How to Compare Strings in Scala
-
Compare Strings Using the
equals()
Method in Scala -
Compare Strings Using the
==
Operator in Scala - Equality in Java and Scala
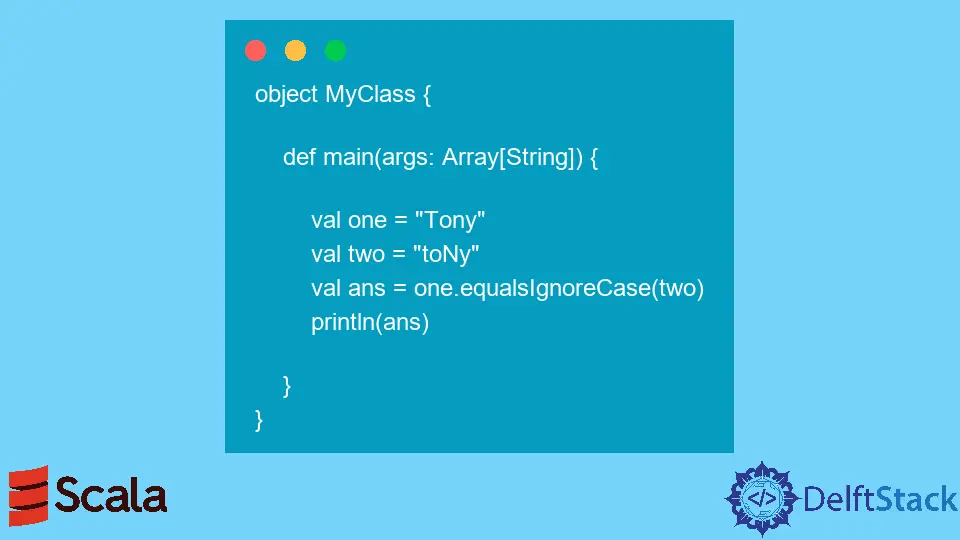
In this article, we will learn how to compare two strings in the Scala programming language.
Two strings are considered equal if they have the same sequence of characters. Now, let’s look at different approaches in Scala to compare strings.
Compare Strings Using the equals()
Method in Scala
In Scala, the String
class has the equals()
method to check for the equality of two strings.
Syntax:
string_one.equals(string_two)
The function returns true
if both string_one
and string_two
are equal.
Example code:
object MyClass {
def main(args: Array[String]) {
val one = "Tony"
val two = "Tony"
val ans = one.equals(two)
println(ans)
}
}
Output:
true
One thing to note is that the equals()
method is case-sensitive, meaning tony
and Tony
are treated differently. To overcome this, we have the equalsIgnoreCase()
method in the String
class, which we can use.
Example code:
object MyClass {
def main(args: Array[String]) {
val one = "Tony"
val two = "toNy"
val ans = one.equalsIgnoreCase(two)
println(ans)
}
}
Output:
true
equals()
function of the String
class (like string one
in the above code) should not be null
; else, we will get a NullPointerException
.Compare Strings Using the ==
Operator in Scala
We can use the ==
method to compare two strings in Scala.
Syntax:
string_one == string_two
The ==
method checks the equality of string_one
and string_two
. It returns true
if both strings are equal; otherwise, it returns false
.
Example code:
object MyClass {
def main(args: Array[String]) {
val one = "tony"
val two = "tony"
if(one==two)
println(true)
else
println (false)
}
}
Output:
true
If we know that the strings are case-sensitive, one major advantage of using the ==
over equals()
method is that it will not throw NullPointerException
even if one of the strings is null
.
Example code:
object MyClass {
def main(args: Array[String]) {
val one = "tony"
if(one == null)
println(true)
else
println (false)
}
}
Output:
false
In the back-end, the ==
method first checks for null
values, then calls the equals()
method with the first string object to check for their equality. Because of this reason, the ==
method doesn’t compare strings in a case-sensitive manner.
Example code:
object MyClass {
def main(args: Array[String]) {
val one = "tony"
val two = "TONY"
if(one == two)
println(true)
else
println (false)
}
}
Output:
false
To overcome this situation of case sensitivity, we can either convert both the strings to uppercase or lowercase and then compare them.
Example code:
object MyClass {
def main(args: Array[String]) {
val one = "tony"
val two = "TONY"
if(one.toLowerCase() == two.toLowerCase())
println(true)
else
println (false)
}
}
Output:
true
In the above code, we have converted both the strings to lower case using the toLowerCase()
method, and then we used the ==
method to compare them.
Equality in Java and Scala
Java and Scala have different definitions when it comes to equality. Java mainly has two ways to check for equality: one is using the ==
operator, which strictly checks for reference
equality, and another is the equals()
method which can be overridden to check for the contents of the objects.
In Scala, the ==
method can be used to compare any type, meaning a==b
will return true
if they refer to the same object or contain the same value. And in Scala, the ==
method is a final
method defined in any class.