Not Equal Operator in Ruby
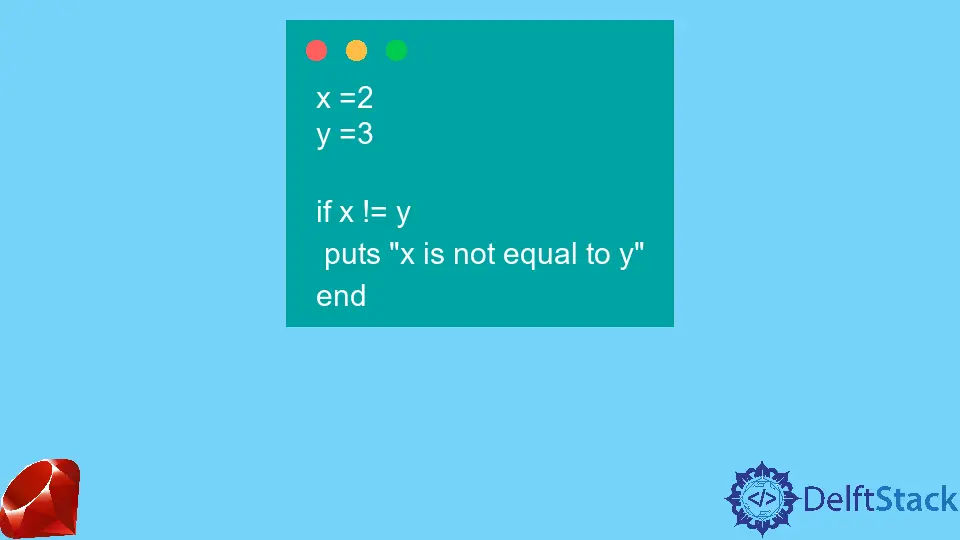
Ruby has several comparison operators. The most commonly used one is the equality operator ==
, which checks if the data types and values are equal.
But what if we wanted to examine if two values are not equal? To do that, we use the !=
operator.
Not Equal Operator in Ruby
The !=
operator can be used with any data type, including numbers, strings, and Booleans. We can use it with data types that can be compared, such as numbers and strings.
This operator is often used in conditionals, such as if
and unless
statements. Using the !=
operator is suitable when checking if two deals are unequal.
Example Code:
puts 'Hello user!' if $name != 'John'
Output:
Hello user!
If the references point to different objects, the objects are not equal, and !=
will evaluate as true. If they point to the same object, the objects are equal and !=
will evaluate as false.
!=
is a comparison operator in Ruby which can also check whether two values or variables are equal. If they are not equal, it returns true
; else, it returns false
.
For example, the following code will print false
to the console because the two values are not equal:
puts 1 != 2
The !=
operator is not part of Ruby’s core syntax. It is a part of the standard library and must be included before use.
The !=
operator is identical to the <=>
operator (spaceship operator). It compares two values for inequality in the same way that the ==
operator is used to compare two values for equality.
Use the Not Equal Operator in Ruby
As mentioned above, this operator is often used in conditional statements to check whether a given value is not identical to another value. For instance, the following code checks whether the value of the variable x
is not similar to the value of the variable y
:
Example Code:
x = 2
y = 3
puts 'x is not equal to y' if x != y
Output:
x is not equal to y
As you can see in this example, x
is not equal to y
, which returns true
, and the body of if
is executed.
Example Code:
i = 10
puts 'Hi, I am a good boy' unless i < 3
Output:
Hi, I am a good boy
Let’s see a few other scenarios.
puts 1 != 2 # => true
puts 1 != 1 # => false
puts 'a' != 'b' # => true
puts 'a' != 'a' # => false
Conclusion
The article concludes that the not equal operator in Ruby is used to check whether two inputs are not equal. It produces true if the objects are not equal and false if they are equal.
The not equal operator in Ruby is a useful tool that can help make your code more concise and easier to read. It can be utilized to review if two values are not equal, and if they are not, they will return true.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedIn