How to Concatenate Strings in Scala
- Using the + Operator
- Using String Interpolation
- Using the mkString Method
- Using the StringBuilder Class
- Conclusion
- FAQ
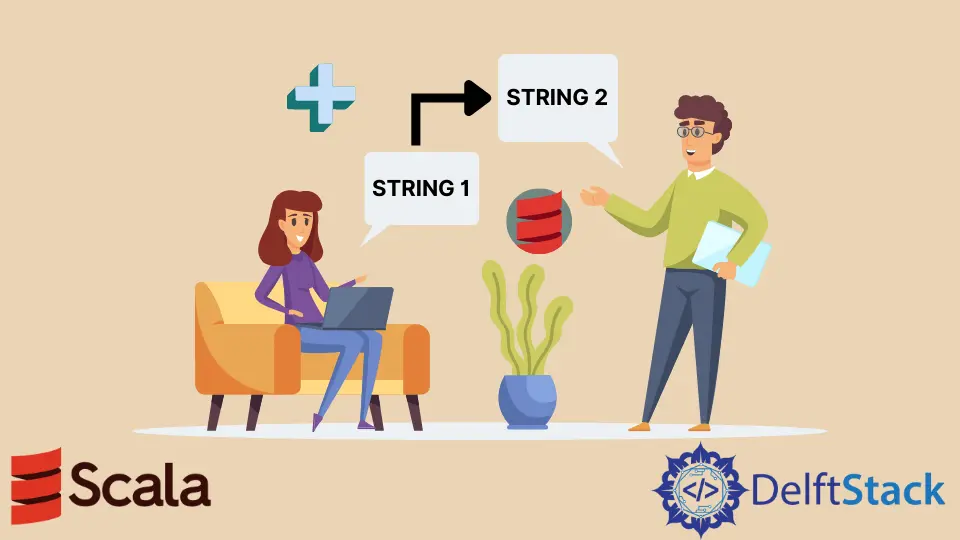
Concatenating strings in Scala is a fundamental skill that every developer should master. Whether you’re building a web application, processing data, or simply manipulating text, knowing how to effectively combine strings can save you time and effort.
In this article, we will explore various methods for concatenating strings in Scala, providing clear examples and explanations for each. By the end, you’ll have a solid understanding of how to work with strings in Scala, enhancing your programming toolkit. Let’s dive into the world of string manipulation and see how easy it is to concatenate strings in this powerful programming language.
Using the + Operator
The simplest way to concatenate strings in Scala is by using the +
operator. This method is intuitive and resembles how you might combine strings in other programming languages. Here’s a quick example:
val firstName = "John"
val lastName = "Doe"
val fullName = firstName + " " + lastName
println(fullName)
Output:
John Doe
In this example, we define two string variables, firstName
and lastName
. By using the +
operator, we can easily concatenate these strings with a space in between. The resulting string, fullName
, is then printed to the console. This method is straightforward and works well for simple concatenation tasks. However, as the number of strings increases, using the +
operator repeatedly can become less efficient, leading us to explore other techniques.
Using String Interpolation
String interpolation is a powerful feature in Scala that allows you to embed variables directly within a string. This can make your code cleaner and more readable. To use string interpolation, prefix your string with the letter s
. Here’s how it works:
val firstName = "Jane"
val lastName = "Smith"
val fullName = s"$firstName $lastName"
println(fullName)
Output:
Jane Smith
In this code snippet, we utilize string interpolation to create the fullName
variable. The s
before the string allows us to include the values of firstName
and lastName
directly within the string. This method is not only concise but also enhances readability, especially when dealing with multiple variables. String interpolation is particularly useful when constructing longer strings or when you want to include expressions or calculations within your strings.
Using the mkString Method
For cases where you have a collection of strings that you want to concatenate, the mkString
method is an excellent choice. This method is part of the Seq
trait and can be used with arrays, lists, and other collections. Here’s an example:
val names = List("Alice", "Bob", "Charlie")
val concatenatedNames = names.mkString(", ")
println(concatenatedNames)
Output:
Alice, Bob, Charlie
In this example, we create a list of names and use the mkString
method to concatenate them into a single string, separated by commas. The mkString
method is highly versatile; you can specify different separators or even wrap the entire output in a prefix and suffix. For instance, names.mkString("[", ", ", "]")
would produce a string formatted like “[Alice, Bob, Charlie]”. This method is particularly useful for processing collections of strings, making it a favorite among Scala developers.
Using the StringBuilder Class
When dealing with large amounts of string concatenation, using the StringBuilder
class can greatly improve performance. Unlike the +
operator, which creates a new string each time you concatenate, StringBuilder
allows you to modify a single instance, making it more efficient. Here’s how to use it:
val stringBuilder = new StringBuilder
stringBuilder.append("Hello")
stringBuilder.append(" ")
stringBuilder.append("World")
val result = stringBuilder.toString()
println(result)
Output:
Hello World
In this code, we create an instance of StringBuilder
and use the append
method to add strings. Finally, we convert the StringBuilder
back to a string using the toString
method. This approach is particularly beneficial when you have multiple strings to concatenate in a loop or when building complex strings dynamically. By minimizing the creation of intermediate strings, StringBuilder
can significantly enhance performance in scenarios where string concatenation is a bottleneck.
Conclusion
In summary, concatenating strings in Scala is a straightforward task with multiple methods available to suit different needs. Whether you opt for the simplicity of the +
operator, the elegance of string interpolation, the convenience of mkString
, or the performance benefits of StringBuilder
, Scala provides you with the tools to handle string manipulation effectively. By mastering these techniques, you can enhance your coding skills and write more efficient, readable code. So go ahead and experiment with these methods in your projects, and watch your string concatenation skills flourish!
FAQ
-
What is the simplest way to concatenate strings in Scala?
The simplest way is to use the+
operator to combine strings. -
How does string interpolation work in Scala?
String interpolation allows you to embed variables directly within a string using thes
prefix. -
What is the advantage of using the
mkString
method?
ThemkString
method is useful for concatenating collections of strings with specified separators. -
When should I use
StringBuilder
for string concatenation?
UseStringBuilder
when you need to concatenate a large number of strings or perform concatenation in a loop for better performance. -
Can I concatenate strings in Scala without creating new instances?
Yes, usingStringBuilder
allows you to modify a single instance for more efficient string building.