How to Read and Write Files in Rust
- Read a File to the String in Rust 1.26 and Onwards
-
to Read a File as a
Vec<u8>
in Rust 1.26 and Onwards - Write a File in Rust in Rust 1.26 and Onwards
- Read a File to a String in Rust 1.0
-
to Read a File as a
Vec<u8>
in Rust 1.0 - Create a Function in Rust
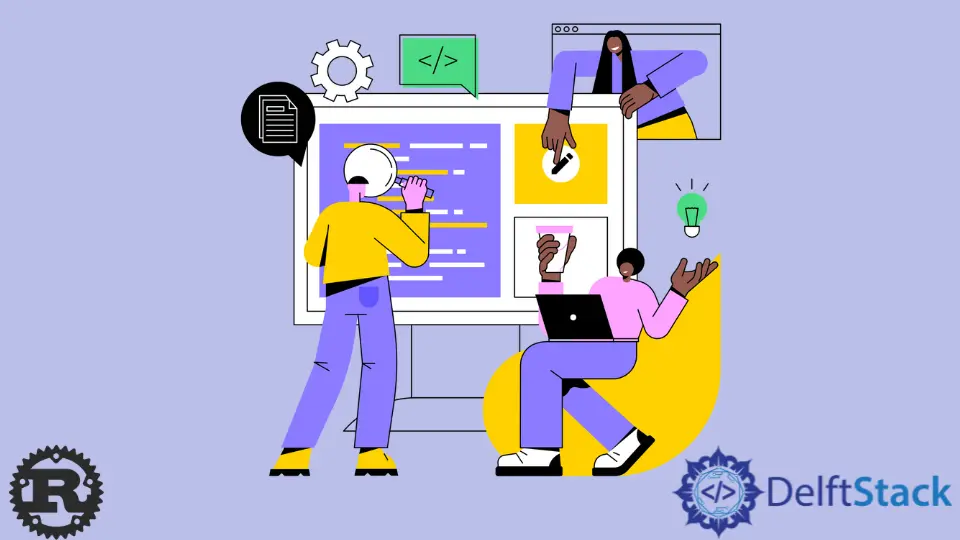
We’ll learn how to read and write files in different versions of Rust using various techniques in this topic. Learning about error handing will be easier to understand and handle errors in the program.
One-line functions are available for reading and writing files in Rust 1.26
and onwards.
Read a File to the String in Rust 1.26 and Onwards
use std::fs;
fn main() {
let info = fs::read_to_string("/etc/hosts").expect("The file could not be read");
println!("{}", info);
}
The std::fs
module includes basic techniques for manipulating the contents of the local file system. The read_to_string()
function opens files with fewer imports
excluding intermediate variables.
Its working mechanism is the same as File::open
. We’ll store the contents from file-/etc/hosts
into info
and then print it.
Output:
128.127.0.0.1 localhost
::1 localhost ip6-localhost ip6-loopback
fe00::0 ip6-localnet
ff00::0 ip6-mcastprefix
ff02::1 ip6-allnodes
ff02::2 ip6-allrouters
to Read a File as a Vec<u8>
in Rust 1.26 and Onwards
Vec
is the short form of Vector
, and u8
refers to the 8-bit unsigned integer type. The below code is used for reading the file as a Vec<u8>
.
use std::fs;
fn main() {
let info = fs::read("/etc/hosts").expect("The file could not be read");
println!("{}", info.len());
}
Output:
150
Write a File in Rust in Rust 1.26 and Onwards
Now, let’s look at an example of writing a file using Rust 1.26.
use std::fs::File;
use std::io::{Write, BufReader, BufRead, Error};
fn main() -> Result<(), Error> {
let path = "/home/user/words.txt";
let mut output = File::create(path)?;
write!(output, "Tick\n\nToe")?;
let input = File::open(path)?;
let buffered = BufReader::new(input);
for words in buffered.lines() {
println!("{}", words?);
}
Ok(())
}
In the above code, File::create
opens a file for writing, and File::open
is used for reading. BufRead
has an internal buffer to read a file and is more useful to read line by line.
The buffer reader or writer uses a buffer to reduce I/O requests, and it is substantially more practical to access the disk once for reading 256 bytes than accessing the same disk 256 times. The std::io
module offers a variety of useful features for doing input and output, such as Read
and Write
.
Output:
Tick
Toe
Read a File to a String in Rust 1.0
These methods are more verbose than the one-line functions that allocate a String
or a Vec
. However, they are more powerful since the allocated data may be reused or added to an existing object.
use std::fs::File;
use std::io::Read;
fn main() {
let mut info = String::new();
let mut f = File::open("/etc/hosts").expect("The file cannot be opened");
f.read_to_string(&mut data).expect("The string cannot be read");
println!("{}", info);
}
The mut
keyword refers to mutable, File::open
opens the file in path /etc/hosts
. The above code instructs to read a file to a string, and expect
is used for error handling.
Output:
128.127.0.0.1 localhost
::1 localhost ip6-localhost ip6-loopback
fe00::0 ip6-localnet
ff00::0 ip6-mcastprefix
ff02::1 ip6-allnodes
ff02::2 ip6-allrouters
to Read a File as a Vec<u8>
in Rust 1.0
use std::fs::File;
use std::io::Read;
fn main() {
let mut info = Vec::new();
let mut f = File::open("/etc/hosts").expect("The file cannot be opened");
f.read_to_end(&mut info).expect("The information cannot be read");
println!("{}", info.len());
}
The read_to_end()
copies data in big chunks. Thus, the transfer can naturally merge into fewer I/O operations.
Output:
150
Create a Function in Rust
The create function is used for opening the file in write-only mode. If the file already exists, the previous content is overwritten, and a new one is generated.
use std::fs::File;
use std::io::Write;
fn main() {
let info = "This is a sentence that exists in file.";
let mut f = File::create("words.txt")
.expect("unable to create file");
f.write_all(info.as_bytes()).expect("Could not write");
}
Output:
We then use the cat
command to read text from the words.txt
.
$ cat words.txt
Output:
This is a sentence that exists in file.