How to Write Multi-Line String in Ruby
- Write Multi-Line String Straight Between Two Quotes in Ruby
-
Use the
+
Operator for Writing Multi-Line String in Ruby - Use the backslash for Writing Multi-Line String in Ruby
-
Use
heredoc
for Writing Multi-Line String in Ruby -
Use the
%
Operator Literal for Writing Multi-Line String in Ruby
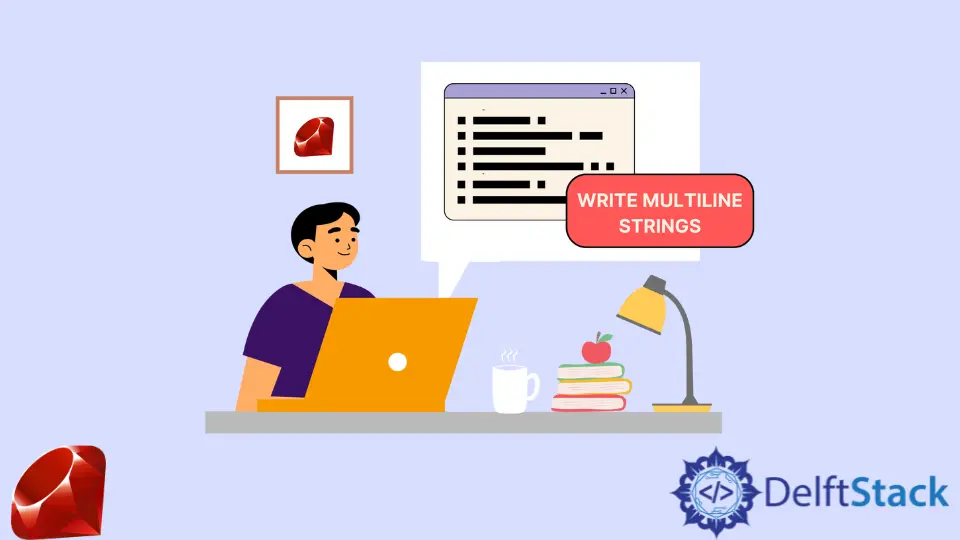
This article will discuss how to create a multi-line string in the Ruby programming language.
Write Multi-Line String Straight Between Two Quotes in Ruby
We can create a multi-line string by simply inserting a newline as long as our string remains between two quotes.
multiline = 'SELECT email, name
FROM users
WHERE is_single'
Output:
"SELECT email, name\n FROM users\n WHERE is_single"
>puts multi-line
SELECT email, name
FROM users
WHERE is_single
This approach preserves whitespaces and newline characters.
It’s possible to use single or double-quotes. We could do interpolation inside the string if we used a pair of double-quotes.
Use the +
Operator for Writing Multi-Line String in Ruby
The string concatenation operator is naive to write multi-line strings in Ruby. Unlike the above method, using +
does not preserve whitespaces or newlines.
multiline = 'SELECT email, name ' +
'FROM users ' +
'WHERE is_single'
Output:
"SELECT email, name FROM users WHERE is_single"
When spanning across lines, this method makes the code look untidy.
Use the backslash for Writing Multi-Line String in Ruby
The backslash is a line continuation, which indicates to the interpreter that the current line of code is not yet complete and will continue on the next line.
This behavior can be used to write multi-line strings in Ruby with the same results as string concatenation.
multiline = 'SELECT email, name ' \
'FROM users ' \
'WHERE is_single'
Output:
"SELECT email, name FROM users WHERE is_single"
Use heredoc
for Writing Multi-Line String in Ruby
heredoc
allows us to write a large string without worrying about adding a +
or a \
at the end of each line. It also maintains the indentations and format as the first solution.
The syntax begins with <<-
, continues with the document’s name content, and ends with the document’s name on a separate line.
multiline = <<~MY_DOC
SELECT email, name
FROM users
WHERE is_single
MY_DOC
Output:
"SELECT email, name\nFROM users\nWHERE is_single\n"
>puts multi-line
SELECT email, name
FROM users
WHERE is_single
MY_DOC
is the name of the document, it can be lowercase, but UPPERCASE is preferred.
heredoc
also allows interpolation.
Use the %
Operator Literal for Writing Multi-Line String in Ruby
Apart from the methods described above, we can use %{}
to create a multi-line lines string in Ruby.
It works in the same way as the first approach. Indentations and newlines are also preserved.
multiline = %(
SELECT email, name
FROM users
WHERE is_single
)
Output:
"\nSELECT email, name\nFROM users\nWHERE is_single\n"