different types of typing that can be achieved while passing functions as parameters in other functions.
class Animal {
name : string;
constructor( name : string){
this.name = name;
}
showInfo(speak : () => void){
console.log(this.name);
speak();
}
}
var dog = new Animal('Dog');
dog.showInfo(() => console.log("Bark"));
Output:
"Dog"
"Bark"
The above example shows the inplace type definition of the speak
callback function passed on to the showInfo
function. The speak
function can also have an interface as its definition.
interface SpeakCallBackFn {
() : void
}
class Animal {
name : string;
constructor( name : string){
this.name = name;
}
showInfo(speak : SpeakCallBackFn){
console.log(this.name);
speak();
}
}
var cat = new Animal('Cat');
cat.showInfo(() => console.log("Meow"));
Output:
"Cat"
"Meow"
Thus, the above shows the implementation of the function typed using an interface.