How to Concatenate Strings in Ruby
- Use String Interpolation to Concatenate Strings in Ruby
-
Use
+
to Concatenate Strings in Ruby -
Use the
concat
Method to Concatenate Strings in Ruby -
Use the
Array.join
Method to Concatenate Strings in Ruby -
Use the
<<
Operator to Concatenate Strings in Ruby -
Use the
*
Operator to Concatenate Strings in Ruby - Conclusion
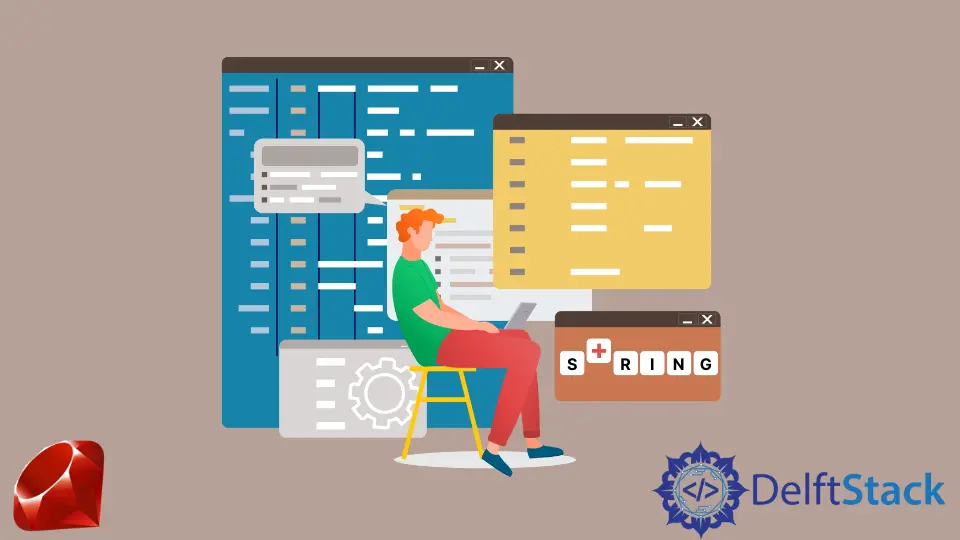
Concatenating strings in Ruby refers to the process of combining two or more strings to create a single string. This operation allows you to join different pieces of text or string variables into a unified string.
This article will introduce different ways to concatenate strings in Ruby.
Use String Interpolation to Concatenate Strings in Ruby
In Ruby, string interpolation is the most popular and elegant concatenating string. String interpolation is preferred over other methods of concatenation.
Syntax:
variable_name = 'some_value'
result = "#{variable_name} and additional text"
In this syntax, the #{}
is used to embed the value of the variable or expression within a string. The variable_name
is the name of the variable or expression you want to include, and the entire expression is enclosed in double quotation marks(" "
).
Example Code:
string_1 = 'hello'
puts "#{string_1} from the outside"
Output:
hello from the outside
In this code, we initialize a variable string_1
with the value 'hello'
. The puts
statement is then used to output a string that incorporates the value of string_1
using string interpolation within double quotes.
The syntax #{}
is employed to embed the content of string_1
into the larger string. Consequently, when this code is executed, the output will be hello from the outside
, as it effectively combines the original string value with the additional text specified in the interpolated string.
Use +
to Concatenate Strings in Ruby
We can also use the +
operator to join strings together.
In Ruby, the +
operator can be used to concatenate strings. This means that when the +
operator is applied to two strings, it combines them into a single string, creating a new string that contains the characters of both original strings in the specified order.
This operation allows for the creation of longer strings by joining together multiple shorter strings.
Example Code:
string_1 = 'players'
string_2 = 'gonna play'
puts string_1 + ' ' + string_2
Output:
players gonna play
In this code, we have two string variables: string_1
with the value 'players'
and string_2
with the value 'gonna play'
. The puts
statement is then employed to output the result of concatenating these two strings using the +
operator and an additional space(' '
).
The output will be players gonna play
, as the two strings are effectively combined with a space in between. The use of the +
operator for string concatenation is less favored in Ruby for its visual impact on code aesthetics.
Use the concat
Method to Concatenate Strings in Ruby
The concat
method in Ruby is used to concatenate strings. This method appends the content of one string to another, effectively combining them into a single string.
When concat
is applied to a string, it modifies the original string in place by adding the characters of another string to the end. This provides an alternative way to concatenate strings in Ruby, particularly when you want to modify the existing string rather than create a new one.
Note: Since the
concat
method concatenates the argument directly to the original string, we must be careful when using it.
Example Code:
apple = 'apple'
apple.concat('pen')
puts apple
Output:
applepen
In the code above, we initialize a string variable apple
with the value 'apple'
.
Next, we use the concat
method to append the string 'pen'
to the original value of apple
. The puts
statement displayed the modified string, resulting in the output applepen
.
The concat
method modifies the original string in place, and when executed, the console will show the concatenated string applepen
.
We can chain the concat
method multiple times.
Example Code:
puts 'HTML'.concat(' is').concat("n't a programming language")
Output:
HTML isn't a programming language
In the code above, the initial string 'HTML'
is modified by sequentially appending the strings ' is'
and "n't a programming language"
. When executed, the output will be HTML isn't a programming language
, reflecting the successful concatenation of the original string with the additional content specified by the concat
method.
We can also use the concat
method to take multiple arguments.
Example Code:
str1 = 'Hello, '
str2 = 'Beautiful '
str3 = 'World!'
str1.concat(str2, str3)
puts str1
Output:
Hello, Beautiful World!
In this code, we work with three string variables: str1
initialized with "Hello, "
, str2
with "Beautiful "
, and str3
with "World!"
. Using the concat
method on str1
, we concatenate the contents of str2
and str3
to it.
The puts
statement is then employed to display the modified str1
in the console. Upon execution, the output will be Hello, Beautiful World!
.
The concat
method allows us to efficiently join multiple strings, and the puts
function ensures the resulting string is presented in the console.
Use the Array.join
Method to Concatenate Strings in Ruby
The Array.join
method is an unusual way to concatenate strings. It’s useful when we want to add a separator between strings.
Basic Syntax:
array_of_strings.join(separator)
In the syntax, array_of_strings
is an array containing the strings you want to concatenate. The separator
string is used to separate each element in the resulting string, and the join
is the method used on the array, and it takes the separator
as an argument.
Example Code:
puts %w[pine apple pen].join(' + ')
Output:
pine + apple + pen
In this code, we utilize the Array.join
method to concatenate strings within an array with the specified separator ' + '
. The array %w[pine apple pen]
contains three strings.
The join
method combines these strings into a single string, inserting the specified separator between each element. When executed, the output will be pine + apple + pen
.
This code illustrates the utility of the Array.join
method for string concatenation within an array.
Use the <<
Operator to Concatenate Strings in Ruby
The <<
operator in Ruby is another way to concatenate strings by appending the content of one string to another.
When the <<
operator is applied to a string, it modifies the original string by adding characters from another string to the end. This operation is performed in place, and the resulting string is the modified version of the original string.
The <<
operator is often employed when you want to concatenate strings and modify the existing string object without creating a new one. It is a concise and direct way to append characters to a string.
Example Code:
str1 = 'Hello, '
str2 = 'World!'
str1 << str2
puts str1
Output:
Hello, World!
In this code, we work with two string variables: str1
initialized with "Hello, "
and str2
with "World!"
. The <<
operator is then used on str1
to efficiently concatenate the contents of str2
to it.
Upon execution, the output will be Hello, World!
. The <<
operator serves as a concise way to append one string to another.
Use the *
Operator to Concatenate Strings in Ruby
We can use the *
operator to concatenate a string with itself multiple times.
The *
operator is used to concatenate strings by repeating the content of a string a specified number of times. When the *
operator is applied to a string and an integer, it creates a new string by repeating the original string’s content the specified number of times.
This operator is particularly useful when you need to concatenate a string with itself multiple times or create a repeated pattern of characters. It provides a concise way to generate longer strings by replicating the content of a given string.
Example Code:
str = 'Hello '
result = str * 3
puts result
Output:
Hello Hello Hello
In this code, we initialize a string variable str
with the value "Hello "
. Next, we use the *
operator to concatenate the string with itself three times, creating a new string, and the result is assigned to the variable result
.
When executed, the output will be Hello Hello Hello
, showcasing the successful repetition and concatenation of the original string. The *
operator provides a concise way to achieve string duplication.
Conclusion
Ruby provides a variety of methods for concatenating strings, each with its unique advantages and use cases. String interpolation emerges as a favored choice for its elegance, while methods like concat
, Array.join
, <<
, and *
operators cater to different scenarios, allowing developers to choose the suitable approach based on their specific requirements and coding preferences.
Understanding these methods equips Ruby developers with the flexibility to enhance code readability and efficiency in string concatenation tasks.