Difference Between Rc::clone(&rc) and rc.clone() in Rust
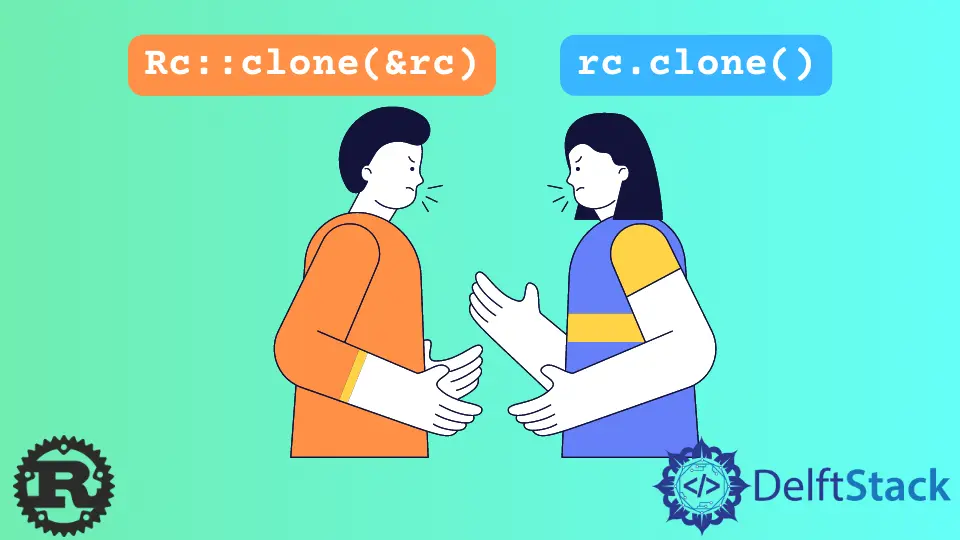
In this article we’ll tackle about the difference between Rc::clone(&rc)
and rc.clone()
in Rust. Both of the traits are used for cloning or duplicating an object.
Use Rc::clone(&rc)
for Cloning in Rust
Rc::clone(&rc)
is used to make one new shared reference instead of cloning the underlying object referenced. Rc
stands for Reference Counted
, and the shared ownership of type T
value is provided by the type Rc<T>
, allocated in a heap.
use std::fmt;
use std::rc::Rc;
fn print_it<T: fmt::Display>(val: T) {
println!("{}", val);
}
fn main(){
let greet = Rc::new("Hello, world".to_string());
let borrowed = Rc::clone(&greet);
print_it(greet);
print_it(borrowed);
}
Output:
Hello, world
Hello, world
We have displayed the scope of Rc
in the code written above by using the use
statement. Then, the Rc
type is created associated with the function::new()
with the original string.
Later a reference is created using another relative function::clone()
for the original greet:RC
(this is not the same as the initial reference, i.e., &greet
). We have tried to print both referenced and an original variable using this code’s function print_it
.
The execution of Rc::clone
does not make a deep copy of the data like most types of executions of clones do. Data’s deep copy can take a great deal of time.
By involving Rc::clone
for reference counting, we can recognize the deep copy type of clones and the type that increases the reference count. While searching for execution issues in the code, we need to consider the deep copy clones and ignore calls to Rc::clone
.
the rc.clone()
in Rust
The clone()
function creates a new owned handle, which can be moved to a new thread. According to the standard library of Rust, this function tends to make common code patterns easy to misinterpret.
The issue is that more-specific processes and types, which could advantage from detailed nomenclature, are only accessible through high-level or abstract terms.