How to Create Table in React TypeScript
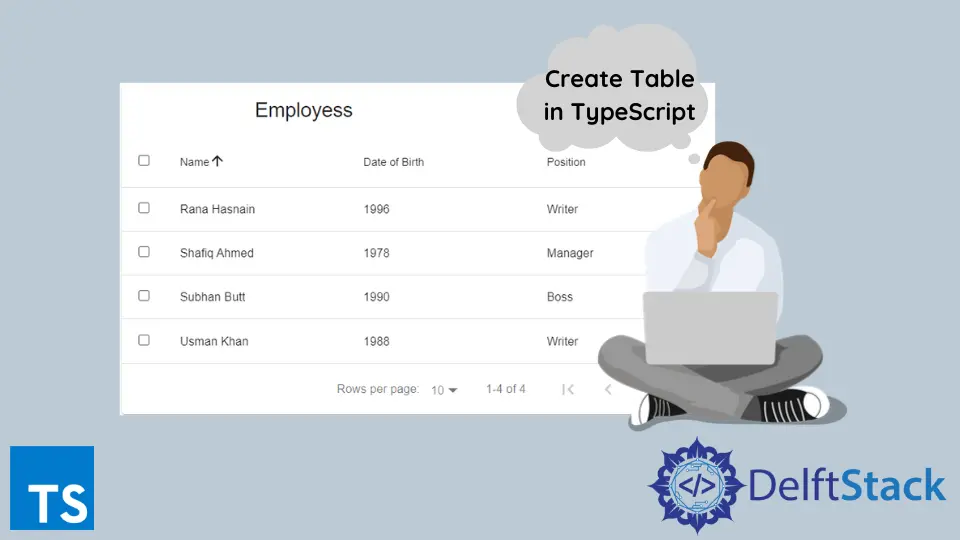
In this tutorial, we will create a data table in React using the JavaScript class for sample data.
Create Table in React TypeScript
Tables are an important part of any management system or modern software solutions used to manage different tasks in small to large firms. Tables are the best way to explain complex data easily.
Data tables are a type of table built with extra features such as filtering, searching, and sorting. Tables can explain a large amount of complex data in a short time.
React is a JavaScript library widely used to build user interfaces and the frontend of applications. We can use React with both JavaScript and TypeScript.
We must install all the dependencies to create a data table in React. But first, we will install react-dom
using the below command.
# react
npm i react-dom
Once we have installed react-dom
, we will install the react-data-table-component
using the following command.
# react
npm i react-data-table-component
We will install the @material-ui
in our react application using the following command.
# react
npm i material-ui
We will import all the dependencies inside our index.tsx
.
# react
import React from "react";
import ReactDOM from "react-dom";
import DataTable from "react-data-table-component";
import Card from "@material-ui/core/Card";
import SortIcon from "@material-ui/icons/ArrowDownward";
import "./styles.css";
Now, let’s create a constant for our sample data of users, as shown below.
# react
const data = [
{
id: 1,
name: "Rana Hasnain",
dob: "1996",
position: "Writer"
},
{
id: 2,
name: "Usman Khan",
dob: "1988",
position: "Writer"
},
{
id: 3,
name: "Shafiq Ahmed",
dob: "1978",
position: "Manager"
},
{
id: 4,
name: "Subhan Butt",
dob: "1990",
position: "Boss"
}
];
Then, we’ll define the columns of our data tables.
# react
const columns = [
{
name: "Name",
selector: "name",
sortable: true
},
{
name: "Date of Birth",
selector: "dob",
sortable: true
},
{
name: "Position",
selector: "position",
sortable: true
}
];
Inside our function, we will return the view of the table with the variables.
# react
function App() {
return (
<div className="App">
<Card>
<DataTable
title="Employess"
columns={columns}
data={data}
defaultSortField="name"
sortIcon={<SortIcon />}
pagination
selectableRows
/>
</Card>
</div>
);
}
Output:
We see from the above example that by using the material UI and react table components, we can easily create a data table of any data.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn