TypeScript 中函式的返回型別
Shuvayan Ghosh Dastidar
2023年1月30日
TypeScript
TypeScript Function
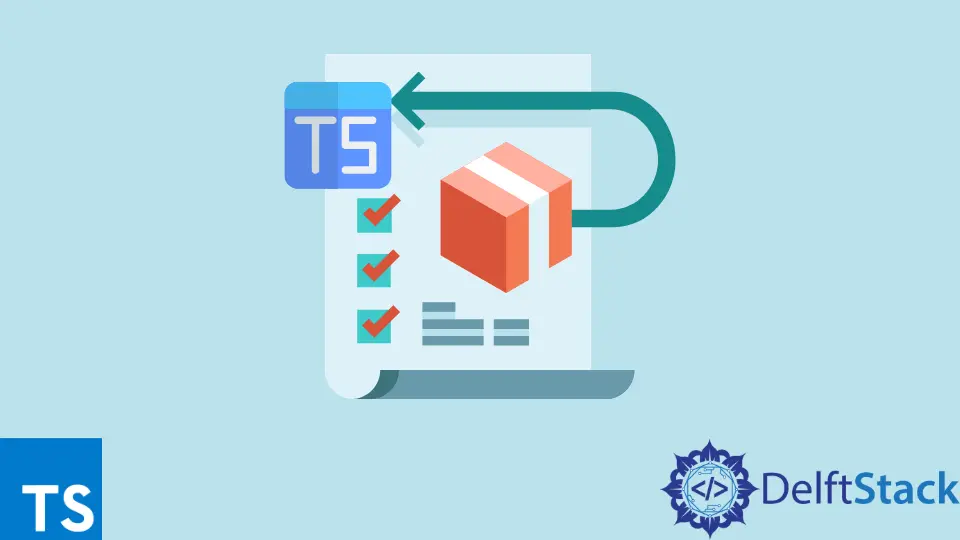
TypeScript 是一種強型別語言,始終鼓勵為 TypeScript 中使用的所有變數正確定義型別。這些型別可以在以後幫助開發,尤其是在除錯期間。
除了變數之外,函式還可以具有返回型別的型別。通常,當未定義型別時,編譯器會根據返回的變數型別自動推斷型別。
但是,這很不方便,並且可能會導致像 any
甚至 undefined
這樣的不太嚴格的型別作為返回型別,因此最好將型別返回給 TypeScript 函式。
在 TypeScript 的函式中為 return
語句使用型別
如果未提及任何型別,TypeScript 編譯器會自動推斷返回型別。最好向函式新增型別以確保通過應用程式程式碼傳遞正確的型別。
下面展示瞭如何將型別新增到 TypeScript 函式中。
function addTwoNumbers( a : number, b : number) : number {
return a + b;
}
console.log(addTwoNumbers(5,6));
輸出:
11
因此 number
型別已新增到 addTwoNumbers
的末尾,因此它表示函式 addTwoNumbers
的返回型別是 number
型別。TypeScript 支援聯合和複雜型別作為函式的返回型別。
以下示例顯示了型別聯合作為函式的返回型別的示例。
function conditionalAdd(a : number, b : number ) : number | string {
if ( a + b > 20){
return "more than 20!";
} else {
return a + b;
}
}
console.log(conditionalAdd(3,21));
輸出:
"more than 20!"
甚至可以將介面作為函式的返回型別。假設有一個返回所有配置資訊的函式。
它可以如下實現。
interface Config {
logLevel : string,
cache : boolean,
dbUrl : string,
password : string,
production : boolean,
version : number
}
function getConfig() : Config {
return {
logLevel : "debug",
cache : true,
dbUrl : "localhost:5432",
password : "postgres",
production : false,
version : 2
}
}
使用這種方法的好處是可以直接返回物件而無需宣告變數並在開發過程中獲得建議。
在 TypeScript 中使用 ReturnType
型別獲取函式的返回型別
ReturnType
型別對於瞭解函式的型別很有用,該函式可能由使用者編寫,也可能不由使用者編寫,因為它可能在第三方庫中。以下程式碼段顯示了 ReturnType
型別如何檢測返回型別。
function findStudentWithRoll(roll : number){
const students : Student[] = [
{
name : 'Tintin',
roll : 1,
passed : true
},
{
name : 'Haddock',
roll : 2,
passed : false
},
{
name : 'Calculus',
roll : 3,
passed : true
},
];
return students.find( student => student.roll === roll) || null;
}
const studentRoll_1 : ReturnType<typeof findStudentWithRoll> = findStudentWithRoll(1);
if ( studentRoll_1 !== null){
console.log(studentRoll_1);
}
輸出:
{
"name": "Tintin",
"roll": 1,
"passed": true
}
因此,ReturnType<typeof findStudentWithRoll>
方便地返回 Student | null
進一步用於編寫應用程式邏輯。
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe