用 Rust 读写文件
- 在 Rust 1.26 及更高版本中读取文件到字符串
-
在 Rust 1.26 及更高版本中将文件读取为
Vec<u8>
- 在 Rust 1.26 及更高版本中用 Rust 编写文件
- 在 Rust 1.0 中读取文件到字符串
-
在 Rust 1.0 中将文件读取为
Vec<u8>
- 在 Rust 中创建一个函数
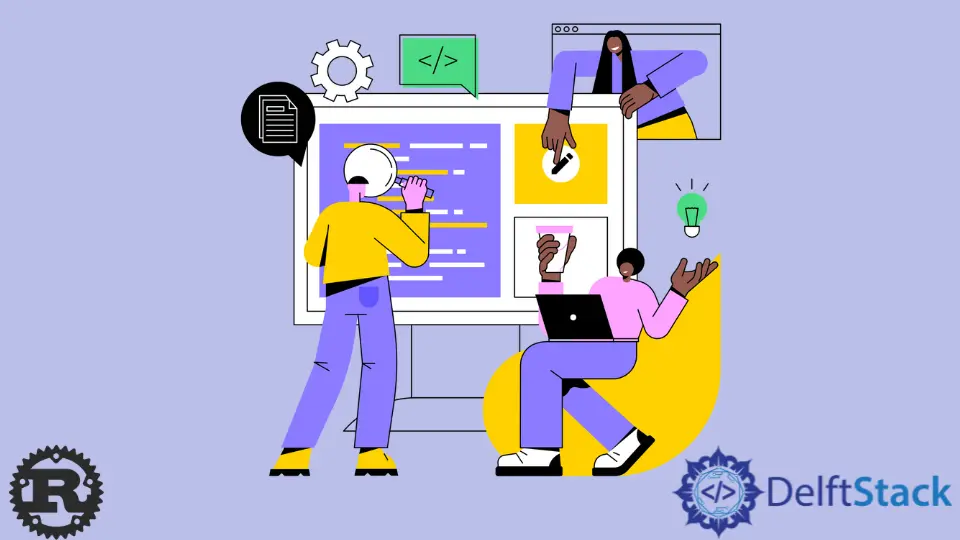
我们将在本主题中学习如何使用各种技术在不同版本的 Rust 中读取和写入文件。学习错误处理会更容易理解和处理程序中的错误。
在 Rust 1.26
及更高版本中,单行函数可用于读取和写入文件。
在 Rust 1.26 及更高版本中读取文件到字符串
use std::fs;
fn main() {
let info = fs::read_to_string("/etc/hosts").expect("The file could not be read");
println!("{}", info);
}
std::fs
模块包括操作本地文件系统内容的基本技术。read_to_string()
函数打开具有较少 imports
的文件,不包括中间变量。
其工作机制与 File::open
相同。我们会将文件-/etc/hosts
中的内容存储到 info
中,然后打印出来。
输出:
128.127.0.0.1 localhost
::1 localhost ip6-localhost ip6-loopback
fe00::0 ip6-localnet
ff00::0 ip6-mcastprefix
ff02::1 ip6-allnodes
ff02::2 ip6-allrouters
在 Rust 1.26 及更高版本中将文件读取为 Vec<u8>
Vec
是 Vector
的简写形式,u8
指的是 8 位无符号整数类型。下面的代码用于将文件作为 Vec<u8>
读取。
use std::fs;
fn main() {
let info = fs::read("/etc/hosts").expect("The file could not be read");
println!("{}", info.len());
}
输出:
150
在 Rust 1.26 及更高版本中用 Rust 编写文件
现在,让我们看一个使用 Rust 1.26 编写文件的示例。
use std::fs::File;
use std::io::{Write, BufReader, BufRead, Error};
fn main() -> Result<(), Error> {
let path = "/home/user/words.txt";
let mut output = File::create(path)?;
write!(output, "Tick\n\nToe")?;
let input = File::open(path)?;
let buffered = BufReader::new(input);
for words in buffered.lines() {
println!("{}", words?);
}
Ok(())
}
在上面的代码中,File::create
打开一个文件用于写入,File::open
用于读取。BufRead
有一个内部缓冲区来读取文件,并且对于逐行读取更有用。
缓冲区读取器或写入器使用缓冲区来减少 I/O 请求,并且访问一次磁盘以读取 256 字节比访问同一磁盘 256 次要实用得多。std::io
模块提供了多种有用的功能来进行输入和输出,例如 Read
和 Write
。
输出:
Tick
Toe
在 Rust 1.0 中读取文件到字符串
这些方法比分配 String
或 Vec
的单行函数更冗长。但是,它们更强大,因为分配的数据可以重用或添加到现有对象。
use std::fs::File;
use std::io::Read;
fn main() {
let mut info = String::new();
let mut f = File::open("/etc/hosts").expect("The file cannot be opened");
f.read_to_string(&mut data).expect("The string cannot be read");
println!("{}", info);
}
mut
关键字是指可变的,File::open
打开路径/etc/hosts
中的文件。上面的代码指示将文件读取为字符串,而 expect
用于错误处理。
输出:
128.127.0.0.1 localhost
::1 localhost ip6-localhost ip6-loopback
fe00::0 ip6-localnet
ff00::0 ip6-mcastprefix
ff02::1 ip6-allnodes
ff02::2 ip6-allrouters
在 Rust 1.0 中将文件读取为 Vec<u8>
use std::fs::File;
use std::io::Read;
fn main() {
let mut info = Vec::new();
let mut f = File::open("/etc/hosts").expect("The file cannot be opened");
f.read_to_end(&mut info).expect("The information cannot be read");
println!("{}", info.len());
}
read_to_end()
以大块的形式复制数据。因此,传输可以自然地合并为更少的 I/O 操作。
输出:
150
在 Rust 中创建一个函数
create 函数用于以只写模式打开文件。如果文件已经存在,则覆盖之前的内容,并生成一个新的。
use std::fs::File;
use std::io::Write;
fn main() {
let info = "This is a sentence that exists in file.";
let mut f = File::create("words.txt")
.expect("unable to create file");
f.write_all(info.as_bytes()).expect("Could not write");
}
输出:
然后我们使用 cat
命令从 words.txt
中读取文本。
$ cat words.txt
输出:
This is a sentence that exists in file.