How to Convert Int to String in Scala
-
Using the
toString
Method - Using String Interpolation
-
Using
String.valueOf
-
Using
String.format
- Conclusion
- FAQ
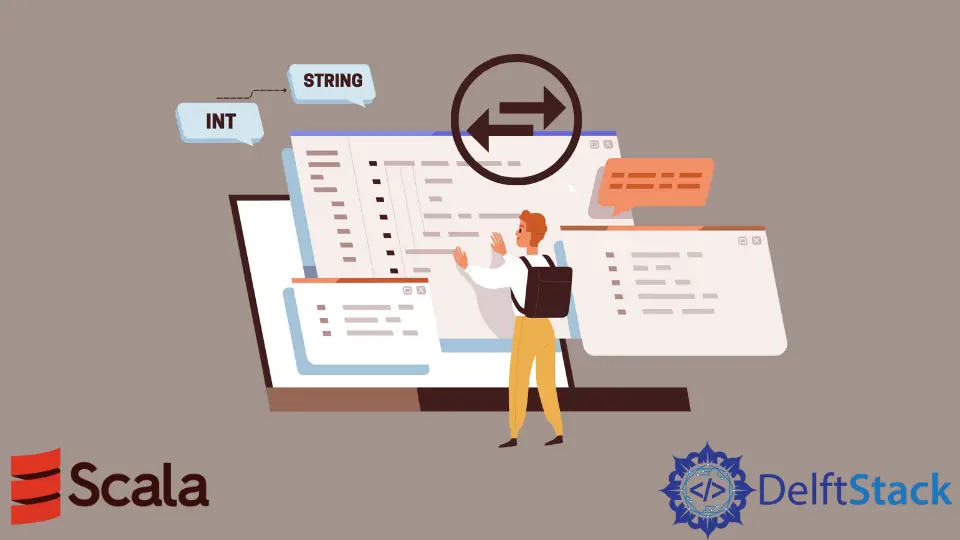
Converting integers to strings in Scala is a fundamental task that developers often encounter. Whether you’re preparing data for output, building user interfaces, or simply need to manipulate strings, knowing how to perform this conversion efficiently is crucial.
This article will guide you through several methods to convert an integer to a string in Scala. We’ll explore various approaches, including the built-in methods and more advanced techniques, ensuring you have a comprehensive understanding of this essential operation. By the end of this article, you’ll be equipped with the knowledge to handle integer-to-string conversions seamlessly in your Scala projects.
Using the toString
Method
One of the most straightforward ways to convert an integer to a string in Scala is by using the toString
method. Every integer in Scala is an instance of the Int
class, which provides this method for conversion. Here’s how you can do it:
val number: Int = 42
val stringRepresentation: String = number.toString
println(stringRepresentation)
Output:
42
In this example, we declare an integer variable named number
and assign it the value of 42. We then call the toString
method on this integer, which converts it into its string representation. The result is stored in stringRepresentation
, and when we print it, we see the output as “42”. This method is simple and effective, making it a popular choice among Scala developers.
Using String Interpolation
Another elegant way to convert an integer to a string in Scala is through string interpolation. This approach is not only concise but also enhances readability. String interpolation allows you to embed variables directly within a string literal. Here’s how you can achieve this:
val number: Int = 42
val stringRepresentation: String = s"The number is: $number"
println(stringRepresentation)
Output:
The number is: 42
In this code snippet, we define an integer number
with the value of 42. Using the string interpolation feature with the s
prefix, we create a new string that includes the integer. The variable number
is seamlessly embedded into the string, resulting in “The number is: 42”. This method is particularly useful when you want to create a more descriptive string while converting an integer.
Using String.valueOf
Scala also provides the Java method String.valueOf
to convert integers to strings. This method is versatile and can be used for various data types, including integers. Here’s how to implement it:
val number: Int = 42
val stringRepresentation: String = String.valueOf(number)
println(stringRepresentation)
Output:
42
In this example, we use String.valueOf
to convert the integer number
into a string. The method is straightforward and works similarly to the toString
method. It’s a good alternative, especially if you are already familiar with Java, as it provides a consistent way to handle type conversions across both languages.
Using String.format
For cases where you need formatted strings, String.format
is an excellent choice. This method allows you to specify the format of the output string, which can be particularly useful for creating user-friendly messages. Here’s how to use it:
val number: Int = 42
val stringRepresentation: String = String.format("The number is: %d", number)
println(stringRepresentation)
Output:
The number is: 42
In this code, we utilize String.format
to create a formatted string. The %d
placeholder is replaced with the integer value of number
. This method is beneficial when you need to format the output in a specific way, such as padding or aligning numbers. It provides more control over the string representation, making it a valuable tool in your Scala programming toolkit.
Conclusion
Converting integers to strings in Scala is a straightforward process, with several methods available to suit different needs. From the simple toString
method to the elegant string interpolation and the versatile String.valueOf
, you have various options at your disposal. Each method has its unique advantages, allowing you to choose the one that best fits your coding style and requirements. By mastering these techniques, you’ll enhance your ability to manipulate data effectively in Scala, making your programming experience smoother and more efficient.
FAQ
-
What is the simplest way to convert an integer to a string in Scala?
The simplest way is to use thetoString
method, which is available on integer variables. -
Can I use string interpolation to convert an integer to a string?
Yes, string interpolation is a concise and readable way to embed an integer within a string. -
Is
String.valueOf
a good alternative for converting integers?
Yes,String.valueOf
is a versatile method that works well for converting various data types, including integers. -
When should I use
String.format
for conversion?
UseString.format
when you need to create formatted strings, especially if you want to control the output format. -
Are these conversion methods specific to Scala, or can they be used in Java as well?
Some methods, likeString.valueOf
andString.format
, are also available in Java, making them familiar to Java developers.