How to Create Editable Table in React
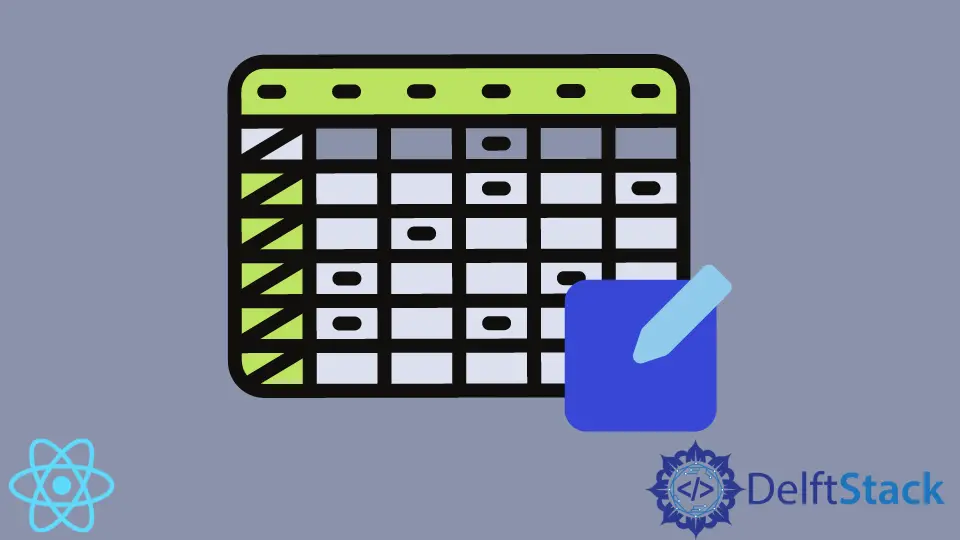
The table is the most important part for any application to visualize data. In React, there is a table named Editable
table through which you can edit dynamically, mainly used to enable editing data by users.
In this short article, we’ll see how we can create an editable table in React with explanations.
Create an Editable Table in React
Our example below will illustrate how we can create an editable table using React. Follow the below example codes.
Code - App.js
:
import React from 'react';
export default class DynamicTable extends React.Component {
// Class constructor
constructor(props) {
super(props);
this.state = { Name: '', Data: [] }
}
// An action event on updated data
UpdateData(event) {
this.setState({Name: event.target.value});
}
// A click action
ClickAction() {
var Data = this.state.Data;
Data.push(this.state.Name);
this.setState({Data: Data, Name: ''});
}
// An action event on data change
OnDataChange(i, event) {
var Data = this.state.Data;
Data[i] = event.target.value;
this.setState({Data: Data});
}
// An action event on data delete
OnDataDelete(i) {
var Data = this.state.Data;
Data.splice(i, 1);
this.setState({Data: Data});
}
// Organizing the table
DrawTable() {
var context = this;
return this.state.Data.map(function(o, i) {
return (
<tr key={'item-' + i}>
<td>
<input type='text' value={o} onChange={
context.OnDataChange.bind(context, i)}/>
</td>
<td>
<button onClick={context.OnDataDelete.bind(context, i)}>Delete</button>
</td>
</tr>
);
});
}
// Rendering the UI
render() {
return (
<div>
<table>
<thead>
<tr>
<th>Name</th>
<th>Actions</th>
</tr>
</thead>
<tbody>{this.DrawTable()}</tbody>
</table>
<hr/>
<input type='text' value={this.state.Name} onChange={this.UpdateData.bind(this)}/>
<button onClick={this.ClickAction.bind(this)}>Add Item </button>
</div>
);
}
}
We already commanded the purpose of each code block. Now let’s look at the index.js
file, which looks like the one below.
Code - index.js
:
// importing necessary packages and files
import './index.css';
import React from 'react';
import ReactDOM from 'react-dom/client';
import reportWebVitals from './reportWebVitals';
import DynamicTable from './table.js';
// Specifying the parent element
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(
<React.StrictMode>
<DynamicTable />
</React.StrictMode>
);
reportWebVitals();
After you finish all the files, you will get the output below in your browser.
Output:
The example codes shared in this article are written in React JS project. Your system must contain the latest Node JS version to run a React project.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn