How to Trim a String Without Creating a New String in Ruby
-
Use the
strip!
Method to Trim a String in Ruby -
Use the
lstrip!
Method to Trim a String in Ruby -
Use the
rstrip!
Method to Trim a String in Ruby -
Use the
gsub!
Method to Trim a String in Ruby - Conclusion
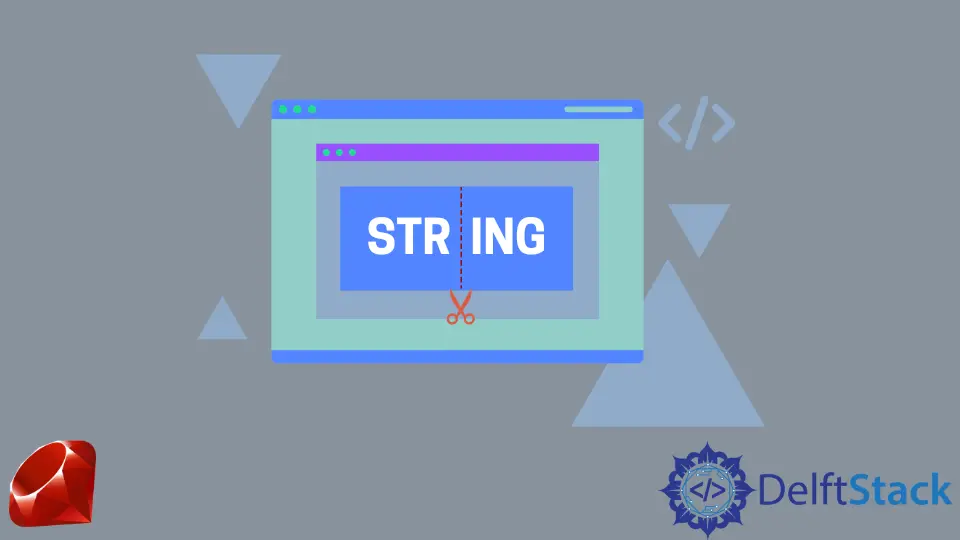
The String#strip
method serves as a valuable tool for eliminating leading and trailing whitespaces, producing a trimmed string. On the flip side, opting for the bang version, strip!
, allows for in-place modification and will help avoid the creation of a new string.
This guide explores the use of strip!
and related methods, such as lstrip!
, rstrip!
, and gsub!
, providing insights into trimming strings in various ways in Ruby.
Use the strip!
Method to Trim a String in Ruby
The strip!
will remove whitespace from the object. It would return to nil
if no changes were made.
In the following code, we use the strip!
method to trim leading and trailing whitespaces from the string " hello world "
. In this case, it trims the spaces before and after the actual phrase, resulting in the output "hello world"
.
The bang version of strip!
indicates that the modification is performed in-place, meaning it directly alters the original string without creating a new one. Therefore, the output of this code snippet is the trimmed string "hello world"
, showcasing the immediate impact of the strip!
method on the provided string.
puts ' hello world '.strip!
Output:
hello world
Please avoid assigning string = string.strip!
as this may result in an unexpected result when no changes to the string have been made.
string = 'hello world'
string = string.strip!
puts string
Output:
In this code, after initializing the string
variable with the value 'hello world'
, we apply the String#strip!
method. However, since the original string already has no leading or trailing whitespaces, the strip!
operation results in a nil
value.
Consequently, when we print the modified string, the result is a blank line. This emphasizes the importance of taking caution when applying strip!
to ensure the expected outcome; in this case, it may lead to unexpected results when no changes to the string are made.
Use the lstrip!
Method to Trim a String in Ruby
Alternatively, you can use String#lstrip!
to remove only the leading whitespaces.
str = ' Hello, World! '
str.lstrip!
puts str
Output:
Hello, World!
In this code, we work with a string variable, str
, initialized with the value " Hello, World! "
. We then apply the String#lstrip!
method, focusing on removing leading whitespaces exclusively.
The use of the bang version, lstrip!
, ensures that this modification is done in place, directly altering the original string. Finally, we print the result, which is the string "Hello, World! "
with the leading whitespaces successfully removed.
Use the rstrip!
Method to Trim a String in Ruby
You can also use the String#rstrip!
to remove trailing whitespaces.
str = 'Hello, World! '
str.rstrip!
print str
Output:
Hello, World!
In this code, we initialized a string variable str
with the value "Hello, World! "
. Employing the String#rstrip!
method, we exclusively target and remove trailing whitespaces.
The use of the bang version, rstrip!
, ensures that this modification occurs in place, directly altering the original string. Subsequently, we print the result, showcasing the "Hello, World!"
string without the trailing whitespaces.
Use the gsub!
Method to Trim a String in Ruby
However, it’s important to note that the strip!
method only removes whitespace. If you want to remove other characters, you will need to use other methods.
You can use the gsub!
method to remove all occurrences of a specific character.
str = 'Hello, World!'
str.gsub!(',', '')
puts str
Output:
Hello World!
In this code, we manipulate a string variable named str
, initialized with the value "Hello, World!"
. Using the String#gsub!
method, we target and remove all occurrences of the specified character—in this case, the comma.
The bang version, gsub!
, ensures this modification is done in place, directly altering the original string. Finally, we print the result, displaying the "Hello World!"
string with the removal of all commas.
Conclusion
When working with strings in Ruby, understanding the various methods for trimming and modifying strings is crucial. The String#strip
method provides a convenient way to create a new string with leading and trailing whitespaces removed.
For scenarios where modifying the original string without creating a new one is preferred, the String#strip!
, String#lstrip!
and String#rstrip!
methods can be employed. It’s essential to be cautious when using the bang version of these methods, as they return nil
when no changes are made, potentially leading to unexpected results.
Additionally, for more advanced string manipulation, like removing specific characters, the String#gsub!
method proves useful. Choosing the method depends on the specific requirements of the task at hand, allowing developers to efficiently handle string trimming and modifications in their Ruby applications.