Difference Between OR and Double Pipe Operator in Ruby
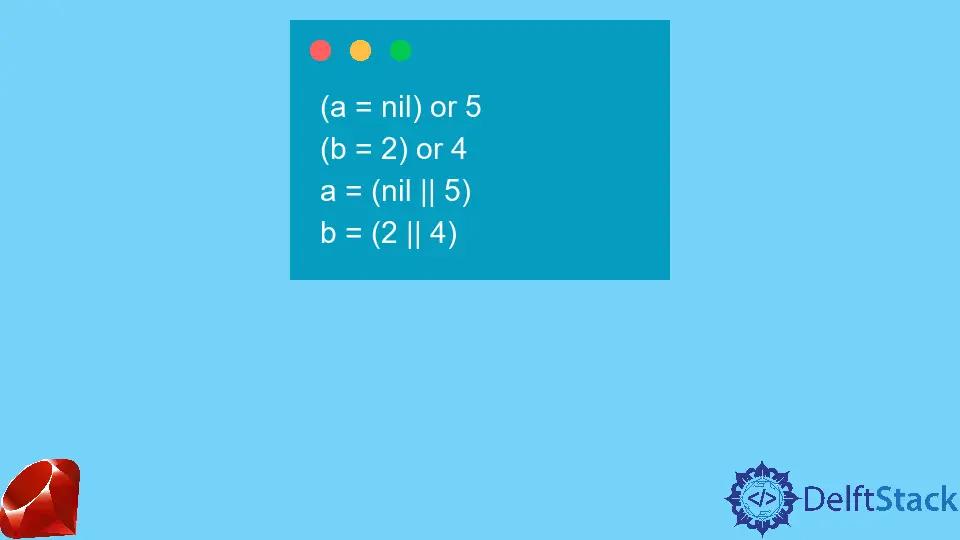
The or
and ||
are both logical operators in Ruby; they can be confusing due to their similar behavior. There is only a slight difference between them, and
In this tutorial, we will be looking at how they are different and how each should be used.
One important thing to remember is that they behave the same way if they appear in an expression that contains no other operator. Otherwise, they behave differently.
When No Other Operator Is Involved
In this classification, the two operators behave the same way, returning the first truthy value.
Example Code:
nil or 5
2 or 4
Output:
5
2
Example Code:
nil || 5
2 || 4
Output:
5
2
Note that in the examples above, the codes are in the form of expression
and not statement
. Combining anything that will make it a statement would produce an unexpected result.
For example, nil or 5
is an expression that can be passed around, maybe as an argument to a method or assigned to a variable, whereas puts nil or 5
is a complete statement on its own.
The best way to test Ruby expressions is by using the interactive Ruby
IRB. It comes pre-installed on macOS. To test with IRB, follow the steps below.
-
Open a terminal
-
Type
irb
and press Enter. This will show a prompt -
Type your expression, for example,
nil || 5
, and press Enter -
The result of the expression will show up on the next line
When Some Other Operators Are Involved
Our focus is on the value of a
after evaluating the code in the examples below.
Example Code:
a = nil or 5
b = 2 or 4
puts a
puts b
Output:
nil
2
Example Code:
a = nil || 5
b = 2 || 4
puts a
puts b
Output:
5
2
The best way to explain the above behavior is by looking at the Ruby operators precedence table. Whenever an expression is made up of multiple operators, as we have in the examples above, we need to evaluate them based on their precedence.
The table shows that the =
operator has higher precedence than the or
operator but is lower than the ||
operator. Therefore the order of evaluation in the examples can be broken down as shown below.
(a = nil) or 5
(b = 2) or 4
a = (nil || 5)
b = (2 || 4)
Although we decided to use the =
operator in the above examples, we can use any operator higher than or
but lower than ||
and get the same behavior; an example is +=
.
Example Code:
a = 1
a += 2 || 4 # a += (2 || 4)
puts a
Output:
3
Example Code:
a = 1
a += 2 or 4 # (a += 2) or 4
puts a
Output:
3
Example Code:
a = 1
a += nil || 5 # a += (nil || 5)
puts a
Output:
6
Example Code:
a = 1
a += nil or 5 # (a += nil) or 5
It will throw a TypeError (nil can't be coerced into Integer)
because Ruby tries to evaluate the expression in the bracket first, incrementing a
by nil
.